A method is a block of code that can perform certain actions as per requirement. In java, almost all operations are performed within a method. In this post, we will learn What is method in java, types of method, define method in java, how to create method in java and Calling methods in java.
Here is the table content of this article that we will cover.
1. What is the method in java?
2. How to define method in java OR How to create method in java?
3. Calling methods in java?
4. How to use return type in java?
5. Instance method in java?
6. Difference between static and non static methods in java?
What is method in java?
A method is just a block or collection of statements that perform some specific tasks. To perform the task, we call the method so that compiler executes the statements written in the method. A method provides the organized structure to the java program, and we can reuse the code. We will discuss the advantages ad usage of the method in detail so that you can relate the things. Methods are also known as functions in java.
Firstly, you have to understand the concept of method and how does it work?
Suppose you want to perform a task in the program then you have to do two things:
- Create a method and define your task within the method.
- Invoke/Call the method so that compiler could execute your task.
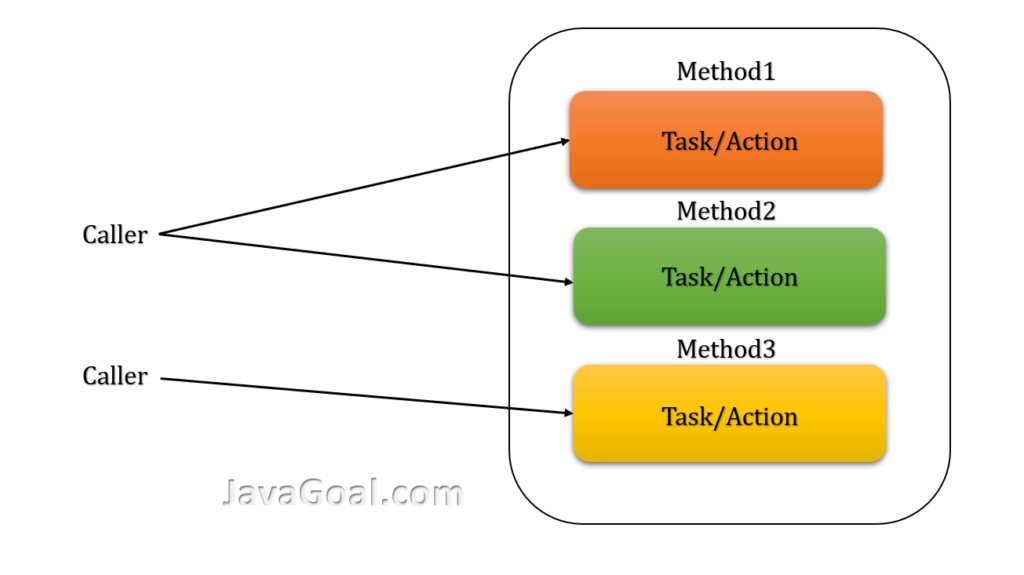
How to define method in java OR How to create method in java
To create a method, we have to follow the syntax and provide the values to all attributes of the method. Let’s see the syntax of the method:

Access Specifier: The Access specifier is also known as Access modifier in java that specifies the visibility of the method. You can read it in detail from the Access modifier in java.
- private: If we declare a method with private access specifier, then the method will be accessible only within the class.
- protected: The protected access modifier has less restriction than private. If we use it with method, then it will be accessible within the same package or subclasses in a different package.
- default: The default access modifier accessible within the same package. If we don’t specify any access specifier in the method declaration, Java uses default access specifier by default.
- public: The public access modifier doesn’t apply ay restriction. The public method is accessible by all classes in our application.
You can see different programs of access modifier in detail.
Return Type: The return type tells which type of data value a method will return. A method can return a type of value like primitive or non-primitive. But if you don’t want to return any value then use the void keyword. You can see different uses of return type here.
Method Name: Like a variable, the method has a name and the rules for variable name apply to the method name as well. When we define the method, we give the method name in java. To give a meaningful name is always considered a good practice. We should start the method name with a lowercase letter. If the method name has more than one word, then use the camel case.
Parameter list: The parameter list/ Argument list is used when we want to send the data in method and want to perform some action on it. We can send any number of parameter lists separated by a comma and enclosed in the pair of parentheses. But here we tell the data type and name of each parameter. We can leave it as blank as it is not mandatory.
Exception list: Many times we want to throw exceptions from the method. We can specify the exceptions that we want to throw.
Method Body: It is the part that contains the action/task of the method. Here we write our logic or set of statements that perform the operation. It is enclosed within the pair of curly braces.
