Here is the table content of the article will we will cover this topic.
1. What is Constructor overloading in java?
2. How many ways to overload Constructors?
3. Why does the Constructor overload?
4. When should we use Constructor overloading in java?
In this topic, we will learn multiple topics that will help you with interview questions. Here are a few topics we will cover:
What is constructor overloading in java? What are the constructor overloading rules? Constructor overloading program in java? Constructor overloading example in java? illustration of Constructor overloading in java? Write a program to implement Constructor overloading in java.
What is Constructor overloading in java?
As you know, Java allows method overloading. In the same manner, the programmer can do constructor overloading in java. A constructor can also be overloaded as a method. We can overload the constructor with different parameter lists. You can arrange the constructors in a way that each constructor performs a different task. The compiler differentiates by the number of parameters in the list and their types.
public class Student { String name; int rollNo; String className; boolean isFromCity; Student(String name, int rollNo) { this.name = name; this. rollNo = rollNo; } Student(String name, int rollNo, String className, boolean isFromCity) { this.name = name; this. rollNo = rollNo; this.className = className; this.isFromCity = isFromCity; } public void studentData() { System.out.println("Name of Student = " + name); System.out.println("Roll no = "+ rollNo); System.out.println("Class Name = "+ className); System.out.println("Is student belongs to city = "+ isFromCity); } public static void main (String arg[]) { // Creating object and providing some values by using parameterized constructor Student student1 = new Student("Ravi", 1); student1.studentData(); Student student2 = new Student("Ram", 2, "MCA", true); student2.studentData(); } }
Output:
Name of Student = Ravi
Roll no = 1
Class Name = null
Is student belongs to city = false
Name of Student = Ram
Roll no = 2
Class Name = MCA
Is student belongs to city = true
As you can see above example, how we are doing Constructor overloading in java. Here we have a class Student. We have created two Constructors that take different numbers of parameters.
How many ways to overload Constructors?
There are three ways to overload the constructor and let’s see the constructor overloading program in java.
1. You can overload by changing the number of arguments/parameters.
2. You can overload by changing the data type of arguments.
3. The order of the parameters of methods.
1. By changing the number of arguments
We can create multiple constructors of a class, but the number of arguments/parameters should be different.
Let’s create two constructors in class with different numbers of parameters/arguments
class Person { Person(String name) { System.out.println("Name of person = "+name); } Person(String name, String voterId) { System.out.println("Name of person = "+name ); System.out.println("Voter ID of " +name+ " = "+ voterId); } public static void main (String [] args) { // If user has not voter ID then just print name. Person person1 = new Person("Ravi"); // If user has voter ID then print name and voter Id Person person2 = new Person("Ram", "12345678"); } }
Output: Name of person = Ravi
Name of person = Ram
Voter ID of Ram = 12345678
A real-time example of constructor overloading in java
Let’s understand the overloading of the constructor with the example of the HashSet class. Let’s have a look at the HashSet class.
public HashSet(int initialCapacity) { map = new HashMap<>(initialCapacity); } public HashSet(int initialCapacity, float loadFactor) { map = new HashMap<>(initialCapacity, loadFactor); }
In HashSet, we have many constructors but we are going to discuss only two constructors.
These two constructors have different numbers of arguments. Here one constructor uses to set the capacity of HashSet that accepts only one argument, and another constructor accepts the two arguments to set the capacity and load factor of HashSet. These constructors are defined in the HashSet class to perform different tasks. So that the developer can use them according to the requirement. You can read these constructors HashSet(int capacity) and HashSet(int capacity, float loadFactor).
