Java HashMap class is one of the most popular Collection classes in java.
The HashMap in java provides the implementation to the Java Map interface.
In this post, we will discuss what is HashMap in java, HashMap internal working, java HashMap iterator, etc.
Here is the table content of the article will we will cover this topic.
1. What is HashMap in java?
2. Important points about HashMap?
3. Create HashMap java?
4. How HashMap increase its size and HashMap load factor?
5. Java hashmap example?
6. Internal working of HashMap?
7. What is hashing in java?
8. How does put method work?
9. How collisions are resolved?
10. How does get method work?
11. HashMap method in java?
12. How to add an object in HashMap by HashMap put() method?
13. How to get values from hashmap in java example?
14. How to remove the element by Java HashMap remove() method?
15. How to replace the value by HashMap replace() method?
16. How to check the map contains key by hashmap containskey() method?
17. How to get java map keyset by hashmap keyset() method?
18. How to get java map entryset by java entryset() method?
19. How to iterate HashMap in Java?
20. ConcurrentHashMap in java?
21. Difference between hashmap and ConcurrentHashMap?
What is HashMap in java?
Java HashMap class extends the AbstractMap class and implements the Map interface, Cloneable interface, and serializable interface.

HashMap is an implementation of the Map interface, so it stores the key and value pair and each pair is known as an entry. In HashMap each value directly associated with a unique key. In JDK, we have a HashMap<K, V> here K denotes the Key and V denotes the Value. The HashMap doesn’t maintain the order of entries(Pair). It means, it doesn’t return the pairs(keys and values) in the same order as in which they have inserted. A HashMap doesn’t allow duplicate keys but it can have duplicate values.
HashMap<K, V> hashMapData = new HashMap<K, V>();
Here K, is the type of type which you want to maintain in HashMap.
V, The type of Value that you want to store in HashMap.
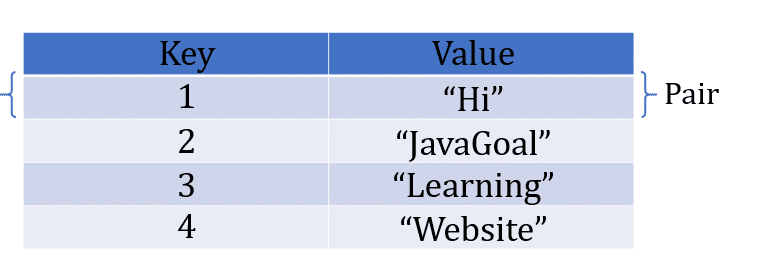
Important points about HashMap
1. HashMap doesn’t allow keys but it can contain duplicate values.
2. HashMap allows only one null key but it can have multiple null values.
3. HashMap doesn’t maintains the order of elements. The order of element can be in any order.
4. In HashMap each value is associated with a unique key.
5. It doesn’t allow Primitive data types like int, char. Because it stores only object references, so we can use wrapper classes of primitive data types.
6. HashMap is not thread safe.
Create HashMap java
To create a HashMap, we need to import the HashMap class from java.util package. The HashMap class provides various constructors that can create objects of HashMap.
There are four types of constructors, we will discuss each constructor with java HashMap example.
HashMap():
The HashMap class provides the default constructor that creates an empty HashMap. It has a default initial capacity is 16 and 0.75 load factor. This constructor creates an object in heap memory and after that, we can perform the operation on it.
import java.util.HashMap; import java.util.Map; class HashMapExample { public static void main(String arg[]) { Map<Integer, String> stringHashMap = new HashMap<Integer, String>(); stringHashMap.put(1, "Hi"); stringHashMap.put(2, "Java"); stringHashMap.put(3, "Goal"); stringHashMap.put(4, "Learning"); stringHashMap.put(5, "Website"); System.out.println("Pair in HashMap: "+ stringHashMap); System.out.println("Get all keys: "+ stringHashMap.keySet()); System.out.println("Get all values: "+ stringHashMap.values()); System.out.println("Get all entry set: "+ stringHashMap.entrySet()); Map<String, Integer> integerHashMap = new HashMap<String, Integer>(); integerHashMap.put("A", 101); integerHashMap.put("B", 121); integerHashMap.put("C", 110); integerHashMap.put("D", 555); System.out.println("Pair in HashMap: "+ integerHashMap); System.out.println("Get all keys: "+ integerHashMap.keySet()); System.out.println("Get all values: "+ integerHashMap.values()); System.out.println("Get all entry set: "+ integerHashMap.entrySet()); } }
Output: Pair in HashMap: {1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website}
Get all keys: [1, 2, 3, 4, 5]
Get all values: [Hi, Java, Goal, Learning, Website]
Get all entry set: [1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website]
HashMap(int initialCapacity)
It is a parameterized constructor that takes a parameter of int type. We can initialize the capacity of HashMap during creation. It has a default load factor that is 0.75. It throws IllegalArgumentException, if the initial capacity is less than 0.
