In this post, we will discuss throw and throws keyword in java. In java throw and throws keywords are important keywords for interview questions.
Here is the table content of the article.
1. What is throw keyword in java?
2. What is throws keyword in java?
3. Difference between throw and throws in java?
throw keyword in java
The throw keyword in java uses to throw an exception from a method or block of code. If you want to throw an exception on the basis of certain conditions, you can use the throw keyword. For example, if a user entered the null/blank password we should throw an exception to the client. We can throw either checked or unchecked exceptions.
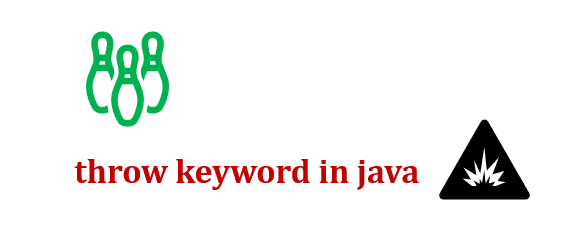
During the execution of the method if the compiler encounters a throw keyword then the execution of the current method stop and returned to the caller. The compiler tries to find the nearest try block. If thrown exception match with the catch block and is handle by a catch block otherwise it tries to find the next try block and so on. If no one catch block matches with the exception, then the default exception handler executes automatically.
method() { throw InstanceOfException; }
public class ExampleOfThrowException { static void validationOnNumbers(int number) { if(number <= 0) throw new ArithmeticException("This number is not allowed"); else System.out.println("Hello!!!!!"); } public static void main(String args[]){ validationOnNumbers(0); System.out.println("rest of the code..."); } }
Output: Exception in thread “main” java.lang.ArithmeticException: This number is not allowed at ExampleOfThrowException.validationOnNumbers(ExampleOfThrowException.java:4) at ExampleOfThrowException.main(ExampleOfThrowException.java:9)
The important thing about throw keyword:
- The instance must be of type Throwable or a subclass of Throwable.
- You can throw user(custom) exceptions because they extend Exception class and Exception extending the Throwable.
- You can throw one exception at a time.
The need to java throw exception by throw keyword: If you want to apply some set of rules in a program, then throw an exception explicitly using the throw keyword. For example, you can throw ArithmeticException if the user enters a number less than 0, or any other numbers.
throws keyword in java
throws keyword in java uses in a method declaration, denoted which Exception can possibly be thrown by this method. By using the throws keyword in java, we can throw multiple exceptions.
During the execution of the method if any exception occurs and the programmer already uses the throws keyword in java then the execution of the current method stops and returns to the caller. Then it depends upon the programmer either handling this exception by catching it or can rethrow it.
throws keyword mainly uses to handle the checked exceptions. If there occurs any unchecked exception, it is the programmer’s responsibility to check before performing any operation. The throws keyword always uses with a method signature.
// Exception name must be separated by comma type methodName(parameters) throws exceptionList { // body of method }
import java.io.IOException; public class ExampleOfThrowsException{ public static void main(String args[]){ try { checkedExceptionByThrows(0); } catch(Exception e) { System.out.println(e); } } public static void checkedExceptionByThrows(int number)throws IOException, ClassNotFoundException { if(number%2 == 0) throw new IOException("IOException Occurred"); else throw new ClassNotFoundException("ClassNotFoundException Occurred"); } }
Output: java.io.IOException: IOException Occurred
Important points to remember about throws keyword:
- throws keyword only uses for checked exceptions and the usage of the throws keyword for the unchecked exception is meaningless. If you are using the throws keyword to prevent this compile-time error we can handle the exception in two ways:
By using try-catch (As mentioned in the above example) By using the throws keyword (You can rethrow the exception)
- By using of throws keyword, you can’t prevent abnormal termination of the program. I help the compiler to provide convincing only.
Difference between throw and throws in java
Both have different purposes and both are used in different scenarios. Here we will find what is the difference between throw and throws in java.
1. throws keyword is used to declare an exception with the method name. It works like the try-catch block because the caller needs to handle the exception thrown by throws. On the other hand, the throw keyword is used to throw an exception explicitly.
2. throw is followed by an instance of Exception class and the throws keyword is followed by exception class names. It means the throw keyword always uses the object of the exception class whereas the throws keyword uses the exception class.
throw
throw new ArithmeticException("Arithmetic Exception");
throws
throws ArithmeticException;
3. throw keyword is used within the body of a method, On the other hand, the throws keyword is used in the method signature to declare the exceptions.
throw:
void usingThrowKeyword() { try { //throwing arithmetic exception using throw throw new ArithmeticException("You can’t divide a number by zero."); } catch (ArithmeticException e) { // handling exception } }
throws:
//arithmetic exception using throws void usingThrowsKeyword() throws ArithmeticException { //Statements }
4. By using the throw keyword you can declare only one exception at a time, but you can handle multiple exceptions by declaring them using the throws keyword. The throws keyword uses the comma(,) to separate the Exceptions.
throw:
void usingThrowKeyword() { //By using of throw keyword single exception is throwing throw new ArithmeticException("You can’t divide a number by zero."); }
throws:
//By using of throws declaring multiple exceptions void myMethod() throws ArithmeticException, NullPointerException { // handling exception }
I am going to go ahead and bookmark this post for my sis for a research project for class. This is a sweet web site by the way. Where did you obtain the template for this web page?
Enjoyed looking through this, very good stuff, thankyou .
I love the information on your website. I totally agree with your post here and I think that you are on the right track.