In java, Set interface is one of the most important interfaces implemented by HashSet, LinkedHashSet, and TreeSet. If you haven’t read HashSet and LinkedHashSet yet, then you should read them first. In this post, we will read what is TreeSet in java and Java TreeSet example.
You can read it with an example from here.
1. What is TreeSet in Java?
2. Important points TreeSet in java?
3. Creation of TreeSet in java?
4. Constructors of TreeSet class?
5. Java TreeSet example?
6. TreeSet internal working?
7. How TreeSet sorts the elements?
8. Why TreeSet doesn’t accept null?
9. TreeSet Methods in Java?
10. Insert Elements to TreeSet?
11. Remove element from TreeSet?
12. Get the element from TreeSet?
13. How to get SubSet from TreeSet?
1. What is TreeSet in Java?
The TreeSet class in java is a part of the Java Collection Framework that implements the NavigableSet interface(It extends SortedSet) and extends the AbstractSet class. It also implements the Cloneable and Serializable interface.
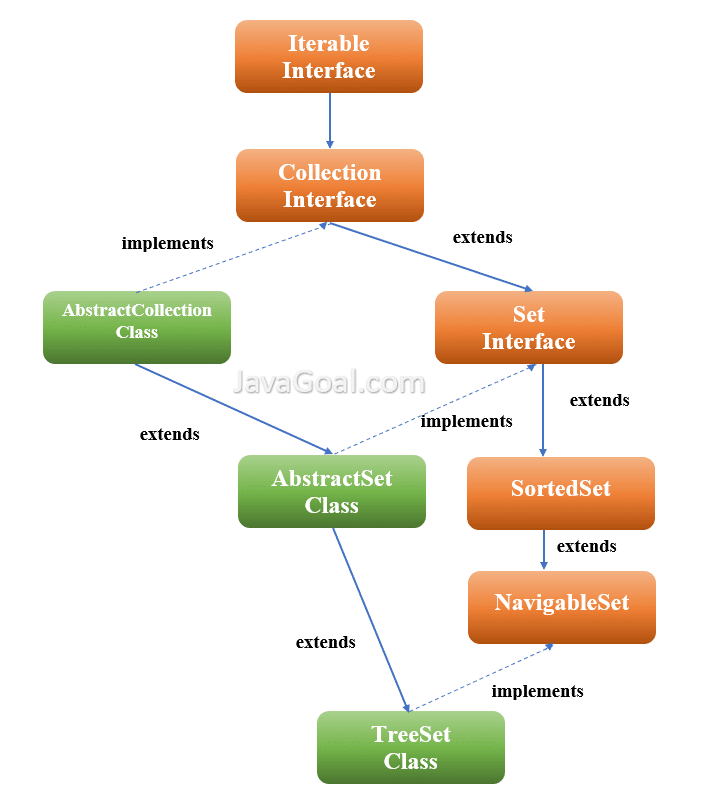
Like HashSet and LinkedHashSet, TreeSet in java also contains only unique elements. A TreeSet maintains the natural order of element but HashSet doesn’t maintain any order. The order of the elements in natural order whether or not an explicit comparator is provided. TreeSet is the most important part of the collection to store the Tree because it implements the SortedSet interface.
2. Important points TreeSet in java
1. The Tree class implements the SortedSet interface and NavigableSet interface. The NavigableSet interface allows to navigate on Set and maintain the order.
2. Like HashSet and LinkedHashSet, duplicate values are not allowed in TreeSet.
3. Unlike HashSet and LinkedHashSet, A TreeSet doesn’t allow NULL value. If we try to insert any NULL value, it throws a NullPointerException.
4. The TreeSet maintains the natural order of elements by default. We can provide an explicit comparator to sort the elements.
5. The performance of TreeSet is similar to HashSet, it provides constant-time performance for the basic operations.
6. TreeSet is not synchronized by default. If we want to use it in a multithreading environment, it must be synchronized externally.
7. TreeSet internally uses the TreeMap to store the elements.
3. Creation of TreeSet in java
Like a HashSet class, TreeSet class has various constructors that are used to create a TreeSet. A TreeSet class provides four constructors, each takes a different type of parameter.
