Java 11 String New Methods
In this post, we will discuss the Java 11 String New Methods. Let’s discuss all the Java 11 String API Additions which help developers.
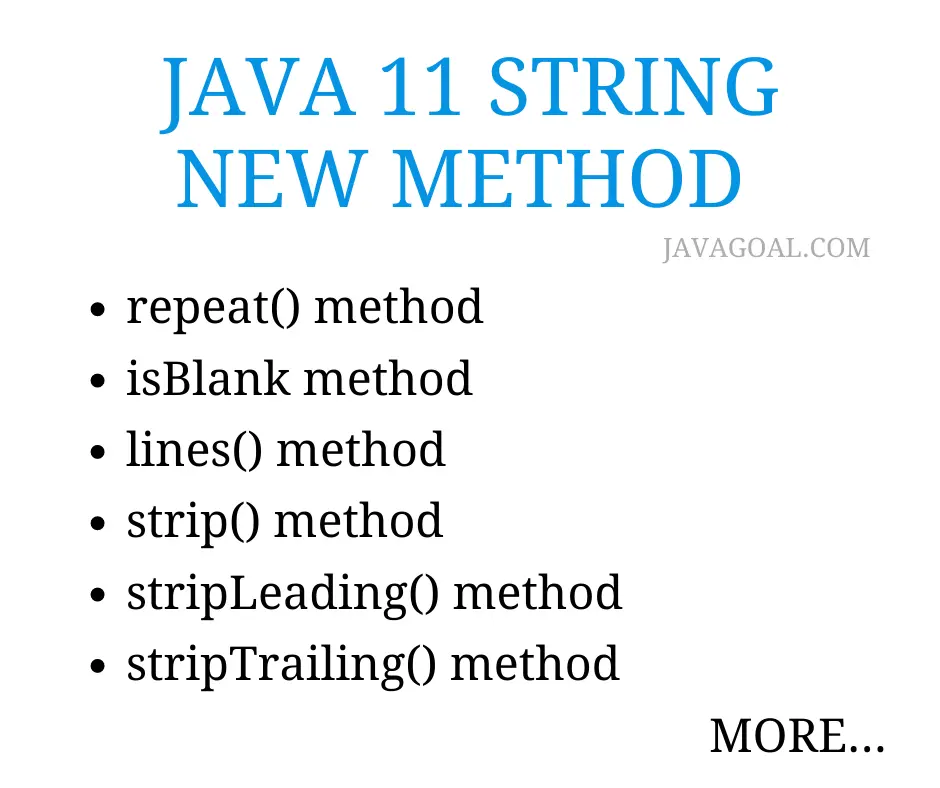
1. repeat() method
2. isBlank() method
3. lines() method
4. strip() method
5. stripLeading() method
6. stripTrailing() method
1. repeat(int count) method
In Java 11, the repeat(int count) method is defined in the String class. It returns a string whose value concatenates with the original string repeated count times. We will see it with an example.
This method takes a single argument of “int” type i.e. count which represents the number of times the string should be repeated. The count parameter must be non-negative, or an IllegalArgumentException will be thrown.
Syntax
public default String repeat(int count)
Let’s discuss the String repeat() method with an example and see how it works.
import java.util.Scanner; public class RepeatExample { public static void main(String[] args) { // Create a Scanner object to read user input Scanner scanner = new Scanner(System.in); // Prompt the user to enter a string System.out.print("Enter a string: "); String input = scanner.nextLine(); // Prompt the user to enter a repeat count System.out.print("Enter a repeat count: "); int count = scanner.nextInt(); // Call the repeat() method to repeat the input string String repeatedString = input.repeat(count); // Print the repeated string System.out.println("Repeated string: " + repeatedString); } }
Output: Enter a string: JavaGoal.com
Enter a repeat count: 3
Repeated string: JavaGoal.comJavaGoal.comJavaGoal.com
In the above program, the user enters a string and a repeat count. The repeat() method repeats the input string the specified number of times.
- Import the Scanner class from java.util package so that user we can read user input.
- Created a public class called RepeatExample.
- As usual, we created a public static main method, which is the entry point for the program.
- Created a Scanner object named scanner to read user input.
