In this chapter, we will discuss all the operators in Java. The operator is a symbol that is used to perform operations. It represents an action. For example: +, -, *, / etc. There are different types of Operators in Java.
Youtube video available in the Hindi language
Types of Operators in Java
1. Arithmetic Operators
2. Unary Operators
3. Assignment Operator
4. Relational Operators
5. Logical Operators
6. Ternary Operator
7. Bitwise Operators
8. instance of operator
9. Precedence and Associativity
1. Arithmetic Operators in java
Arithmetic operators are used to perform arithmetic operations on primitive data types. JAVA has some arithmetic operators:
Operators | Symbol |
Multiple | * |
Divide | / |
Modulo | % |
Addition | + |
Subtraction | – |
public class ArithmeticOperators { public static void main(String args[]) { // Multiple * // Divide / // Modulo % // + // - int a = 10, b = 5; String x = "JAVA", y = "Goal.com"; // Example of * operator System.out.println("a * b = " + (a * b)); // Example of / operator System.out.println("a / b = " + (a / b)); // Example of % operator. // It gives remainder on dividing first operand with second System.out.println("a % b = " + (a % b)); // Example of + operator with numeric data System.out.println("a + b = " + (a + b)); // Example of + operator with String data and concatenate the string System.out.println("x + y = " + (x + y)); // Example of - operator System.out.println("a - b = " + (a - b)); // Example of Precedence and Associativity int expressionOne = a * b / 2 % 2 + b - a; System.out.println("Value : "+ expressionOne); int expressionTwo = 10 - 5 + 2 + 10 * 5 / 2 + 5; System.out.println("Value : "+ expressionOne); } }
Output:
a * b = 50
a / b = 2
a % b = 0
a + b = 15
x + y = JAVAGoal.com
a – b = 5
Value : -4
Value : -4
2. Unary Operators in java
Unary operators required only one operator. Unary operators are used to performing various operations:
- Unary minus (-): It used to convert into a negative number
- Unary plus (+): It used to convert into a positive number
- Increment operator (++): It increments the value by 1. There are two variations of increment operators.
- Post-Increment: First use the variable to increment the value of a variable.
- Pre-Increment: It is the opposite of post-increment. In this first increment, the value and after that used the value of a variable.
- Decrement operator (–): It decrements the value by 1. There are two variations of the decrement operator.
- Post-Decrement: First use the variable after that decrement of the value of a variable.
- Pre- Decrement: It is the opposite of post-decrement. In these first decrements, the value and after that used the value of a variable.
- Logical not operator (!): It is used with a boolean value. It inverts the value of the boolean.
Example of Increment and decrement operators:
public class UnaryOperators { public static void main(String[] args) { // Increment operator ++ , +1 // Decrement operator -- , -1 int x = 0; x++; x = x + 1; x--; x =x-1; int a = 5; // It will print 5 because in post-increment // It will assign the value first and then increment System.out.println("After post increment print value: "+ a++); // After increment value of a is 6. System.out.println("After post increment backend value: 6"); // It will print 7 because in pre-increment // It will increment first and then assign the value first System.out.println("After pre increment print value: "+ ++a); // After increment value of a is 7. System.out.println("After post increment backend value: 7"); // It will print 7 because in post-decrement // It will assign the value first and then decrement System.out.println("After post decrement print value: "+ a--); // After increment value of a is 6. System.out.println("After post decrement backend value: 6"); // It will print 5 because in pre-decrement // It will increment first and then assign the value first System.out.println("After pre decrement print value: "+ --a); // After increment value of a is 5. System.out.println("After post increment backend value: 5"); System.out.println("Value of a: "+ --a); System.out.println("Value of a: "+ a++); System.out.println("Value of a: "+ ++a); } }
Output:
After post increment print value: 5
After post increment backend value: 6
After pre increment print value: 7
After post increment backend value: 7
After post decrement print value: 7
After post decrement backend value: 6
After pre decrement print value: 5
After post increment backend value: 5
Value of a: 4
Value of a: 4
Value of a: 6
Example of Logical not operators:
class UnaryOperatorExample { public static void main(String args[]) { boolean a = false; System.out.println("Value of a by use of logical and operator: "+ !a); } }
Output: Value of a by use of logical and operator: true
3. Assignment Operator
- The assignment operator is used to assign value to a variable.
- The value given on the right-hand side of the operator is assigned to the variable on the left.
- The right side value must be constant or declared before used.
variable_Name = value;
Shorthand operator: When an assignment operator is combined with another operator. Like +=, *= etc.
If the assignment operator combined with other operators to build a shorter version of a statement called Compound Statement.
