Here is the table content of the article will we will cover this topic.
1. What is the functional interface in Java 8?
2. How to use the Function interface?
3. Advantages of functional interface?
What is the function interface in Java 8?
The Function interface is defined in the java.util.function package. It contains one abstract method that is known as apply() method. This interface accepts the input and provides output. It takes one parameter as input and produces the output.
This interface is used in a lambda expression and method reference to evaluate the condition.
@FunctionalInterface public interface Function<T, R> { // Body of Interface }
Where T, The type of input to the function.
R, The type of the result of the function.
R apply(T t) method: This method is used to perform the operation on the given input and produce the result. Its return type is R, where R is a data type specified while using the interface.
R apply(T t)
This interface contains a few extra methods but since they all come with a default implementation, you do not have to implement these extra methods.
This method is very useful whenever you want to perform the operation on the object and want results accordingly. The programmer must have to specify the data type of input and result.
Here T is used for the input data type and R is used for the result.
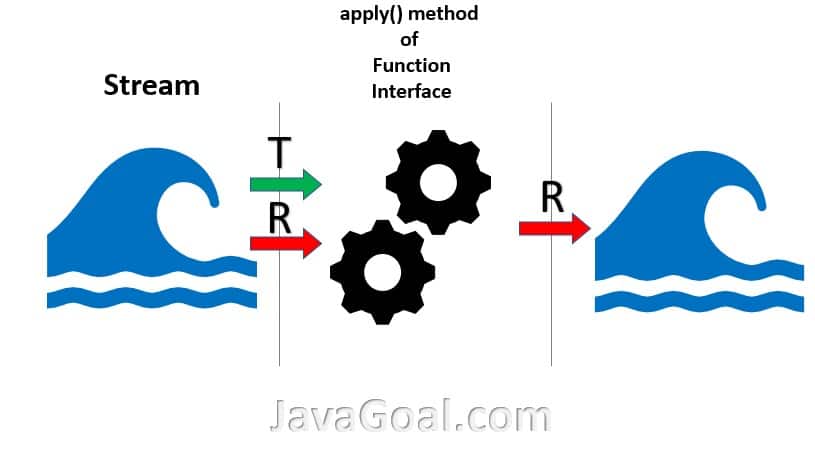
How to use the Function interface?
First of all, we will see how to use the Function interface without a lambda expression.
import java.util.function.Function; public class ExampleOfFunctionWithoutLambda { public static void main(String[] args) { Function<String, String> function = new Function<String, String>() { @Override public String apply(String string) { return string.toUpperCase(); } }; System.out.println(function.apply("Hi")); System.out.println(function.apply("Hello")); } }
Output: HI
HELLO
You can perform the action according to requirements. Let’s say create an example for the different data types. In this example, we are providing an Integer type for input and a String type for Result.
import java.util.function.Function; public class ExampleOfFunctionWithoutLambda { public static void main(String[] args) { Function<Integer, String> function = new Function<Integer, String>() { @Override public String apply(Integer integer) { return integer.toString(); } }; System.out.println(function.apply(123)); System.out.println(function.apply(55555)); } }
Output: 123
55555
Now, we will see how you can use the Function interface with a lambda expression:
import java.util.function.Function; public class ExampleOfFunctionWithLambda { public static void main(String[] args) { Function<Integer, String> function = (value) -> value.toString(); System.out.println(function.apply(123)); System.out.println(function.apply(55555)); } }
Output: 123
55555
Advantage of Function
1. By use of Function Interface, you can move the operational code to one place.
2. It improves code maintenance because if you want to perform operations on the object then you have to make it in a central place.
Advantage of Predicate should be Advantage of Function (Title is wrong)
Thanks…I have updated it
I truly appreciate what you have done with the java goal. One stop solution before my interview