- Used System.out.print to prompt the user to enter a string.
- Used the nextLine() method of the Scanner object to read a line of text entered by the user and store it in the input variable.
- Then again used the System.out.print to prompt the user to enter a repeat count.
- Used the nextInt() method of the Scanner object to read an integer entered by the user and store it in the count variable.
- In the next line, we used the repeat() method on the input string, passing in the count variable to repeat the input string the specified number of times and store the result in the repeated string variable.
- Used System.out.println that repeated string to the console, along with a message indicating that it is the repeated string.
Why should we use the repeat() method with a practical example
Let’s say we want to create a program that needs to generate a string that consists of a repeated sequence of characters. For example, you need to generate a string that consists of alternating “A” and “B” characters, like this: ABABABABAB
Solution 1 without repeat() method
public class RepeatWithLoopExample { public static void main(String[] args) { int sequenceLength = 10; String sequence = ""; for (int i = 0; i < sequenceLength; i++) { if (i % 2 == 0) { sequence += "A"; } else { sequence += "B"; } } System.out.println(sequence); } }
Output: ABABABABAB
Solution 2 with repeat() method
public class RepeatWithExample { public static void main(String[] args) { int sequenceLength = 10; String sequence = "AB".repeat(sequenceLength / 2); System.out.println(sequence); } }
Output: ABABABABAB
2. isBlank() method
Java 11 introduces a new method, isBlank() method in the String class in Java 11. This method returns a boolean value that indicates whether a string is empty or contains only whitespace characters. This method doesn’t take any parameters.
Syntax
public boolean isBlank()
The isBlank() method uses to check whether the string is empty or contains only whitespace characters. If the string is blank, i.e., it is empty or contains only whitespace characters such as space, tab, newline, carriage return, then it returns true, etc. Otherwise, it returns false.
public class IsBlankExample { public static void main(String[] args) { // empty string String str1 = ""; // string containing only whitespace characters String str2 = " \t \n"; // non-blank string String str3 = "Hello"; boolean isStr1Blank = str1.isBlank(); // true boolean isStr2Blank = str2.isBlank(); // true boolean isStr3Blank = str3.isBlank(); // false System.out.println("First result: str1: "+ isStr1Blank); System.out.println("Second result: str2: "+ isStr2Blank); System.out.println("Third result: str3: "+ isStr3Blank)l } }
Output: First result: str1: true
Second result: str2: true
Third result: str3: false
Why should we use isBlank() method with a practical example
Data Validation: Whenever we process the user input, We should validate the data to ensure it is correct and complete. There are many cases, where we need to check if the user’s input contains only whitespace characters or is empty.
For example, Validation on the user’s password, name, etc so we can use the isBlank() method.
String password = request.getParameter("password"); if (password.isBlank()) { // Show error message: "Password is required." } else { // Process user input }
File Processing: When we process the text files, the compiler encounters blank lines or lines that contain only whitespace characters. In such cases, we can use the isBlank() method to filter out those lines.
List<String> urls = Files.lines(Paths.get("urls.txt")) .filter(line -> !line.isBlank()) .collect(Collectors.toList());
3. lines() method
Java 11 introduces a new method, The lines() method in the String class that returns a stream of lines extracted from a given string. It returns a sequential ordered stream, which means the lines will be returned in the order they occur in the string.
The lines() method works based on the newline character (‘\n’) and carriage return (‘\r’). It extracts the lines based on the newline character (‘\n’) or the combination of the carriage return (‘\r’) followed by the newline character (‘\n’). It automatically removes any leading or trailing white space from each line before returning them.
Syntax
public Stream<String> lines()
import java.util.stream.Stream; public class LinesMethodExample { public static void main(String[] args) { String str = "This is the first line.\nThis is the second line.\nThis is the third line."; Stream<String> lines = str.lines(); lines.forEach(System.out::println); } }
Why should we use lines() method with a practical example
This method helps to organize the text data. Suppose you want to parse text data that is organized into separate lines. So lines() method can split a large string into a stream of individual lines, which can then be processed or analyzed individually.