Shorthand operator | Description |
*= | Multiplying left operand with right operand and then assigning it to the variable on the left. |
/= | Dividing left operand with right operand and then assigning it to the variable on the left. |
%= | Assigning modulo of left operand with right operand and then assigning it to the variable on the left. |
+= | Adding left operand with right operand and then assigning it to the variable on the left. |
-= | Subtracting left operand with right operand and then assigning it to the variable on the left. |
Example of Shorthand operators:
public class AssignmentOperator { public static void main(String[] args) { // Assignment operator = // shorthand operator // Right side value must be constant or declared before used int number1 = 8; int number2 = 5; String webSite = "JavaGoal.com"; int sum = 8 + 2; // Shorthand operator sum = sum + 5; System.out.println("Value: " + sum); sum += 5; System.out.println("Value: " + sum); sum = sum - 5; System.out.println("Value: " + sum); sum -= 5; System.out.println("Value: " + sum); sum = sum * 5; System.out.println("Value: " + sum); sum *= 5; System.out.println("Value: " + sum); int a = 1; a++; System.out.println("Value: " + a); a += 1; System.out.println("Value: " + a); } }
Output: Value: 15
Value: 20
Value: 15
Value: 10
Value: 50
Value: 250
Value: 2
Value: 3
4. Relational operator
These operators are used to check the relations between two values. It returns the boolean result after the comparison. The relational operator is mostly used in looping and conditional statements. Some Relational operators:
- Equals to (= =): It returns true if the left-hand side and right-hand side are equal otherwise false.
- Not equals (! =): It returns true if the left-hand side does not equal to right-hand side otherwise true.
- Less than (<): It returns true if the left-hand side is less than from the right-hand side otherwise false.
- Less than equals to (<=): It returns true if the left-hand side is less than or equals to the right-hand side otherwise false.
- Greater than (>): It returns true of the left-hand side is greater than the right-hand side.
- Greater than equals to(>=): It returns true of the left-hand side is greater than or equals to the right-hand side.
Example of Relational Operators:
public class RelationalOperator { public static void main(String[] args) { // Equal to == // Not equal to != // Less than < // Less than or equal to <= // Greater than > // Greater than or equal to >= int a = 1, b = 1, c = 3, d = 4; System.out.println("Is a equal to b: " + (a == b)); System.out.println("Is a equal to c: " + (a == c)); System.out.println("Is a not equal to b: " + (a != b)); System.out.println("Is a not equal to c: " + (a != c)); System.out.println("Is a less than b: " + (a < b)); System.out.println("Is a less than c: " + (a < c)); System.out.println("Is a less or equal than b: " + (a <= b)); System.out.println("Is a less or equal than c: " + (a <= c)); System.out.println("Is a greater than b: " + (a > b)); System.out.println("Is a greater than c: " + (c > a)); System.out.println("Is a greater or equal than b: " + (a >= b)); System.out.println("Is a greater or equal than c: " + (a >= c)); } }
Output: Is a equal to b: true
Is a equal to c: false
Is a not equal to b: false
Is a not equal to c: true
Is a less than b: false
Is a less than c: true
Is a less or equal than b: true
Is a less or equal than c: true
Is a greater than b: false
Is a greater than c: true
Is a greater or equal than b: true
Is a greater or equal than c: false
5. Logical Operators
These operators are used to perform “logical AND” and “logical OR” operation. Working logical operators are the same as Logical gates in digital. Logical operators three types are:
- Logical AND (&&): If will return true if both conditions are true
- Logical OR (||): It will return true if at least one condition is true
- Logical NOT(!): It is used to reverse the state of the variable
Example of Logical Operators:
public class LogicalOperators { public static void main(String[] args) { // AND && // OR | // NOT ! int a = 0; int b = 1; boolean c = false; // In this case both condition should true. // It will print the statement if both will be true if((a < 1) && (b < 2)) { System.out.println("AND operation is executed"); } // In this case first condition(a < 0) is false. // So it will not any print the statement if((a < 0) && (b < 2)) { System.out.println("AND operation is executed"); } // In this case only one condition should be true. // So it will print the statement if((a < 1) || (b < 0)) { System.out.println("OR operation is executed"); } // In this case first condition is false and second is true. // But OR operator need at least one condition true. // So it will print the statement if((a < 0) || (b < 2) || (b < 3)) { System.out.println("OR operation is executed"); } if((a < 1) || (b < 0)) { System.out.println("OR operation is executed"); } // NOT perform reverse operation. // So it will return value of c is true if(!c) { System.out.println("NOT operation is executed"); } } }
Output: AND operation is executed
OR operation is executed
OR operation is executed
OR operation is executed
NOT operation is executed
6. Ternary operator
The ternary operator is an alternate version of the if-else statement. It is based on condition. It has three operands.
