We have seen, how to insert objects in HashMap and the internal working of HashMap. In this post, we will see How to get values from hashmap in java example, How does the java hashmap get() method work?
Here is the table content of the article will we will cover this topic.
1. get(Object key) method
2. getOrDefault(Object key, V defaultValue) method
3. values() method
get(Object key) method
This method is used to get or fetch the value mapped by the specified key. It returns the value if the specified key is mapped with value but if HashMap hasn’t mapped for the key then it returns NULL.
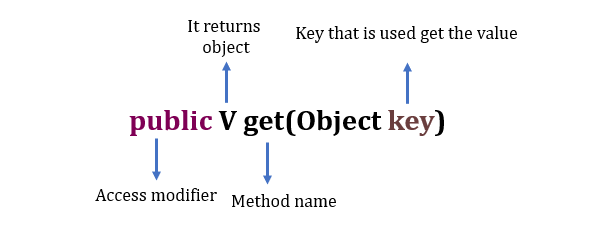
Where, Key is the key with which the specified value(V) is to be associated in HashMap.
return NULL, if there was no mapping for key otherwise it returns the value that associated with specified key.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); String value = hashMap.get(1); System.out.println("Value of key 1: "+value); value = hashMap.get(5); System.out.println("Value of key 5: "+value); } }
Output: Value of key 1: JavaGoal.com
Value of key 5: null
getOrDefault(Object key, V defaultValue) method
The getOrDefault(Object key, V defaultValue) method is used to get the value with the corresponding key or get the default value. This method checks the entry presented in HashMap with the given key, If the entry is not presented then it returns the default value.
It takes two parameters one is key and another is the default value.

Where, Key is the key with which the specified value(V) is to be associated in HashMap.
return value, if there is mapping for key otherwise it returns the default value.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); String value = hashMap.getOrDefault(1, "Hi"); System.out.println("Value of key 1: "+value); value = hashMap.getOrDefault(5, "Hi"); System.out.println("Value of key 5: "+value); } }
Output: Value of key 1: JavaGoal.com
Value of key 5: Hi
How to get all values from hashmap java
We can get all the values from HashMap by use of values() method. This method doesn’t take any parameter and returns a collection of values from HashMap. Let’s see How to get values from hashmap in java example
values() method
This method is used to get all the values from HashMap. It doesn’t take any parameter and returns the collection of values. If hashmap is empty then it returns the empty collection.

import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); // Getting all values from HahMap System.out.println("Get all the values: "+hashMap.values()); } }
Output: Get all the values: [JavaGoal.com, Learning, Website]
Your article has really peaked my interest. I am going