Let’s suppose we have a large text file having data about customer orders. A single line of text is representing the order and order details are separated by commas. We just want to analyze data to determine the total number of orders and the total amount of money spent on all orders.
import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.stream.Stream; public class UseOfLinesMethod { public static void main(String[] args) throws IOException { // Read the orders from the text file into a stream of lines Stream<String> orders = Files.lines(Paths.get("orders.txt")); // Compute the total number of orders and the total amount spent int numOrders = 0; double totalAmount = 0.0; for (String order : (Iterable<String>) orders::iterator) { String[] components = order.split(","); int quantity = Integer.parseInt(components[0]); double price = Double.parseDouble(components[1]); totalAmount += quantity * price; numOrders++; } // Print the results System.out.println("Total number of orders: " + numOrders); System.out.println("Total amount spent: $" + totalAmount); } }
4. strip() method
The strip() method is also introduced in java 11 in the String class. This method uses to remove the whitespace characters from both the beginning and end of a string. But It always returns a new string with the trimmed whitespace characters removed.
In whitespace characters, the spaces, tabs, and line breaks are included. The working of the strip() method is almost similar to the trim() method, but strip() method is more powerful as it can remove other Unicode white space characters in addition to the ASCII whitespace characters that trim() handles. We will discuss the difference between strip() and trim() methods later.
Syntax:
public String strip()
import java.util.Scanner; public class UserLogin { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter your username: "); String username = scanner.nextLine().strip(); System.out.print("Enter your password: "); String password = scanner.nextLine().strip(); if (username.equals("admin") && password.equals("password123")) { System.out.println("Login successful!"); } else { System.out.println("Invalid username or password!"); } scanner.close(); } }
Why should we use strip() method with a practical example
We can use the strip() method to remove leading (beginning) and trailing (ending) whitespace characters from a string. This type of method helps to prevent errors and improve data quality.
Suppose we are creating an application where we want to validate the email address to sign up for a newsletter. We want to validate the email address to make sure it’s in the correct format, and we also want to make sure that there are no leading or trailing spaces that could cause issues later on.
import java.util.Scanner; public class EmailSignup { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter your email address: "); String email = scanner.nextLine().strip(); // Validate the email address format if (!email.matches("[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}")) { System.out.println("Invalid email address format!"); } else { // Send email confirmation or add email to database System.out.println("Thanks for signing up for our newsletter!"); } scanner.close(); } }
Difference between strip() and trim() method
Both methods use to remove leading and trailing whitespace characters from a string in Java. But there are a few differences between them, Let’s discuss them one by one
Unicode support: The strip() method removes all Unicode whitespace characters, while the trim() method only removes ASCII whitespace characters, such as spaces, tabs, and line breaks.
public class DiffStripAndTrim { public static void main(String[] args) { String str = " \t Hello World! \n "; // Using trim() method String trimmed = str.trim(); System.out.println("Using trim() method:"); System.out.println("Original string: \"" + str + "\""); System.out.println("Trimmed string: \"" + trimmed + "\""); // Using strip() method String stripped = str.strip(); System.out.println("\nUsing strip() method:"); System.out.println("Original string: \"" + str + "\""); System.out.println("Stripped string: \"" + stripped + "\""); } }
Output:
Using trim() method:
Original string: ” Hello World!
“
Trimmed string: “Hello World!
”Using strip() method:
Original string: ” Hello World!
“
Stripped string: “Hello World!”
5. stripLeading() method
It uses to remove all leading white spaces from a string. The stripLeading() method was introduced with java 11 and it’s part of the String class. It returns a new string with all the leading white spaces removed.
public String stripLeading()
public class StripLeadingExample { public static void main(String[] args) { String str = " Hello, World!"; String strippedStr = str.stripLeading(); System.out.println("Original string: " + str); System.out.println("Stripped string: " + strippedStr); } }
6. stripTrailing() method
The stripTrailing() method was introduced in java 11 that uses to remove all trailing white spaces from a string. It’s a part of the String class and returns a new string with all the trailing white spaces removed.
public String stripTrailing()
public class StripTrailingExample { public static void main(String[] args) { String str = "Hello, World! "; String strippedStr = str.stripTrailing(); System.out.println("Original string: " + str); System.out.println("Stripped string: " + strippedStr); } }
Java 11 String New Methods