We have seen how to create the Java LinkedList. In this post we will learn how does LinkedList insertion work? In Java LinkedList, each element is a node that linked with other nodes. Let’s see how to insert a node in linked list. Java LinkedList provides some methods that can insert element in linked list.
Here is the table content of the article will we will cover this topic.
1. add(E e) method
2. add(int index, E e) method
3. addAll(Collection c) method
4. addAll(int index, Collection c)
5. addFirst(E e) method
6. addLast(E e) method
7. offer(E e) method
8. offerFirst(E e) method
9. offerLast(E e) method
10. push(E e) method
add(E e) method
The add(E e) method is used to add the given element in LinkedList. It adds the element at the end of the LinkedList. It internally invokes the lastLink() method. This method is useful when we want to insert node at end of LinkedList java.
boolean add(E e);
Where E represents the type of elements in LinkedList.
e is the element that you want to add in this LinkedList.
return type: Its return type is boolean.
Let’s take an example LinkedList insertion, how does add method work. Suppose we have a LinkedList having some element and now we want to add one more element.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22,33)); System.out.println("Elements: "+ firstList); firstList.add(12); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22, 33]
Elements: [11, 22, 33, 12]

2. add(int index, E e) method
The add(int index, E e) method is used to add the specified element at the specified index in LinkedList. This method doesn’t return any value because its return type is void. It is used to insert a node at a specific position in a linked list.
boolean add(int index, E e);
Where index, index at which the specified element is to be inserted.
E, represents the type of elements in the LinkedList.
e, is the element which you want to add in this LinkedList.
return type: Its return type is void. throw, IndexOutOfBoundsException if index is invalid.
Suppose we have a LinkedList having some element and now we want to add insert element in linked list at specific position. Let’s see example of LinkedList insertion
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22,33)); System.out.println("Elements: "+ firstList); firstList.add(1, 12); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22, 33]
Elements: [11, 12, 22, 33]
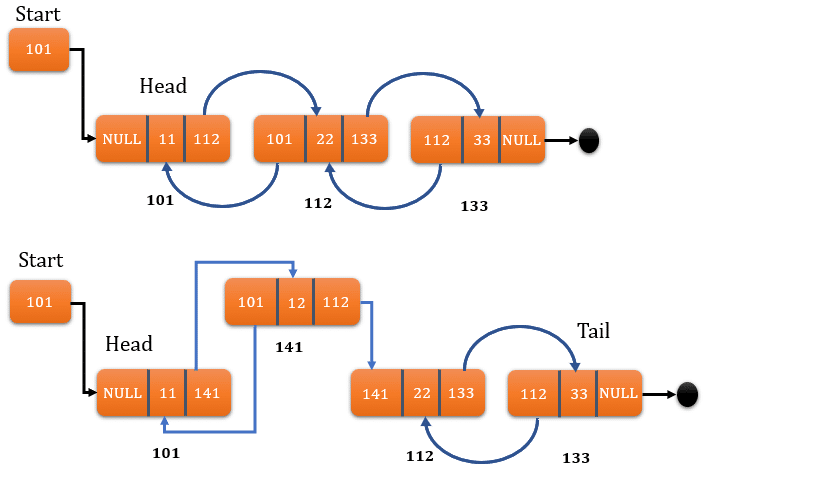
3. addAll(Collection c) method
The addAll(Collection c) method is used to add all the elements at the end of the LinkedList. This method appends the specified collection to the end of ArrayList. Its return type is boolean. It invokes internally the addAll(int index, collection c).
boolean addAll(Collection c);
Where, c collection containing elements to be added to LinkedList.
throws NullPointerException, if the specified collection is null.
return type, its return type is boolean.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22)); System.out.println("Elements: "+ firstList); LinkedList<Integer> secondList = new LinkedList<Integer>(Arrays.asList(33,13)); firstList.addAll(secondList); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22]
Elements: [11, 22, 33, 13]

