Most of the programmers know about the Object class in Java. But some of them want to know what’s the need for Object class was. Why it was introduced? In this article, we will discuss the Object class in Java. Here is the table content of the article will we will cover on this topic.
What is Object class?
Why object class is a superclass?
What is the need for an Object class?
Object class method and use of the method?
1. toString() method
2. equals() method
3. hashCode() method
4. getClass() method
5. finalize() method
6. clone() method
7. wait() method
8. notify() method
9. notifyAll() method
What is Object class?
1. Object class is super most class
In Java, Object is the super most class that is present in java.lang package. It means every class in java is a subclass, which inherited the properties from the Object class(Super most). Every class in Java is directly or indirectly inheriting the properties from the Object class.

class A { //Body of class A }
As you can see, class A is not inheriting any class, it means it inheriting the Object class. Here you can see the Object class is directly inherited by class A.
Class C { //Body of class C } Class B extends C { //Body of class B }
In the above example, class B is inheriting a class C, which means it is not directly inheriting the Object class. Here you can see the class C is directly inherited by class B. But Object class is indirectly inherited by class B. because somewhere class C inheriting the Object Class.
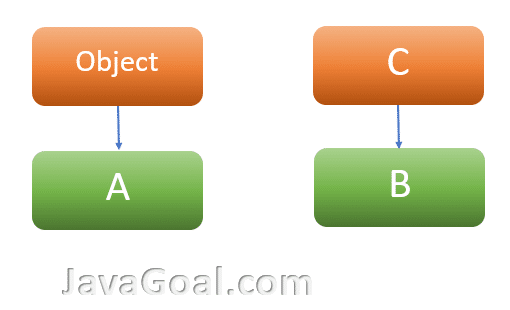
NOTE: All the method of Object class is available to all Java classes.
2. Object class provides common behavior
As you know every class inherits an Object class. The Object class provides some methods that are extended by each class of Java. So, The Object class provides common behaviors to all the objects. Like comparison of an object, cloning of objects or object can be notified, etc.
NOTE: If you want to refer any object whose type is unknown then the Object class is beneficial. Because Object is parent class, so you can refer to the child class object.
Object obj = new A(); Object obj = new B();
Why object class is a superclass? OR
What is the need for an Object class?
As we discussed, Object is super most class in Java and each class inheriting its properties. Now the question arises why the Object class is superclass? What is the need for an Object class?
1. Code reusability
The Object class has serval methods that have common and generic logic for each subclass. If any class is not satisfying with common logic, then that class can override the method and provide implementation according to need.
For example, The equals() method of the object uses to compare the Objects. But you can override it and provide the implementation according to the requirement. In Java, each object has some common properties inherited from the Object class.
2. Common functionalities
There is a lot of functionality that comes from Object class. Each object needs these common functionalities. Like compare, hashcode, tostring().
Object class method and use of the method
Object class has a various method that is inherited by all class. Here we will discuss all methods of Object class and also discuss the use of the method.
toString() method
The toString() method is defined in Object class which is the super most class in Java. This method returns the string representation of the object. Let’s have a look at the code.
public String toString() { return getClass().getName() + "@" + Integer.toHexString(hashCode()); }
As you can see in the above code, its return type is String. It returns the class name of the object and appends the hashCode of that object.
As you already know, every class in java is the child of the Object class. It means each class inheriting the toString() method from the Object class.
Whenever a user tries to print the object of any class then the java compiler internally invokes the toString() method.
You can read this article in detail.
equals() method
The equals() method is defined in Object class which is the super most class in Java. This method utilizes to compares two objects, and it returns the boolean value based on the comparison. Let’s have a look at the code.
public boolean equals(Object obj) { return (this == obj); }
As you can see in the above code, it compares the given object obj to “this” object. Here “this” is the object on which the method is called.
As you already know, every class in java is the child of the Object class. It means each class inheriting the equals() method from the Object class. You can override the equals() method as per your functionality. Suppose you want to compare the object’s own equality condition. Then it is recommended to override the equals(Object obj) method.
You can read this article in detail.
hashCode() method
The hashCode() method is defined in Object class which is the super most class in Java. This method returns a hash code value for the object. Let’s have a look at the code.
public native int hashCode();
1. It returns an integer value of the object memory address. Some programmer thinks it is the memory address of an object which is not correct. It’s the integer representation of the object’s memory address.
2. It is a native method. Through the native method, it is written in C/C++ language. As you know, in java we can’t find the memory address of the object, so that the hashCode() method is written in C/C++ that helps find the memory address.
You can read this article in detail.
getClass() method
The getClass() method is defined in Object class which is the super most class in Java. This method returns the object of the Class class. As you know String, Array, etc are the classes in java. In the same manner ”Class” is also a class in Java. It returns the runtime class of this object. This method is also a native method. It is a final method, so we don’t override it.
Let’s have a look at code.
public final native Class<?> getClass();
It is a native method. Using the native method, it is written in C/C++ language.
You can read this article in detail.
finalize() method
The finalize() method is defined in Object class which is the super most class in Java. This method is called the Garbage collector, just before the destruction of the object from memory. The Garbage collector is used to destroy the Object which is eligible for garbage collection.
Let’s have a look at the code.
protected void finalize() throws Throwable { }
The finalize() method is a protected and non-static method of Object class. As you know, Object is super most class and each class inherits the Object class, Which means the finalize() method is available in each class.
You can read this article in detail.
clone() method
The clone() method is defined in Object class which is the super most class in Java. This method is used to create an exact copy of an object. It creates a new object and copying all data of the given object to that new object.
Let’s have a look at the code.
protected native Object clone() throws CloneNotSupportedException;
It is a native method. By means of the native method, it is written in C/C++ language.
You can read this article in detail.
wait() method
The wait() method is defined in Object class which is the super most class in Java. This method tells the calling thread (Current thread) to give up the lock and go to sleep until some other thread enters the same monitor and calls notify() method or notifyAll() method. It is a final method, so we can’t override it.
Let’s have a look at the code.
public final void wait() throws InterruptedException { wait(0L); }
You can read this article in detail.
notify() method
The notify() method is defined in Object class which is the super most class in Java. It is used to wakes up only one thread that is waiting on the object, and that thread starts execution. It is a final method, so we can’t override it.
Let’s have a look at the code.
public final native void notify();
Suppose multiple threads are waiting for an object, then it will wake up only one of them. Only one thread gets the notification, and the remaining thread has to wait for further notification.
You can read this article in detail.
notifyAll() method
The notifyAll() method is defined in Object class which is the super most class in Java. It is used to wakes up all threads that are waiting on the given object. Therefore, This method gives the notification to all waiting threads of an object.
Whenever we use the notifyAll() method and multiple threads that are waiting for the notification, then all the threads got the notification. But it will execute all the threads one by one. All thread gets the notification but only one thread gets a lock. So that only one thread can execute at a time after that next that will be executed. It is a final method, so we don’t override it.
Let’s have a look at the code.
public final native void notifyAll();