Let’s see some valid example of method
private void sum() { // Body of method } private void sumOfMarks() { // Body of method } void getData() { // Body of method } protected int sum(String marks) { // Body of method } public void sum() { // Body of method } private ArrayList<Integer> sum() { // Body of method }
Calling methods in java
After the creation of the method, we can invoke or call the method so that it can perform the task. Here we will see how we do function calls in java. When we call the method the control transfer to the method and execute the statements of the method.
public class Example { public static void main(String[] args) { int a = 5; int b = 8; Example obj = new Example(); obj.findGreater(a, b); } public void findGreater(int num1, int num2) { int max; if (num1 < num2) max = num2; else max = num1; System.out.println(max); } }
Output: 8
How to use return type in java
The return type of method tells the compiler about the data type of value that will be returned by the method. A method may or may not return any value. When you perform some operations in method and you want to get back the result of the operation to the caller then method must have a return type. But when you don’t want any result then you don’t need any return type. In this case, you can use void keyword in java. Let’s discuss it with some examples.
void keyword in java
Firstly, we will see when we don’t want to return a value from the method. I this case we have to use the void keyword in java with method. The void keyword means we will not return anything. Let’s see a java void function:
public class MainClass { public static void main(String args[]) { MainClass obj = new MainClass(); obj.sum(); } /** * The sum method has public access modifier * It return type is void * name of method is sum */ public void sum() { System.out.println("Sum of Numbers:" + (5+5)); } }
Output: Sum of Numbers:10
class Student { int rollNo; String name; Student(int rollNo, String name) { this.rollNo = rollNo; this.name = name; } public int getRollNo() { return rollNo; } public String getName() { return name; } } public class MainClass { public static void main(String args[]) { Student student1 = new Student(101, "Vindhu"); Student student2 = new Student(102, "Ram"); Student student3 = new Student(103, "Krishna"); MainClass obj = new MainClass(); obj.printStudentDetails(student1); obj.printStudentDetails(student2); obj.printStudentDetails(student3); } public void printStudentDetails(Student student) { System.out.println("RollNo: "+student.getRollNo()+ " "+"Name: "+ student.getName()); } }
Output: RollNo: 101 Name: Vindhu
RollNo: 102 Name: Ram
RollNo: 103 Name: Krishna
How does control flow work when we call method and it get executed.
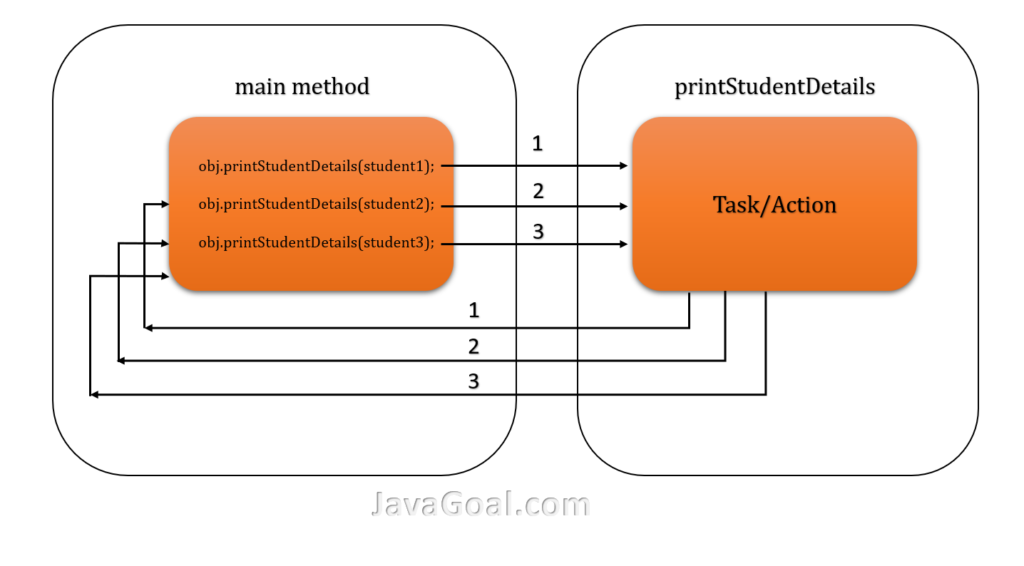
Returning a value in java
Let’s see when we want to return the value from a method. I this we define the data type of value that we want to return. A method return types java defines the value type that it will return. Let’s see with example in which java method returns boolean value.
boolean return type in java method
public class ReturnExample { public static void main(String args[]) { ReturnExample obj = new ReturnExample(); boolean hasGreaterValue = obj.isGreaterThanFive(8); System.out.println("Is value greater than 5: " + hasGreaterValue); } /** * This method returns a boolean type value * @param number is type of int * @return */ public boolean isGreaterThanFive(int number) { return number > 5; } }
Output: Is value greater than 5: true
How does control flow work when we call method and return boolean java value?
