In this article, we will learn what is toString() method in Java. Most of the programmers may have good knowledge about the toString() method but we want to share some things about the toString() method in java.
Here is the table content of the article will we will cover this topic.
1. What is the toString() method?
2. Why OR When we should Override the toString() method?
3. How Wrappers, Collections, and StringBuffer using toString() method?
4. Best practices for Overriding toString() method?
What is the toString() method in java?
The toString() method is defined in Object class which is the super most class in Java. This method returns the string representation of the object. Let’s have a look at code.
public String toString() { return getClass().getName() + "@" + Integer.toHexString(hashCode()); }
As you can see in the above code its return type is String. It returns the class name of the object and appends the hashCode of that object.
As you already know every class in java is the child of Object class. It means each class inheriting the toString() method from Object class.
Whenever a user tries to print the object of any class then the java compiler internally invokes the toString() method.
public class Example { public static void main(String[] args) { Object obj = new Object(); System.out.println(obj); } }
Output: java.lang.Object@1e81f4dc
Why OR When we should Override the toString() method?
The default implementation of the toString() method is to return only the class name and hashcode of the object. But sometimes default implementation is not useful. In these types of situations, it is better to override the toString() method and return some informative data.
Let’s take an example of the default implementation of toString() method.
public class Student { int rollNo; String name; public static void main(String[] args) { Student s1 = new Student(); System.out.println("Object s1 = "+ s1); Student s2 = new Student(); System.out.println("Object s2 = "+ s2); } }
Output: Object s1 = Create.Student@1e81f4dc
Object s2 = Create.Student@5ccd43c2
In the above example, we are creating a class Student. In the Student class, we are creating and printing two objects s1 and s2. When the compiler tries to print the object s1 and s2, Then it invokes the toString() method. As toString() method is not defined in Student class then it will invoke the toString() method of Object class. The default implementation prints the class name and hashcode as you can see.
Let’s take the example of override the toString() method.
public class Student { int rollNo; String name; public static void main(String[] args) { Student s1 = new Student(); s1.name = "Ravi"; s1.rollNo = 1; System.out.println("Object s1 = "+ s1); Student s2 = new Student(); s2.name = "Ram"; s2.rollNo = 2; System.out.println("Object s2 = "+ s2); } @Override public String toString() { return this.rollNo + this.name; } }
Output: Object s1 = 1Ravi
Object s2 = 2Ram
How Wrappers, Collections, and StringBuffer using it?
All wrapper class, collections, and StringBuffer are already overridden the toString() method. When you create an object of Wrapper then you don’t need to override the toString() method.
public class Example { public static void main(String[] args) { Integer obj = new Integer(1); System.out.println(obj); } }
Output: 1
As you can see in the above example. We are creating an Object of Integer type and print that obj. It prints the value of Integer because the toString() method is already overridden in the Integer class.
Let’s have a look at the toString() method of the Integer class.
public String toString() { return toString(value); }
Best practices for Overriding toString() method
1. It would be better if you are using @Override annotation whenever overriding the method. It is used to avoid any errors because of typos.
Let’s say you want to override toString() method in your class. Then you can override the method with annotation or without annotation. Suppose you are overriding the method with annotation and you did a typing mistake. You wrote tostring() instead of toString(). Then compiler will show a compilation error.
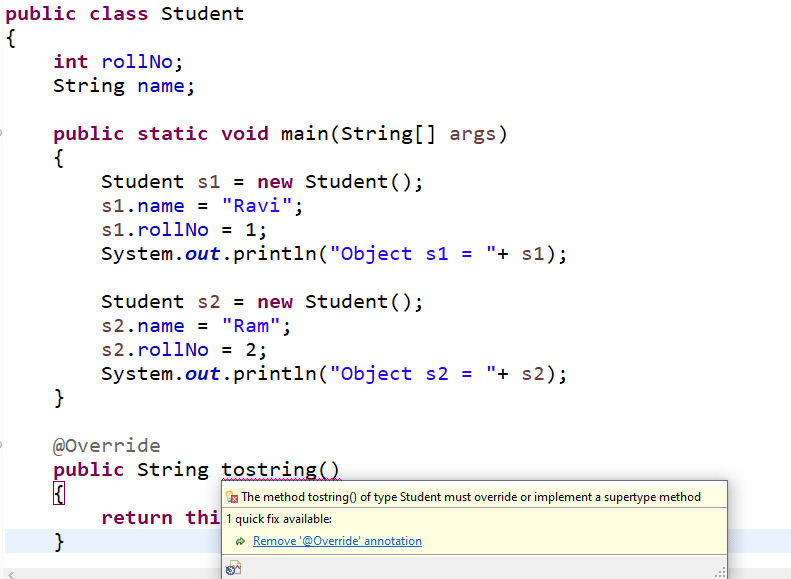
The method tostring() of type Student must override or implement a supertype method.
NOTE: If you are not using annotation, then the compiler will not show any error.
2. You should always try to return only useful data in toString() method. As you know our classes may have some sensitive information like phone numbers, passwords, etc. This information should not be public. If you are returning this type of data in the toString() method, it means you are creating security and data privacy issues.