We have seen how to add the element in ArrayList, Now we will see how to remove element from ArrayList java. We can use the remove() method, removeIf() method to remove the element from ArrayList. This method is overloaded to perform multiple operations based on different parameters.
Here is the table content of the article will we will cover this topic.
1. remove(Object o) method
2. remove(int index) method
3. removeAll(Collection c) method
4. removeIf(Predicate filter) method
remove(Object o) method
In java ArrayList remove(Object o) method is used removes the first occurrence of the specified element from ArrayList. Its return type is boolean. If an ArrayList contains duplicate elements the remove method removes the only the first occurrence of the element. Let’s see remove element from ArrayList java
boolean remove(Object obj);
Where, Object represents the type of class in ArrayList .
obj is the element which you want to remove from the ArrayList .
return type: Its return type is boolean. It can return either true or false.
If specified obj presents in ArrayList then it returns true after removal of obj otherwise it returns false.
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("Learning"); listOfNames.add("GOAL"); System.out.println("Before remove method list: "+listOfNames); boolean isRemovedSucessfully = listOfNames.remove("GOAL"); System.out.println("Is element is removes successfully = "+isRemovedSucessfully); // This element doesn't exists isRemovedSucessfully = listOfNames.remove("NOW"); System.out.println("Is element is removes successfully = "+isRemovedSucessfully); System.out.println("After remove method list: "+listOfNames); } }
Output: Before removing method list: [JAVA, GOAL, Learning, GOAL]
Is the element is removed successfully = true
Is the element is removed successfully = false
After removing method list: [JAVA, Learning, GOAL]

remove(int index) method
The remove(int index) method is used removes the element at the specified position from ArrayList. It returns the element after removing the element. When we want to remove the element based on index value we should use the remove(int index) method.
E remove(int index);
Where index, the index of the element to be removed.
E, the element that was removed from the list.
throw, IndexOutOfBoundsException if index is invalid.
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("Learning"); listOfNames.add("GOAL"); System.out.println("Before removing method list: "+listOfNames); String removedElement1 = listOfNames.remove(3); System.out.println("The element is removed successfully = "+removedElement1); String removedElement2 = listOfNames.remove(0); System.out.println("The element is removed successfully = "+removedElement2); System.out.println("After removing method list: "+listOfNames); } }
Output: Before removing method list: [JAVA, GOAL, Learning, GOAL]
The element is removed successfully = GOAL
The element is removed successfully = JAVA
After removing method list: [GOAL, Learning]

removeAll(Collection c) method
This method is used to remove all the elements of the specified collection from ArrayList. Its return type is boolean. It removes all the elements from the ArrayList that matches with the given collection. It removes all occurrence of elements even they are duplicate or not.
boolean remove(Collection c);
Where c, collection containing elements to be removed from this list.
return type: Its return type is boolean. It can return either true or false.
If specified collection c presents in ArrayList then it returns true after removal of c otherwise it returns false.
throws ClassCastException, if the class of an element of the list is incompatible with the specified collection.
throws NullPointerException, if the list contains a null element and the specified collection does not permit null elements.
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("Learning"); listOfNames.add("NEW"); listOfNames.add("SITE"); listOfNames.add("Learning"); System.out.println("Before removal names from list = "+listOfNames); ArrayList<String> listOfNames2 = new ArrayList<String>(); listOfNames2.add("Learning"); listOfNames2.add("NEW"); listOfNames2.add("SITE"); // It removes the collection boolean isRemovedSucessfully = listOfNames.removeAll(listOfNames2); System.out.println("Are all elements removed of specified collection = "+isRemovedSucessfully); System.out.println("After removal names from list = "+listOfNames); } }
Output: Before removal names from list = [JAVA, GOAL, Learning, NEW, SITE, Learning]
Are all elements removed of specified collection = true
After removal names from list = [JAVA, GOAL]

removeIf(Predicate<? super E> filter) method
This method was introduced in Java 8. Before moving further, you should read about Predicate in java. The removeIf(Predicate<? super E> filter) is used to remove all elements from the ArrayList if the predicate satisfies the condition. Its return type is boolean. It will return True if the predicate satisfies the condition and able to remove some elements. Java 8 has an important in-built functional interface which is Predicate.
boolean removeIf(Predicate<? super E> filter)
Where filter, represents a reference of predicate which returns true for elements to be removed.
fromIndex, the index of the first element to be removed.
toIndex, index after the last element to be removed.
throw NullPointerException, if the specified filter is null.
package package1; import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("RAVI"); listOfNames.add("NEW"); listOfNames.add("WWW"); System.out.println("Before removing the Names from list = "+listOfNames); // It removes the all element which satisfy the condition of predicate listOfNames.removeIf(name -> (name.length() == 3)); System.out.println("After removing the Names from list = "+listOfNames); } }
Output: Before removing the Names from list = [JAVA, GOAL, RAVI, NEW, WWW]
After removing the Names from list = [JAVA, GOAL, RAVI]
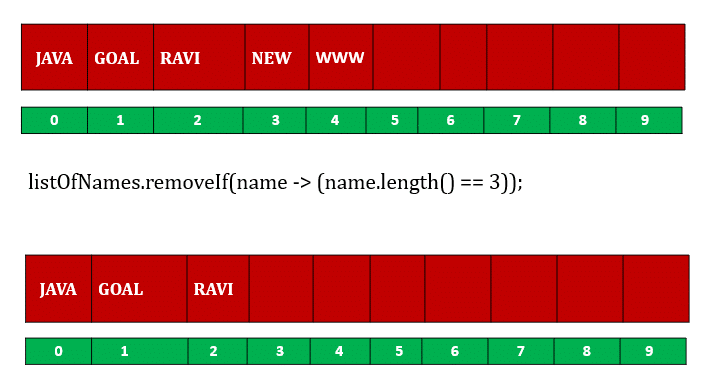