In java, inheritance is the most important topic. Inheritance is an important concept/feature of Object-Oriented. You must learn about inheritance and its type. The most common question asked in an interview “What is multiple inheritance in Java” and “Why multiple inheritance is not supported in Java”.
In this post, we will see how to achieve multiple inheritance using interface.
Here is the table content of the article will we will cover this topic.
1. What is multiple inheritance?
2. Why Java doesn’t support multiple inheritances?
3. How to achieve multiple inheritances by interface?
What is multiple inheritance?
As you know a class can extend the features of other classes by use of inheritance. When a class extending more than one class is known as multiple inheritance. But Java doesn’t allow it because it creates the diamond problem and too complex to manage. We can achieve the multiple inheritances by use of interface we will discuss it later. Firstly, we will concentrate on the current discussion.
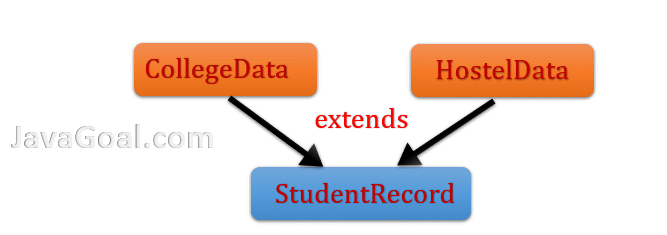
Here CollegeData and HostelData are two classes that are extended by the StudentRecord class. This is known as multiple inheritances. But in java, it is not possible to implement it. We will learn what is the reason behind and why java doesn’t support multiple inheritances.
Why Java doesn’t support multiple inheritances?
Let’s say we have two classes CollegeData and HostelData. Both classes have student records and having some methods. Here we have two methods in each class and one method is common in both classes.
StudentRecord is another class that extends both the classes(CollegeData and HostelData). It means the StudentRecord inherited all the methods of CollegeData and HostelData.

class CollegeData { public void collegeDetail() { System.out.println("College Name : DCSA"); System.out.println("College Grade : A"); System.out.println("University of College : KUK"); } public void studentData() { System.out.println("courses of Student : MCA, MTECH, MBA, BCA"); } } class HostelData { public void hostelDetail() { System.out.println("Hostel Name : RAMA"); System.out.println("Hostel location : KUK"); } public void studentData() { System.out.println("Student selected on based : Percentage, Financial condition"); } } public class StudentRecord extends CollegeData, HostelData { public static void main (String[] args) { StudentRecord obj = new StudentRecord(); obj.collegeDetail(); obj.studentData(); obj.hostelDetail(); obj.studentData(); } }
Output: Compilation error
There may be many reasons to show a compilation error. We will discuss the two major reasons.
First reason: You have seen CollegeData and HostelData class are extended by the StudentRecord class. Both classes have a common method studentData() that is inherited by StudentRecord. This is the route cause of the problem because when the programmer tries to call studentData() method in StudentRecord Class then the compiler will get confused about which method would be called? That’s the main reason why Java doesn’t support multiple inheritance.But we achieve the multiple inheritance using interface.
Second reason: As must be heard about the constructor chaining. A constructor can call the constructor of the superclass and the constructor defined in the same class. By default, JVM is dealing with the constructor chaining because if you are creating an object by a new keyword then JVM invokes the constructor of that class and that constructor invokes the superclass constructor.
When the programmer tries to create an object of StudentRecord then the compiler will get confused about whose constructor should be invoked?
Multiple inheritance using interface in java
We can achieve multiple inheritances by the use of interfaces. As you already know a class can implement any number of interfaces, but it can extend only one class. Before Java 8, Interfaces could have only abstract methods. It just defined the contract implementing by concrete classes.

Here we will discuss it by two examples.
Firstly, we will see if both interfaces don’t contain any common method. It will not create any ambiguity.
After that, if the interface has some common method then how to resolve the ambiguity.
Let’s discuss the first scenario when interfaces haven’t any common method. Here we will create two interfaces and one concrete class that implements them and provide the implementation to the abstract method.