2. By changing the type of arguments
We can create multiple constructors with the same number of arguments, but the type of argument/parameters should be different.
class Person { Person(String name, String city) { System.out.println("Name of person = "+name); System.out.println("Name of City = "+city); } Person(String name, int voterId) { System.out.println("Name of person = "+name ); System.out.println("Voter ID of " +name+ " = "+ voterId); } public static void main (String [] args) { // If user has city and name then perform different action Person person1 = new Person("Ravi", "YNR"); // If user has voter ID then print name and voter Id Person person2 = new Person("Ram", 12345678); } }
Output: Name of person = Ravi
Name of City = YNR
Name of person = Ram
Voter ID of Ram = 12345678
Real time example to overload the constructors
Let’s understand the overloading of the constructor with the example of the ArrayList class. Let’s have a look at the ArrayList class. In ArrayList, we have many constructors but we are going to discuss two constructors. These two constructors have different types of arguments.
Here one constructor uses to set the capacity of ArrayList, and another uses it to accept a collection and insert an Arraylist. These constructs are defined in the ArrayList class to perform a different task. So that the developer can use them according to the requirement. You can read these constructors ArrayList(int capacity) and ArrayList(Collection c).
3. The Order of the parameters of the methods
You can overload the constructor by changing the order of the parameter. But it is only possible if both constructors have more than one parameter.
class Person { Person(String name, int PAN) { System.out.println("Name of person = "+name); System.out.println("PAN card details = "+PAN); } Person(int voterId, String name) { System.out.println("Name of person = "+name ); System.out.println("Voter ID of " +name+ " = "+ voterId); } public static void main (String [] args) { // If user has name and PAN card details then perform some action Person person1 = new Person("Ravi", 0001112); // If user has name and voter ID then perform some action Person person2 = new Person(12345678, "Ram"); } }
Output: Name of person = Ravi
PAN card details = 586
Name of person = Ram
Voter ID of Ram = 12345678
Why does the Constructor overload?
As we know constructor uses to initialize the object. Whenever we create an object by a new keyword, JVM invokes the constructor and initializes values in memory. Sometimes we want to initialize the objects in different ways and perform different actions.
We will discuss the example of Constructor overloading in ArrayList in Java. The ArrayList class has many constructors those uses to initial the object with different values and perform a different action.
Here ArrayList(int initialCapacity) is the constructor that takes only one argument that is used to set the initial capacity of ArrayList.
To provide more flexibility the ArrayList constructor is overloaded. Here ArrayList has another constructor ArrayList(Collection<? extends E> c) that takes a collection object and one takes initial Capacity. But Suppose we don’t know the size of ArrayList at the time of creation then we can simply use the default constructor ArrayList().
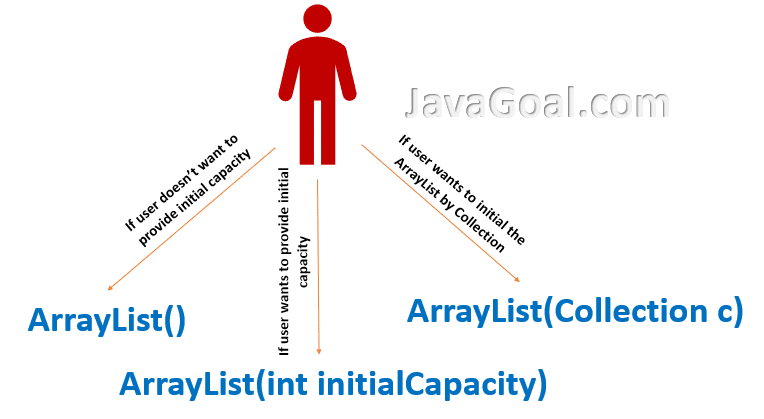
When should we use Constructor overloading in java?
Let’s say we have a record of Books. Books have some details like name, session, author Name, etc. So we can create a class having a Constructor that will take all the detail of a book.
