Java 10 introduced a new feature called local variable type inference. In this post, we will discuss the Local variable type inference. This feature allows defining a variable using var and without specifying its type of it. A local variable can define by the use of the var keyword in java. In Java var is the most common way to declare local variables.
Everyone knows we must declare a data type with the variable name. From Java 10 onwards, we don’t need to declare data type with a local variable. We can simply use the var keyword. The compiler automatically detects the data type of the variable at compile time. Now the developer can skip the data type declaration only for local variables and the compiler will infer the data type.
Here is the table content of this article that we will cover.
- Why has the var keyword in java feature been introduced?
- How to declare local variables by use of var?
- var is not a keyword
- Where it can be used?
- Where it cannot be used?
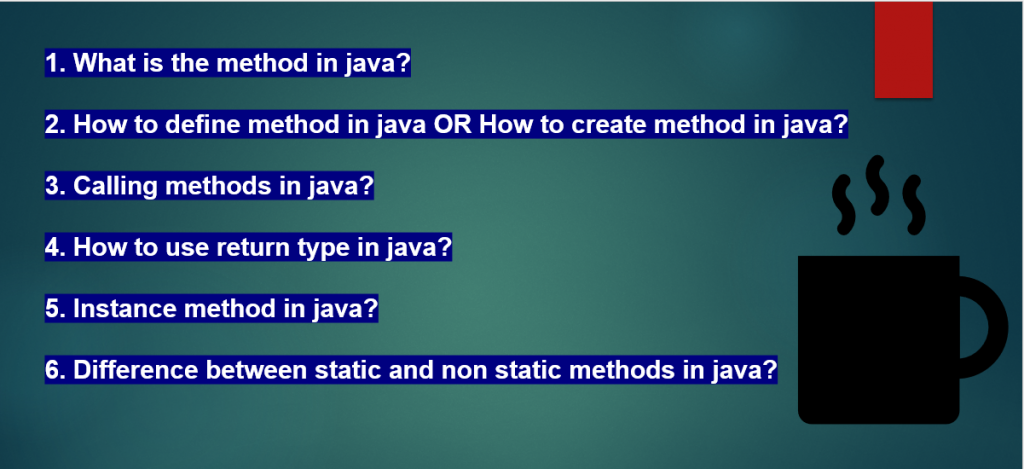
Why has the var keyword in java feature been introduced?
Before java 10, to declare a local variable we use the specific syntax:
Class_name variable_name = new Class_name(arguments);
Let’s see some examples and have a discussion on the same.
Example 1: ArrayList<String> listOfString = new ArrayList<String>(); Example 2: String hello = “Hello world!”; Example 3: String string = "This is a simple string for java example"; Example 4: Collector<String, ?, Map<String, Long>> byOccurrence = groupingBy(Function.identity(), counting()); Map<String, Long> wordFrequency = Arrays.stream(string.split(" ")).collect(byOccurrence);
The above examples are completely fine. But there are a few points that I want to discuss here:
1. In the first example, The type of variable clearly depends on the expression on the right-hand side, So the mentioning same thing before the name of the variable makes it redundant.
2. In the second example, we can clearly see the right-hand side is a string. Because it’s enclosed in double inverted commas. So variable declaration can be shorter.
3. The above two examples were simple so let’s discuss the 3rd example. Here we are using the Collector for groupBy and the output of one collector will be the input for the second one. So, we can simply use the var to reduce the complexity.
How to declare local variables by use of var?
You can simply right the var instead of mentioning the variable with datatype on the left side.
Syntax:
var variable_Name = new ClassName(arguments);
import java.util.Arrays; import java.util.HashMap; public class UseOfVar { public static void main(String[] args) { var intValue = 5; var stringValue = "JavaGoal.com"; var floatValue = 5.5f; var longValue = 5.5555; var listOfIds = Arrays.asList(1, 2, 3, 4, 5); var hashMap = new HashMap<Integer, String>(); System.out.println(intValue); System.out.println(stringValue); System.out.println(floatValue); System.out.println(longValue); System.out.println(listOfIds.toString()); System.out.println(hashMap); } }
Output: 5
JavaGoal.com
5.5
5.5555
[1, 2, 3, 4, 5]
{}
var is not a keyword
var is not a keyword but it’s a reserved type name. Also, keywords in Java cannot be used as identifiers. As we know a keyword has more restrictions than the reserved word. A keyword can’t be used for any type of identifier like variables, methods, classes and interfaces etc. But a reserved type name has fewer restrictions.
- We can use the var as a local variable
- Use of var as a method name
- Use of var as the package name
- Can’t use it as a class name
// Creating a package name with var package var; public class UseOfVar { public static void main(String[] args) { // Creating a local variable by use of var var var = new UseOfVar(); // Calling the method var.var(); } // Here we are using var as method name private void var() { System.out.println("Using var as method name"); } }
Output: Using var as method name
Where it can be used?
In java 10, developers skip the type declaration only for local variables (those defined inside method definitions, initialization blocks, for-loops, and other blocks like if-else). Let’s see different places where we can use it.
