In this article, we will discuss the most important concept of OOPs which is class and object in Java. First of all, we will discuss what is class in java and after that move on to what is object in java.
Here is the table content of the article will we will cover this topic.
1. What is class in java?
2. How to create a class?
3. What is object in java?
4. How to create an object of class?
5. Memory representation of object after the declaration?
6. Initializing an object?
7. Memory representation of object after initialization?
8. A real-life example of class and object in Java?
What is the class in java?
class is the basic concept of OOPs. A class is defined as a blueprint/prototype. You can create an individual object by use of a class. A class can represent the set of properties and methods that are common for all the objects of a class.
In the Java class, we define a pattern/blueprint which is followed by every object. Before moving further we must need to know what a java class consists. What’s the meaning of blueprint here? Here is the blueprint print of the java class that consists of a number of things.
class <class_name> { members of class }
This is the common pattern of every class in Java. We will discuss it in detail later but as of now, we are giving an overview of the syntax.
class: To create a class in java, we use the keyword class. This keyword tells the compiler to create a class.
<class_name>: After the use of the class keyword, we can specify the name of the class.
{
member/behavior of class
}
Body of class: It contains the member/behavior of class.
What are members of Class in java?
A class contains two types of members in java. One is the field and another is the method. Each class contains some fields and methods. A class is incomplete without defining the members of the class. You must think what is the meaning of members of class? Let’s take the example of a Car.
Here the car is a class and the car has some properties and operations also. These properties and operations are the members of the car. These properties are known as fields and operations as methods.
Properties/ fields: Each car has color, body, shape, brand name, etc.
Operations/ Method: Start, stop, accelerator, break these are the operation of the car.
In the same manner, Each Java class contains some field/properties and method/operations. It depends on the developer and what type of operation he/she wants to do.
Real-life example: A house architecture is a blueprint(class) for us. We can create any number of houses (Objects) by using the same blueprint. A blueprint(class) of a house can represent the interior or exterior (properties and method) of a house.
Here, we have a blueprint of a house.
We can define the number of rooms,
galleries, etc. When we are done with the blueprint then we can start the construction of our house.
In JAVA, first, we defined our class and its properties and method after that we can create an object of the class. So basically, an object is a real entity.

How to create a class
Access_Specifier class class_Name { // properties/attributes/variables // behavior/operations/method }
Access_Specifier: A class can be private, protected, public and default access. You can read it in more detail.
class: It is a keyword that should be placed before the class name.
class_Name: You can give any name to class according to your choice of data but there are some naming conventions for a class name you can refer the link.
Body of class: The body of class start with open curly bract “{” and ends with close curly bract “}”.
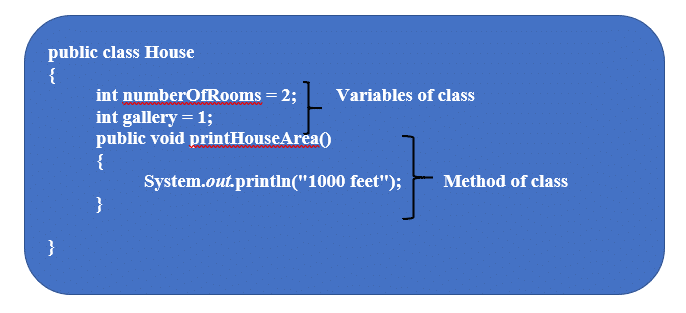
Naming concentrations:
- It should start with the uppercase letter.
- It should be a noun such as Color, Button, System, Thread, etc.
- Use appropriate words, instead of acronyms.
What is object in java?
Every entity is an object. Each entity has some properties and behavior. In the same manner, the object has also properties and behaviors. In technical terms, properties are the variables and behavior are the methods.
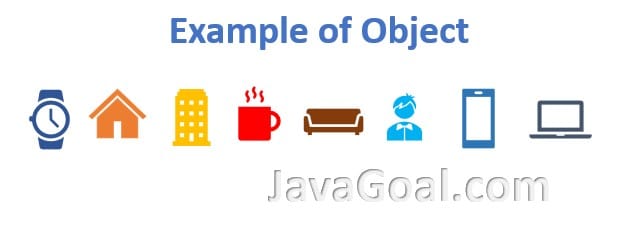
Real-life example: In real life, A Mobile, pen, table, car, house, etc. are entities because they have some properties and behavior.