4. addAll(int index, Collection c) method
The addAll(int index, Collection c) method is used to add all the elements of the specified collection at a specific position in LinkedList. Its return type is boolean. We can use this method when we want to insert multiple node at a specific position in a linked list.
boolean addAll(int index, Collection c);
Where index, index at which the specified element is to be inserted.
c, a collection containing elements to be added to LinkedList.
throws NullPointerException, if the specified collection is null.
return type, it’s return type is boolean.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22)); System.out.println("Elements: "+ firstList); LinkedList<Integer> secondList = new LinkedList<Integer>(Arrays.asList(33,13)); firstList.addAll(1, secondList); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22]
Elements: [11, 33, 13, 22]

5. addFirst(E e) method
The addFirst(E e) method is used to add the given element at the beginning of the LinkedList. Its return type is void. It internally invokes the linkFirst() method.
void addFirst(E e);
Where E represents the type of elements in LinkedList.
e is the element that you want to add on this LinkedList.
return type: Its return type is void. It means it doesn’t return anything.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22,33)); System.out.println("Elements: "+ firstList); firstList.addFirst(44); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22, 33]
Elements: [44, 11, 22, 33]

6. addLast(E e) method
The addLast(E e) method is used to add the given element at the end of the LinkedList. It works like add() method because it is also invoking the linkLast(E e) method. Its return type is void.
void addLast(E e);
Where E represents the type of elements in LinkedList.
e is the element that you want to add in this LinkedList.
return type: Its return type is void. It means it doesn’t return anything.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22,33)); System.out.println("Elements: "+ firstList); firstList.addLast(44); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22, 33]
Elements: [11, 22, 33, 44]
7. offer(E e) method
The offer(E e) method is used to add the given element at the end of LinkedList. Its return type is boolean. This method is declared in the Queue interface. This method internally invokes the add() method.
boolean offer(E e);
Where, E represents the type of elements in Linkedlist.
e is the element which you want to add in this Linkedlist.
return type: Its return type is boolean. It returns true if element added successfully otherwise false.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22,33)); System.out.println("Elements: "+ firstList); firstList.offer(44); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22, 33]
Elements: [11, 22, 33, 44]
8. offerFirst(E e) method
The offerFirst(E e) method is used to add the given element at the beginning of the LinkedList. Its return type is boolean. This method is declared in Deque interface.
.boolean offerFirst(E e);
Where, E represents the type of elements in Linkedlist.
e is the element which you want to add in this Linkedlist.
return type: Its return type is boolean. It returns true if element added successfully otherwise false.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22,33)); System.out.println("Elements: "+ firstList); firstList.offerFirst(44); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22, 33]
Elements: [44, 11, 22, 33]
9. offerLast(E e) method
The offerLast(E e) method is used to add the given element at the end of the LinkedList. Its return type is boolean.
boolean offerLast(E e);
Where E represents the type of elements in LinkedList.
e is the element which you want to add in this LinkedList.
return type: Its return type is boolean. It returns true if element added successfully otherwise false.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22,33)); System.out.println("Elements: "+ firstList); firstList.offerFirst(01); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22, 33]
Elements: [1, 11, 22, 33]
10. push(E e) method
The push(E e) method is used to add the given element at the beginning of the LinkedList. Its return type is void. This method is declared in the Deque interface. This method internally invokes the addFirst() method and perform LinkedList insertion operation.
void push(E e);
Where, E represents the type of elements in Linkedlist.
e is the element which you want to add in this Linkedlist.
return type: Its return type is void. It means it doesn’t return anything.
import java.util.Arrays; import java.util.LinkedList; public class ExampleOfLinkedList { public static void main(String[] args) { // Creating a empty LinkedList LinkedList<Integer> firstList = new LinkedList<Integer>(Arrays.asList(11,22,33)); System.out.println("Elements: "+ firstList); firstList.push(12); System.out.println("Elements: "+ firstList); } }
Output: Elements: [11, 22, 33]
Elements: [12, 11, 22, 33]