We have learned about HashSet in java and how we can add elements in HashSet? Now we will see how to remove element from HashSet. We can use remove() method of HashSet or by use of iterator. Let’s discuss both ways in java remove from HashSet
remove(Object o) method
The remove(Object o) method is used to remove the given object o from the HashSet. It returns boolean type value either true or false. If the given object exists in HashSet then it will remove the object and return true. But if the object doesn’t exist it return false.
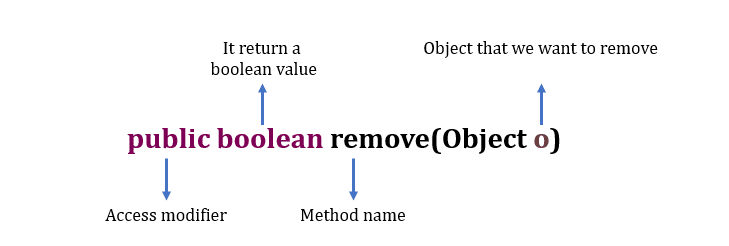
access modifier, The access modifier is public, So it can be accessible from everywhere.
return type, Its return type is boolean either returns true or false.
Object o, The object which we want to remove from HashSet.
public boolean remove(Object o) { return map.remove(o)==PRESENT; }
Let’s discuss it with example how to do in java remove from hashset
import java.util.HashSet; public class HashSetExample { public static void main(String[] args) { HashSet<String> setOfNames = new HashSet<String>(); setOfNames.add("Java"); setOfNames.add("Goal"); setOfNames.add("Website"); boolean isRemoved = setOfNames.remove("Goal"); System.out.println("Is successfully removed: "+ isRemoved); isRemoved = setOfNames.remove("Ok"); System.out.println("Is successfully removed: "+ isRemoved); System.out.println("Object present in HashSet: "+setOfNames); } }
Output: Is successfully removed: true
Is successfully removed: false
Object present in HashSet: [Java, Website]
You have seen the HashSet internally uses the HashMap. So when we remove the object from HashSet by use of remove(Object o) method, it internally removes the object from HashMap.It checks whether the object if present or not? If the object exists in HashSet it will remove it and return true otherwise false.
By use of Iterator
We can remove the object from HashSet by use of iterator. We can traverse the objects of HashSet and remove it. We use the iterator() method to get an object of iterator.
public Iterator<E> iterator()
In the first step, we will transverse the objects of HashSet.
In the second step, We will compare the object that we want to remove.
In the third step, We will remove the object from HashSet.
import java.util.HashSet; import java.util.Iterator; public class HashSetExample { public static void main(String[] args) { HashSet<String> setOfNames = new HashSet<String>(); setOfNames.add("Java"); setOfNames.add("Goal"); setOfNames.add("Website"); Iterator<String> iterator = setOfNames.iterator(); while(iterator.hasNext()) { if(iterator.next().equals("Java")) iterator.remove(); } System.out.println("Object present in HashSet: "+ setOfNames); } }
Output: Object present in HashSet: [Goal, Website]
Can you provide an example for set.remove that works with non-string objects? I have been googling all morning for this and all I find are examples working with sets that contain String objects.
Example of what I mean…
suppose I have a method with:
Set widgets = new HashSet();
Widget widget1 = new Widget();
widgets.add(widget1);
Widget widget2 = new Widget();
widgets.add(widget2);
return widgets;
and then in the main function after this method is called where the above returned “widgets” is store in another HashSet called “main_widgets”, I want to remove an element from it:
main_widgets.remove(widget1);
does not work, because in main it no longer can identify widget1. So how do you do this?