Deque interface in java is part of Collection framework. In java Deque interface extends the Queue interface and its available in java.util package. It maintains the orders the elements in FIFO(First In First Out) or LIFO(Last In First Out) manner. The Deque is related to the double-ended queue that supports addition or removal of elements from either end of the data structure. The Deque is faster than LinkedList and Stack. The Deque interface has its implementation in ArrayDeque class.

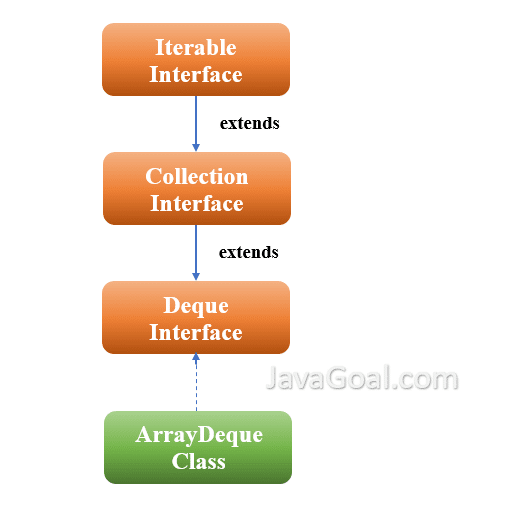
Methods in Queue:
1. add() method: This method is used to add elements at the tail in the queue. As you know the Queue interface is implement by PriorityQueue and LinkedList. In both classes add method work differently. In LinkedList the element will be add at the last. But in PriorityQueue, element will be added according to the priority in case of priority queue implementation.
2. peek() method: This method is used to get the head(First) element of queue without removing it. If the queue is empty it will return.
3. element() method: This method is also used to get the head(First) element of queue without removing it. It is very similar to peek() method. If queue is empty it will throw NoSuchElementException.
4. remove() method: This method is used to remove and get the head(First) element from the queue. If queue is empty it will throw NoSuchElementException.
5. poll() method: This method is also used to remove and get the head(First) element from the queue. It is very similar to remove() method. If queue is empty it will return null.
6. size() method: This method returns the number of elements presents in the queue.
import java.util.LinkedList; import java.util.Queue; public class ExampleOfQueue { public static void main(String[] args) { Queue<Integer> numbers = new LinkedList<Integer>(); // Adding some number/elements in queue by use of add() method numbers.add(1); numbers.add(2); numbers.add(3); numbers.add(4); numbers.add(5); // Showing all data from queue for(Integer num : numbers) System.out.println("Elements from the queue: "+num); // Getting the head(First) element from queue by use of peek() method. int firstNumberByPeek = numbers.peek(); System.out.println("Getting the by peek() method: " + firstNumberByPeek); // Getting the head(First) element from queue by use of element() method. int firstNumberByElement = numbers.element(); System.out.println("Getting the by element() method: " + firstNumberByElement); // Removing the head(First) element from queue by use of remove() method. int removedElement = numbers.remove(); System.out.println("Removing element by remove() method: " + removedElement); // Removing the head(First) element from queue by use of poll() method. int removedElementByPoll = numbers.poll(); System.out.println("Removing element by poll() method: " + removedElementByPoll); // Getting size of Queue int size = numbers.size(); System.out.println("Size of queue: " + size); } }
Output: Elements from the queue: 1
Elements from the queue: 2
Elements from the queue: 3
Elements from the queue: 4
Elements from the queue: 5
Getting the by peek() method: 1
Getting the by element() method: 1
Removing element by remove() method: 1
Removing element by poll() method: 2
Size of queue: 3