If Mobile is an object it has some properties and behavior.
Properties: color, size, shape, company name.
Behavior/Operation: Call, music, camera
How to create an object of class
In java, an object is an instance of a class. An object contains some memory space in heap memory because the object contains the data.
We will declare a reference variable as we declared variables like data_Type name. The reference variable holds the object.
House newHouse;
Here House is a class and newHouse is a reference variable. The object’s newHouse value will be null until an object is created and assigned to it. Simply declaring a reference variable does not create an object.
Memory representation of object after the declaration
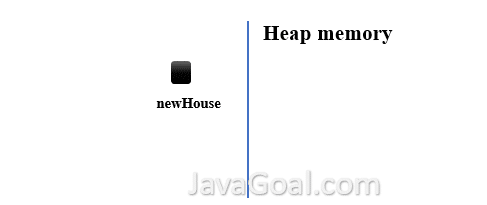
Creating an object and assigning to the reference variable
There are multiple ways to create a new object. We will discuss them later. As of now, we will create object by use of new operator. To create an object in memory we are using the new operator. The new operator instantiates a class by allocating memory for a new object and returning a reference to that memory. The new operator invokes the class constructor.
House newHouse = new House();
When a new house() statement executed by compiler it will allocate some memory in heap memory and returning a reference to that memory.
Memory representation of object after initialization
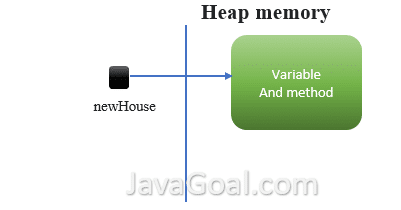
Let’s understand the concept of class and object. How it’s working together in OOPs.
A real-life example of class and object in Java
If you want to build a mobile phone then you plan for it. Firstly, you will decide it all features, advantage, operations, performances, etc. When you made a good plan then you want the production of mobiles. And obviously all the phones will share the same plan, but they can acquire different features.
Now, I am going to convert this idea into the JAVA class.
- First, we will create a class for mobile.
- The class has some variables and methods (it means features and operation of mobile).
- Now, we can create multiple objects of the same class (it means the production of mobile)
- Each object shares a common structure of a class, but they can contain different values.
public class Mobile { // Variables declared for mobile String phone_Name; String company = "Apple"; int size = 5; // Method of class Mobile public void printPhoneDetail() { System.out.println("Phone Name = "+ phone_Name); System.out.println("Company Name = "+ company); System.out.println("Size = "+ size +" inch"); } public static void main (String arg[]) { // Now creating first object of type class Mobile Mobile newMobile1 = new Mobile(); // access the variable by using first object newMobile1.phone_Name = "6s"; newMobile1.size = 6; // access the method by using first object newMobile1.printPhoneDetail(); // Now creating second object of type class Mobile Mobile newMobile2 = new Mobile(); // access the variable by using second object newMobile2.phone_Name = "7"; newMobile2.size = 7; // access the method by using second object newMobile2.printPhoneDetail(); } }
Output:
Phone Name = 6s
Company Name = Apple
Size = 6 inch
Phone Name = 7
Company Name = Apple
Size = 7 inch
Memory representation:
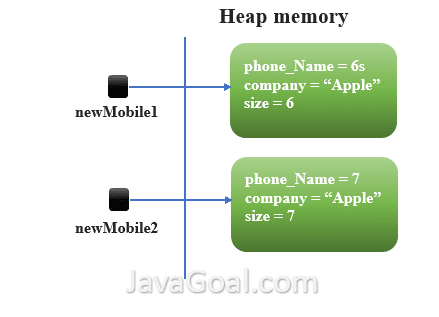
this web site is easy to understand each concept.This is good for every one
Thankyou padma thirunamn 🙂
You are doing a great job brother. Keep doing. Best Wishes 🙂
Every excellent website. Each topic is very easy to understand. One small suggestion, try to add more real-time examples for all topics so that it will be very easy to understand and no need to spend more time on each topic.
Thanks,
Megha
Thankyou
I have been finding it very difficult to understand the concept of Objects and Classes but this site is helping me a lot. Thanks for the simplicity. Please include more real life examples for us to keep practicing in every topic.
thanks you much