4. Constructors of TreeSet class
1. TreeSet()
2. TreeSet(Collection c)
3. TreeSet(Comparator comparator)
4. TreeSet(SortedSet<E> s)
1. TreeSet()
It is the default constructor of TreeSet class that creates an empty TreeSet. It sorts all the elements in the natural order. It internally uses the TreeMap and performs all operations on TreeMap. The TreeSet compares each element before insertion by use of compareTo() method.
public TreeSet() { this(new TreeMap<>()); }
Let’s take an example and see how to create a TreeSet by default constructor.
import java.util.TreeSet; public class TreeSetExample { public static void main(String arg[]) { TreeSet<String> stringTreeSet = new TreeSet<String>(); stringTreeSet.add("BBB"); stringTreeSet.add("ZZZ"); stringTreeSet.add("AAA"); stringTreeSet.add("CCC"); stringTreeSet.add("YYY"); TreeSet<Integer> integerTreeSet = new TreeSet<Integer>(); integerTreeSet.add(10); integerTreeSet.add(2); integerTreeSet.add(1); integerTreeSet.add(5); integerTreeSet.add(8); System.out.println("Element from String TreeSet: "+ stringTreeSet); System.out.println("Element from Integer TreeSet: "+ integerTreeSet); } }
Output: Element from String TreeSet: [AAA, BBB, CCC, YYY, ZZZ]
Element from Integer TreeSet: [1, 2, 5, 8, 10]
2. TreeSet(Collection c)
It is a parameterized constructor of TreeSet class that creates a TreeSet and copy all the elements of the given collection. It maintains the natural order of elements. It uses the compareTo() method to sort the elements. It throws NullPointerException if the specified collection is null.
public TreeSet(Collection<? extends E> c) { this(); addAll(c); }
Let’s take an example and see how to create a TreeSet by use of Collection.
import java.util.ArrayList; import java.util.TreeSet; public class TreeSetExample { public static void main(String arg[]) { ArrayList<String> stringArrayList = new ArrayList<String>(); stringArrayList.add("BBB"); stringArrayList.add("ZZZ"); stringArrayList.add("AAA"); stringArrayList.add("CCC"); stringArrayList.add("YYY"); stringArrayList.add("ZZZ"); TreeSet<String> treeSet = new TreeSet<String>(stringArrayList); System.out.println("Element from TreeSet: "+ treeSet); } }
Output: Element from TreeSet: [AAA, BBB, CCC, YYY, ZZZ]
3. TreeSet(Comparator comparator)
It is a parameterized constructor that takes a comparator. It creates a constructor and sorts the elements of TreeSet according to the specified comparator. Before insertion of the element, each element compares with comparator.
public TreeSet(Comparator<? super E> comparator) { this(new TreeMap<>(comparator)); }
Let’s take an example and see how to create a TreeSet by use of custom comparator.
import java.util.Comparator; import java.util.TreeSet; class Student implements Comparator<Student> { int rollNo; String className; String name; public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getClassName() { return className; } public void setClassName(String className) { this.className = className; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Student(int rollNo, String className, String name) { this.rollNo = rollNo; this.className = className; this.name = name; } Student() { } @Override public int compare(Student student1, Student student2) { if(student1.rollNo==student2.rollNo) return 0; else if(student1.rollNo>student2.rollNo) return 1; else return -1; } } public class TreeSetExample { public static void main(String arg[]) { TreeSet<Student> students = new TreeSet<Student>(new Student()); students.add(new Student(5, "MCA", "Ram")); students.add(new Student(3, "MCA", "John")); students.add(new Student(15, "MCA", "Krishna")); students.add(new Student(10, "MCA", "Johny")); students.add(new Student(1, "MCA", "Vindhu")); System.out.println("Elements from TreeSet: "); for(Student student : students) { System.out.println("RollNo: "+student.getRollNo()); System.out.println("Class Name: "+ student.getClassName()); System.out.println("Name: "+ student.getName()); } } }
Output: Elements from TreeSet:
RollNo: 1
Class Name: MCA
Name: Vindhu
RollNo: 3
Class Name: MCA
Name: John
RollNo: 5
Class Name: MCA
Name: Ram
RollNo: 10
Class Name: MCA
Name: Johny
RollNo: 15
Class Name: MCA
Name: Krishna
4. TreeSet(SortedSet<E> s)
This constructor is used to create a TreeSet and contain all the elements from given SortedSet. It maintains the order of elements the same as in specified SortedSet. It throws NullPointerException if given SortedSet is null.