Let’s say we have two interfaces CollegeData and HostelData. Both classes declared some method, but they haven’t any method. Here we have two methods in each class that inherited by concrete class.
StudentRecord is a concrete class that implements both interfaces. It means the StudentRecord inherited all the methods and provide the implementation to the method of CollegeData and HostelData interface.
interface CollegeData { public void collegeDetail(); public void studentData(); } interface HostelData { public void hostelDetail(); public void studentRecord(); } public class StudentRecord implements CollegeData, HostelData { @Override public void hostelDetail() { System.out.println("Hostel Name : RAMA"); System.out.println("Hostel location : KUK"); } @Override public void studentRecord() { System.out.println("Student selected on based : Percentage, Financial condition"); } @Override public void collegeDetail() { System.out.println("College Name : DCSA"); System.out.println("College Grade : A"); System.out.println("University of College : KUK"); } @Override public void studentData() { System.out.println("courses of Student : MCA, MTECH, MBA, BCA"); } public static void main (String[] args) { StudentRecord obj = new StudentRecord(); obj.collegeDetail(); obj.studentData(); obj.hostelDetail(); obj.studentData(); } }
Output: College Name : DCSA
College Grade : A
University of College : KUK
courses of Student : MCA, MTECH, MBA, BCA
Hostel Name : RAMA
Hostel location : KUK
courses of Student : MCA, MTECH, MBA, BCA
Let’s discuss the second scenario where we have a common method in both interfaces. When a concrete class implements both the interfaces and provides the implementation then how compiler take decision what method should be invoked?
To resolve this problem we will use default methods that was introduced in Java 8. You can read the default method in detail.
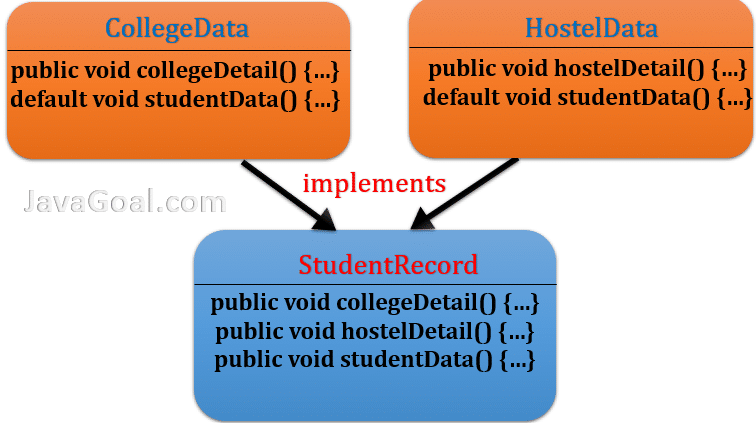
interface CollegeData { public void collegeDetail(); default void studentData() { System.out.println("courses of Student : MCA, MTECH, MBA, BCA"); } } interface HostelData { public void hostelDetail(); default void studentData() { System.out.println("Student selected on based : Percentage, Financial condition"); } } public class StudentRecord implements CollegeData, HostelData { @Override public void hostelDetail() { System.out.println("Hostel Name : RAMA"); System.out.println("Hostel location : KUK"); } @Override public void collegeDetail() { System.out.println("College Name : DCSA"); System.out.println("College Grade : A"); System.out.println("University of College : KUK"); } @Override public void studentData() { CollegeData.super.studentData(); HostelData.super.studentData(); } public static void main (String[] args) { StudentRecord obj = new StudentRecord(); obj.collegeDetail(); obj.hostelDetail(); obj.studentData(); } }
Output: College Name : DCSA
College Grade : A
University of College : KUK
Hostel Name : RAMA
Hostel location : KUK
courses of Student : MCA, MTECH, MBA, BCA
Student selected on based : Percentage, Financial condition
In the above example to resolve the diamond problem, we are using the super keyword and overriding the method again. You can read the diamond problem in detail.