import java.util.HashMap; import java.util.Map; class HashMapExample { public static void main(String arg[]) { Map<Integer, String> stringHashMap = new HashMap<Integer, String>(7); stringHashMap.put(1, "Hi"); stringHashMap.put(2, "Java"); stringHashMap.put(3, "Goal"); stringHashMap.put(4, "Learning"); stringHashMap.put(5, "Website"); System.out.println("Pair in HashMap: "+ stringHashMap); System.out.println("Get all keys: "+ stringHashMap.keySet()); System.out.println("Get all values: "+ stringHashMap.values()); System.out.println("Get all entry set: "+ stringHashMap.entrySet()); Map<String, Integer> integerHashMap = new HashMap<String, Integer>(7); integerHashMap.put("A", 101); integerHashMap.put("B", 121); integerHashMap.put("C", 110); integerHashMap.put("D", 555); System.out.println("Pair in HashMap: "+ integerHashMap); System.out.println("Get all keys: "+ integerHashMap.keySet()); System.out.println("Get all values: "+ integerHashMap.values()); System.out.println("Get all entry set: "+ integerHashMap.entrySet()); } }
Output: Pair in HashMap: {1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website}
Get all keys: [1, 2, 3, 4, 5]
Get all values: [Hi, Java, Goal, Learning, Website]
Get all entry set: [1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website]
HashMap(int initialCapacity, float loadFactor)
This constructor takes two parameters, one is int type and another is float type. We can initialize the capacity and load factor during the creation of HashMap. It throws IllegalArgumentException, if the initial capacity is less than 0 or load factor is negative.
import java.util.HashMap; import java.util.Map; class HashMapExample { public static void main(String arg[]) { Map<Integer, String> stringHashMap = new HashMap<Integer, String>(7, 0.80f); stringHashMap.put(1, "Hi"); stringHashMap.put(2, "Java"); stringHashMap.put(3, "Goal"); stringHashMap.put(4, "Learning"); stringHashMap.put(5, "Website"); System.out.println("Pair in HashMap: "+ stringHashMap); System.out.println("Get all keys: "+ stringHashMap.keySet()); System.out.println("Get all values: "+ stringHashMap.values()); System.out.println("Get all entry set: "+ stringHashMap.entrySet()); Map<String, Integer> integerHashMap = new HashMap<String, Integer>(7, 0.80f); integerHashMap.put("A", 101); integerHashMap.put("B", 121); integerHashMap.put("C", 110); integerHashMap.put("D", 555); System.out.println("Pair in HashMap: "+ integerHashMap); System.out.println("Get all keys: "+ integerHashMap.keySet()); System.out.println("Get all values: "+ integerHashMap.values()); System.out.println("Get all entry set: "+ integerHashMap.entrySet()); } }
Output: Pair in HashMap: {1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website}
Get all keys: [1, 2, 3, 4, 5]
Get all values: [Hi, Java, Goal, Learning, Website]
Get all entry set: [1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website]
HashMap(Map<K,V> m)
This constructor creates a HashMap with a specified map m. It contains all the elements contained in the specified map and use the same mapping as in specified map.