Condition ? first Statement : second Statement;
If the condition is true, the first statement will execute otherwise the second statement will execute.
public class TernaryOperatorExample { public static void main(String args[]) { // Ternary operator ? : int a = 5; int b = 2; int result = 0; // Here we try to find bigger number. //If "a" is greater than "b" then "a" will assign to result otherwise "b"; result = (a > b) ? a : b; System.out.println("The bigger number is : "+ result); result = (b > a) ? a : b; System.out.println("The smallest number is : "+ result); } }
Output: The bigger number is : 5
The smallest number is : 2
7. Bitwise Operators
JAVA has some Bitwise operator that can be applied on any integer types, long, int, short, char, and byte. Bitwise operators work on bits of a number.
- Bitwise AND operator (&): It will convert the operands to the binary digit and copies a bit to the result if it exists in both operands.
- Bitwise OR operator ( | ): It will convert the operands to the binary digit and copies a bit if it exists in either operand.
- Bitwise XOR operator (^): It will convert the operands to the binary digit and copies the bit if it is set in one operand but not both.
- Bitwise Complement Operator (~): This is a unary operator that returns the one’s complement representation of the input value, i.e. with all bits inverted.
How Bitwise Operator is working?
Let’s take two operands
int a = 5;
int b = 3
Bitwise operator works on bits. So firstly, the compiler converts both the operand in binary form.
a = 101
b = 011
Bitwise AND operator (&): It will copy the bit which is 1 in both operands.
1 0 1
0 1 1
Result of a&b = 0 0 1
Bitwise OR operator ( | ): It will copy the bit 1 either in the first operand or in second operands.
1 0 1
0 1 1
Result of a|b = 1 1 1
Bitwise XOR operator ( ^ ): It will copy the bit 1 if it is set in one operand but not both.
1 0 1
0 1 1
Result of a^b = 1 1 0
Bitwise complement operator ( ~ ): It returns the one’s complement representation of the input value
1 0 1
Result of ~a = 0 1 0
8. instance of an operator
It is used for type check the object. It can be used to test an object is an instance of a class or an interface. It can apply only non-primitive data types.
Object instanceOf className/InterfaceName;
import java.util.ArrayList; public class InstanceOfOperator { // instanceOf public static void main(String[] args) { InstanceOfOperator object = new InstanceOfOperator(); System.out.println(object instanceof InstanceOfOperator); String name = "Hello"; System.out.println(name instanceof String); // System.out.println(name instanceof InstanceOfOperator); ArrayList<Integer> list = new ArrayList<Integer>(); System.out.println(list instanceof ArrayList); } }
Output: true
true
true
9. Precedence and Associativity of Operators in Java
As we already discuss all the Operator in Java. So now we are starting with the Precedence and associative are rules. There are certain rules followed by all Operators in Java. That would be used when we are solving any equations which have more than one type of operator. In such type of cases, these rules determine which part of the equation will be evaluated first. Here we have a table that represents the precedence of operators from high to low.
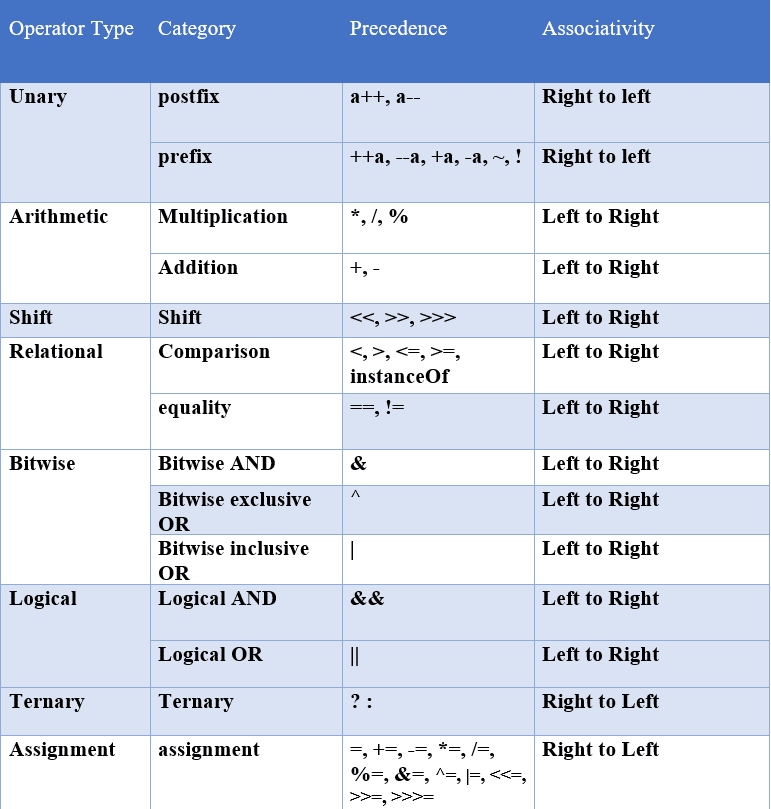
1. Quiz, Read the below code and do answer.
Click on anyone to know the answer.