Let’s take an example and see how to create a TreeSet by use of SortedSet.
import java.util.SortedSet; import java.util.TreeSet; public class TreeSetExample { public static void main(String arg[]) { SortedSet<String> sortedSet = new TreeSet<String>(); sortedSet.add("ZZ"); sortedSet.add("BB"); sortedSet.add("XX"); sortedSet.add("AA"); sortedSet.add("DD"); TreeSet<String> treeSet = new TreeSet<String>(sortedSet); System.out.println("Element from TreeSet: "+ treeSet); } }
Output: Element from TreeSet: [AA, BB, DD, XX, ZZ]
5. Java TreeSet example
As we have learnt, A TreeSet maintain the natural order of element. We can maintain the order of element by provide of explicit Comparator. TreeSet internally uses to compareTo() method to maintain the order. Let’s take various example of TreeSet.
TreeSet with natural order
import java.util.TreeSet; public class TreeSetExample { public static void main(String arg[]) { TreeSet<String> treeSet = new TreeSet<String>(); treeSet.add("Hi"); treeSet.add("Java"); treeSet.add("Goal"); treeSet.add("Learning"); treeSet.add("Website"); System.out.println("Element from TreeSet: "+ treeSet); } }
Output: Element from TreeSet: [Goal, Hi, Java, Learning, Website]
TreeSet with a custom comparator
We can sort the elements by use of custom comparator. We can sort the elements in any order. Here we will see how we can sort the elements based on the different comparators.
import java.util.Comparator; import java.util.TreeSet; class Person { int id; int age; String name; public Person(int id, int age, String name) { this.id = id; this.age = age; this.name = name; } } class AgeComparator implements Comparator<Person> { @Override public int compare(Person person1, Person person2) { if(person1.age == person2.age) return 0; else if(person1.age > person2.age) return 1; else return -1; } } class IdComparator implements Comparator<Person> { @Override public int compare(Person person1, Person person2) { if(person1.id == person2.id) return 0; else if(person1.id > person2.id) return 1; else return -1; } } public class TreeSetExample { public static void main(String arg[]) { TreeSet<Person> personSortById = new TreeSet<Person>(new IdComparator()); personSortById.add(new Person(10, 25, "Ram")); personSortById.add(new Person(5, 22, "John")); personSortById.add(new Person(1, 23, "Krishna")); personSortById.add(new Person(15, 24, "Alley")); personSortById.add(new Person(8, 25, "Jack")); System.out.println("Sorted by Id from TreeSet: "); for(Person person : personSortById) { System.out.println("Person Id: "+ person.id); System.out.println("Person Age: "+ person.age); System.out.println("Person Name: "+ person.name); } TreeSet<Person> personSortByAge = new TreeSet<Person>(new AgeComparator()); personSortByAge.add(new Person(10, 25, "Ram")); personSortByAge.add(new Person(5, 22, "John")); personSortByAge.add(new Person(1, 23, "Krishna")); personSortByAge.add(new Person(15, 24, "Alley")); personSortByAge.add(new Person(8, 25, "Jack")); System.out.println("Sorted by Age from TreeSet: "); for(Person person : personSortByAge) { System.out.println("Person Id: "+ person.id); System.out.println("Person Age: "+ person.age); System.out.println("Person Name: "+ person.name); } } }
Output: Sorted by Id from TreeSet:
Person Id: 1
Person Age: 23
Person Name: Krishna
Person Id: 5
Person Age: 22
Person Name: John
Person Id: 8
Person Age: 25
Person Name: Jack
Person Id: 10
Person Age: 25
Person Name: Ram
Person Id: 15
Person Age: 24
Person Name: Alley
Sorted by Age from TreeSet:
Person Id: 5
Person Age: 22
Person Name: John
Person Id: 1
Person Age: 23
Person Name: Krishna
Person Id: 15
Person Age: 24
Person Name: Alley
Person Id: 10
Person Age: 25
Person Name: Ram