class Book { String name; int bookId; String authorName; String session; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getBookId() { return bookId; } public void setBookId(int bookId) { this.bookId = bookId; } public String getAuthorName() { return authorName; } public void setAuthorName(String authorName) { this.authorName = authorName; } public String getSession() { return session; } public void setSession(String session) { this.session = session; } Book(String name, int bookId, String authorName, String session) { this.name = name; this.bookId = bookId; this.authorName = authorName; this.session = session; } } public class BookRecord { private void detailsOfBook(Book book) { System.out.println("Name of Book= "+ book.getName()); System.out.println("Id of Book= "+ book.getBookId()); System.out.println("Auther Name of Book= "+ book.getAuthorName()); System.out.println("Session of Book= "+ book.getSession()); } public static void main (String arg[]) { // Creating object and providing some values by using parameterized constructor Book bookOne = new Book("Digital & Logics", 101, "O.P", "2000"); Book bookTwo = new Book("Operating System", 181, "M.N", "2014"); BookRecord bookRecord = new BookRecord(); bookRecord.detailsOfBook(bookOne); bookRecord.detailsOfBook(bookTwo); } }
Output: Name of Book= Digital & Logics
Id of Book= 101
Auther Name of Book= O.P
Session of Book= 2000
Name of Book= Operating System
Id of Book= 181
Auther Name of Book= M.N
Session of Book= 2014
But, there are some books whose ID is not written on them. In that case, we can’t provide the book id to them. So we can’t use the constructor that is mentioned above because that needs all parameters and we haven’t ID of the book.
So what can we do?
We can create another constructor according to our needs. Where we will not use the ID of the book in the parameter list. Now we are introducing another Constructor in the Book class.
class Book { String name; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getBookId() { return bookId; } public void setBookId(int bookId) { this.bookId = bookId; } public String getAuthorName() { return authorName; } public void setAuthorName(String authorName) { this.authorName = authorName; } public String getSession() { return session; } public void setSession(String session) { this.session = session; } int bookId; String authorName; String session; Book(String name, int bookId, String authorName, String session) { this.name = name; this.bookId = bookId; this.authorName = authorName; this.session = session; } Book(String name, String authorName, String session) { this.name = name; this.authorName = authorName; this.session = session; } } public class BookRecord { private void detailsOfBook(Book book) { System.out.println("Name of Book= "+ book.getName()); System.out.println("Id of Book= "+ book.getBookId()); System.out.println("Auther Name of Book= "+ book.getAuthorName()); System.out.println("Session of Book= "+ book.getSession()); } public static void main (String arg[]) { // Creating object and providing some values by using parameterized constructor Book bookOne = new Book("Digital & Logics", 101, "O.P", "2000"); Book bookTwo = new Book("Operating System", 181, "M.N", "2014"); Book bookThree = new Book("Design System", "Q.U", "2018"); BookRecord bookRecord = new BookRecord(); bookRecord.detailsOfBook(bookOne); bookRecord.detailsOfBook(bookTwo); bookRecord.detailsOfBook(bookThree); } }
Output:Name of Book= Digital & Logics
Id of Book= 101
Auther Name of Book= O.P
Session of Book= 2000
Name of Book= Operating System
Id of Book= 181
Auther Name of Book= M.N
Session of Book= 2014
Name of Book= Design System
Id of Book= 0
Auther Name of Book= Q.U
Session of Book= 2018
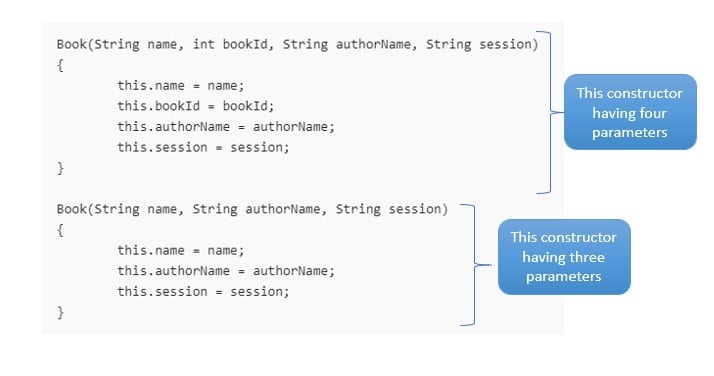
Amazing!
Hi
Can you give me some more real-time examples for this topic?
When should we use Constructor overloading in java?
https://javagoal.com/constructor-overloading-program-in-java/#4
When I will get the solutions of the question
thanks budddy.