import java.util.HashMap; import java.util.Map; class HashMapExample { public static void main(String arg[]) { Map<Integer, String> stringHashMap = new HashMap<Integer, String>(); stringHashMap.put(1, "Hi"); stringHashMap.put(2, "Java"); stringHashMap.put(3, "Goal"); stringHashMap.put(4, "Learning"); stringHashMap.put(5, "Website"); Map<Integer, String> stringHashMap2 = new HashMap<Integer, String>(stringHashMap); System.out.println("Pair in HashMap: "+ stringHashMap2); System.out.println("Get all keys: "+ stringHashMap2.keySet()); System.out.println("Get all values: "+ stringHashMap2.values()); System.out.println("Get all entry set: "+ stringHashMap2.entrySet()); } }
Output: Pair in HashMap: {1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website}
Get all keys: [1, 2, 3, 4, 5]
Get all values: [Hi, Java, Goal, Learning, Website]
Get all entry set: [1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website]
How HashMap increase its size and HashMap load factor?
A HashMap has default initial capacity 16 and 0.75 load factor. It increases itself when we add elements in it. Internally a HashMap doesn’t increase itself, it creates a new HashMap with double size and copy all the element to new HashMap from old one. We will discuss it in separate topic that is How does HashMap internal implementation work? This process is known as rehashing in java. We have to understand some
Initial capacity of HashMap: Thedefault initial capacity of HashMap is 16, that assigned at the time of its creation. It means, it set the number of buckets a HashMap can hold.
HashMap Load factor: The defaultload factor of HashMap is 0.75. The load factor is used to calculate the threshold after which the capacity of HashMap is to be increased. It directly impacts to the performance of HashMap.
Threshold: Thethreshold tells when to increase the capacity of HashMap. The threshold is calculated by capacity and Load Factor. As we have seen, HashMap has by default capacity is 16 and load factor is 0.75. So it’s threshold will be (16 * 0.75 = 12). It means the when we will add 12th element, it creates a new HashMap with double capacity and copy all the elements from old to new one.
Rehashing in java – It is the process that creates a new HashMap with double capacity of old HashMap when size reaches its Threshold. It copies all the objects from old HashMap to new HashMap. Rehashing is very costly process because it copies all the data. Suppose if HashMap rehash 100 times then it wastes a lot of effort in memory or performance.
We can stop the rehashing if we initialize the capacity according to use. The rehashing will never be done if capacity is higher than threshold. But by keeping it higher size it increases the time complexity of iteration.
Java hashmap example
We have seen many examples of HashMap in the above discussion. Let’s take another example of HashMap for a user-defined class.
Suppose we want to store a record of Persons. We will use a HashMap and store each person record based on a unique key. Here we will choose PAN as a unique key.
import java.util.HashMap; class HashMapExample { public static void main(String arg[]) { HashMap<String, Person> data = new HashMap<String, Person>(); data.put("ABC123", new Person("ABC123", "Ram", "CHD")); data.put("A3B2A1", new Person("A3B2A1", "John", "KKR")); data.put("AA1BB2", new Person("AA1BB2", "Krishna", "YNR")); data.put("BB33AA", new Person("BB33AA", "Sham", "DELHI")); data.put("ACB123", new Person("ACB123", "Kelly", "SHN")); // Get particular object System.out.println("Get only one Person: "+ data.get("AA1BB2").toString()); // Get all keys System.out.println("All keys: "+ data.keySet()); // Get all values System.out.println("All values: +"+ data.values()); // Transverse all objects System.out.println("Transverse all Objects:"); for(String key : data.keySet()) System.out.println(data.get(key)); } } class Person { String PAN; String name; String address; public Person(String PAN, String name, String address) { this.PAN = PAN; this.name = name; this.address = address; } public String getPAN() { return PAN; } public String getName() { return name; } public String getAddress() { return address; } @Override public String toString() { return this.getName() + ", " + this.getPAN()+ ", " + this.getAddress(); } }
Output: Get only one Person: Krishna, AA1BB2, YNR
All keys: [AA1BB2, BB33AA, A3B2A1, ACB123, ABC123]
All values: +[Krishna, AA1BB2, YNR, Sham, BB33AA, DELHI, John, A3B2A1, KKR, Kelly, ACB123, SHN, Ram, ABC123, CHD]
Transverse all Objects:
Krishna, AA1BB2, YNR
Sham, BB33AA, DELHI
John, A3B2A1, KKR
Kelly, ACB123, SHN
Ram, ABC123, CHD