An ArrayList is a growable array and each element stored in a particular index. We can get the subList from an ArrayList. In this post, we will see java list sublist.
The subList() method is used to get a sublist from ArrayList. It returns a portion of ArrayList between the specified fromIndex and toIndex (If fromIndex and toIndex are equal, the returned list is empty.)
public List<E> subList(int fromIndex, int toIndex)
Where fromIndex, It is the index of the first element of the sublist.
toIndex, It is the index of the last element of the sublist.
NOTE: fromIndex, always include to get the subList but toIndex always excludes.
Exception, It can throw the following Exception.
IndexOutOfBoundsException – if a fromIndex or toIndex value is out of range
IllegalArgumentException – if fromIndex or toIndex are out of order (fromIndex > toIndex)
Let’s see java sublist example and also discuss memory representation:
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<Integer> listOfNumber = new ArrayList<Integer>(); listOfNumber.add(11); listOfNumber.add(22); listOfNumber.add(33); listOfNumber.add(44); listOfNumber.add(55); System.out.println("SubList: "+ listOfNumber.subList(0, 2)); System.out.println("SubList: "+ listOfNumber.subList(3, 5)); } }
Output: SubList: [11, 22]
SubList: [44, 55]
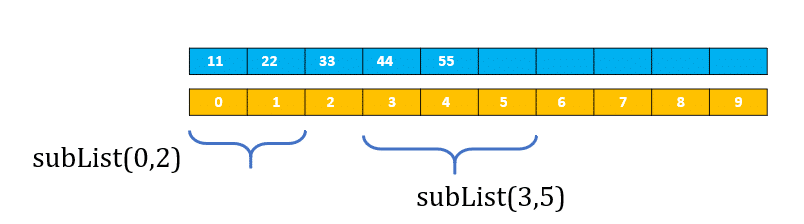
As you can see in about example, we are getting two subList from an ArrayList. The fromIndex includes in subList and toIndex excludes from subList.
The subList() method returns a portion from ArrayList so we need to store it in another ArrayList. We can’t use it directly we need to typecast the returned subList. Let’s see the java list sublist
import java.util.ArrayList; import java.util.List; public class ArrayListExample { public static void main(String[] args) { ArrayList<Integer> listOfNumber = new ArrayList<Integer>(); listOfNumber.add(11); listOfNumber.add(22); listOfNumber.add(33); listOfNumber.add(44); listOfNumber.add(55); List<Integer> subList1 = listOfNumber.subList(0, 2); System.out.println("SubList1: "+ subList1); ArrayList<Integer> subList2 = new ArrayList<Integer>(listOfNumber.subList(0, 4)); System.out.println("SubList2: "+ subList2); } }
Output: SubList1: [11, 22]
SubList2: [11, 22, 33, 44]