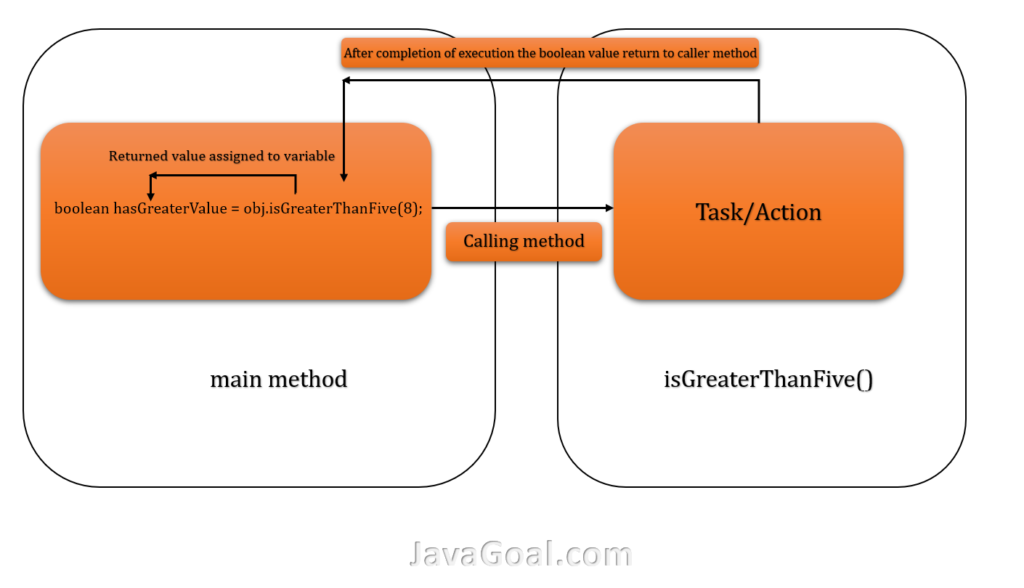
In the above example, you have seen, we are calling a method / Invoking a method and it is returning the boolean value from method. Always remember the return type and data type of returned value must be compatible. If they are not compatible the JVM shows a compilation error.
Java return ArrayList
import java.lang.reflect.Array; import java.util.ArrayList; class Student { int rollNo; String name; Student(int rollNo, String name) { this.rollNo = rollNo; this.name = name; } public int getRollNo() { return rollNo; } public String getName() { return name; } } public class MainClass { public static void main(String args[]) { Student student1 = new Student(101, "Vindhu"); Student student2 = new Student(102, "Ram"); Student student3 = new Student(103, "Krishna"); MainClass obj = new MainClass(); ArrayList<Student> listOfStudent = new ArrayList<Student>(); listOfStudent.add(student1); listOfStudent.add(student2); listOfStudent.add(student3); ArrayList<Integer> rollNumbers = obj.printStudentDetails(listOfStudent); System.out.println("All roll numbers are: "+rollNumbers.toString()); } /** * This method prints returns the ArrayList of Integer * It takes one parameter that is also type of ArrayList * @param studentsData * @return */ public ArrayList<Integer> printStudentDetails(ArrayList<Student> studentsData) { ArrayList<Integer> listOfRollNo = new ArrayList<>(); for (Student student : studentsData) listOfRollNo.add(student.getRollNo()); return listOfRollNo; } }
Output: All roll numbers are: [101, 102, 103]
Instance method in java
A non-static method declared within the class is known as the instance method. If you are not familiar with static method, then you should read it so that you can find the difference.
An instance method or non-static method doesn’t belong to the class, it belongs to the instance of the class. The non-static methods are not common for all instances. So, it is mandatory to create an object to invoke an instance method.
public class InstanceMethodExample { public static void main(String [] args) { //Here we are creating an object of the class InstanceMethodExample obj = new InstanceMethodExample(); //invoking/Calling the instance method System.out.println("Sum of Numbers: " + obj.sum(12, 13)); } /** * This method is instance method because it is non-static * @param a * @param b */ public int sum(int a, int b) { return a+b; } }
Output: Sum of Numbers: 25
Difference between static and non static methods in java
Here we will see the difference between static method and instance method or we can say instance method vs static method.
static method
- When we use static keyword with method, that method is known as static method. A static method directly belongs to class instead of instances. We can invoke a static method by use of class name. This method is also known as class method.
- In a static method, we can access only the static variables and static methods. We can’t access any non-static variable and non-static method.
- Static method uses the early binding. They bind with class a compile time because static method loads in memory during the class loading.
- A static method can’t be override because it binds with class at compile time. You can’t override it.
- The static methods are stored in static memory pool. They consume less memory because they load at one time and doesn’t create multiple copies in memory.
- We can’t use this and super keyword within the static method. Because this and super keywords belongs to the instance. The static method is not belonging to any instance, so compiler give an error if we use this and super keyword within method.
- Static methods can be called by null reference because these methods don’t belongs to object reference. When we call a static method by object the JVM finds the class name of object and invokes the object. So, if we use null reference then JVM doesn’t throw NullPointerException.
Non-static method(Instance method)
- When we create a method and if don’t use static keyword with the method. It means It’s a non-static or instance method. The instance method or non-static methods are binds with instance instead of class. We can access it by use of instance/object.
- In non-static method, we can access the static and non-static variables as well as static and non-static methods.
- But non-static methods bind at runtime. Because they get load in memory when any object invokes them.
- A non-static method can be override. Because it binds at runtime.
- But non static methods are stored in heap memory. They consume much memory during the execution because they create a new copy in memory when any object invokes them.
- In a non statice or instance method we can use the this and super keyword. Because both keyword refers the current instance/object.
- But non static method can’t be accessible through the null reference. It throws NullPointerException.