- Local variable initializers
- Use in loop
- In a static/instance initialization block
- Resource variables of the try-with-resources statement
Local variable initializers
In this example, we will create a different type of object by use of var. This example will explain the use of var
a Local variable. For each variable, we will use the data type and var, then see the result. Both ways should work fine.
import java.util.ArrayList; import java.util.Arrays; import java.util.HashMap; import java.util.List; public class UseOfVar { public static void main(String[] args) { int intValue = 5; String stringValue = "JavaGoal.com"; float floatValue = 5.5f; double doubleValue = 5.5555; List<Integer> listOfIds = Arrays.asList(1, 2, 3, 4, 5); HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); var intValue1 = 5; var stringValue1 = "JavaGoal.com"; var floatValue1 = 5.5f; var doubleValue1 = 5.5555; var listOfIds1 = Arrays.asList(1, 2, 3, 4, 5); var hashMap1 = new HashMap<Integer, String>(); System.out.println(intValue); System.out.println(stringValue); System.out.println(floatValue); System.out.println(doubleValue); System.out.println(listOfIds.toString()); System.out.println(hashMap); System.out.println(intValue1); System.out.println(stringValue1); System.out.println(floatValue1); System.out.println(doubleValue1); System.out.println(listOfIds1.toString()); System.out.println(hashMap1); } }
Output: 5
JavaGoal.com
5.5
5.5555
[1, 2, 3, 4, 5]
{}
5
JavaGoal.com
5.5
5.5555
[1, 2, 3, 4, 5]
{}
Use in loops
You can use the var in the loops to declare a local variable. Here, we will use var to declare the index value as well as a normal variable.
import java.util.ArrayList; import java.util.Arrays; import java.util.HashMap; import java.util.List; public class UseOfVar { public static void main(String[] args) { var list = Arrays.asList(1,2,5,6,8,4,7); for(var i = 0; i < list.size(); i++) { var a = 5; System.out.println("Value of i: "+ i + " and a: "+a); } for (var val : list) { System.out.println("Value from enhanced for loop: " + val); } var x = 0; while(x < list.size()) { var string = "Hello world!"; System.out.println("Value from while loop: " + list.get(x)); x++; } } }
Output: Value of i: 0 and a: 5
Value of i: 1 and a: 5
Value of i: 2 and a: 5
Value of i: 3 and a: 5
Value of i: 4 and a: 5
Value of i: 5 and a: 5
Value of i: 6 and a: 5
Value from enhanced for loop: 1
Value from enhanced for loop: 2
Value from enhanced for loop: 5
Value from enhanced for loop: 6
Value from enhanced for loop: 8
Value from enhanced for loop: 4
Value from enhanced for loop: 7
Value from while loop: 1
Value from while loop: 2
Value from while loop: 5
Value from while loop: 6
Value from while loop: 8
Value from while loop: 4
Value from while loop: 7
In a static/instance initialization block
We are already familiar with static blocks and instance blocks. So here we can declare a local variable by use of var.
public class UseOfVar { static { var x = "Hi there"; System.out.println(x); } public static void main(String[] args) { // main method } }
Output: Hi there
Resource variables of the try-with-resources statement
On the other hand, the var
type is a very nice fit for try-with-resource. For example:
import java.io.File; import java.io.FileNotFoundException; import java.io.PrintWriter; public class UseOfVar { public static void main(String[] args) { try (PrintWriter writer = new PrintWriter(new File("welcome.txt"))) { writer.println("Welcome message"); } catch (FileNotFoundException e) { throw new RuntimeException(e); } } }
Where it cannot be used
- For Global variable
- In Method parameters
- In Method return types
- Local variable declarations without any initialization
- Cannot use null for initialization
1. For Global variable: You can’t create a variable with var at class level (Global variable or class variable). It can be used only for local variables. If you try to create a global variable with var you will get a compile-time error.
class TryToUseVar { var a = 5; public static void main(String args[]) { System.out.println("Hello world!"); } }
Output: java: ‘var’ is not allowed here
2. In method parameter: You can’t use var in method parameters. It shows a compilation error if we use it in parameters.
class TryToUseVar { public static void main(String args[]) { System.out.println("Hello world!"); TryToUseVar.sample(1,5); } private static void sample(var a, var b) { System.out.println(a+b); } }
Output: java: ‘var’ is not allowed here
3. In Method return types: We can’t return the var from any type of method. Java doesn’t permit it. It shows a compilation error.
class TryToUseVar { public static void main(String args[]) { System.out.println("Hello world!"); TryToUseVar.sample(1,5); } private static var sample(int a, int b) { return (a+b); } }
Output: Compilation error
4. Local variable declarations without any initialization: As we know, we can leave the initialization of local variable. It means we can just declare then and later we can assign the value. But when we use var we have to initlize the local variable.
class TryToUseVar { public static void main(String args[]) { int i; // valid statement var a; // Invalid statement System.out.println("Hello world!"); } }
Output: Compilation error
5. Cannot use null for initialization: We can’t initialize a variable by null. It is not allowed. It shows a compilation error.
class TryToUseVar { public static void main(String args[]) { int i = 0; // valid statement var a = null; // Invalid statement System.out.println("Hello world!"); } }
Output: Compilation error
Thanks you much