In this post, we see the diff between comparable and comparator and how comparable useful over Comparator? What are the different scenarios in which we should use the comparable and comparator in java.
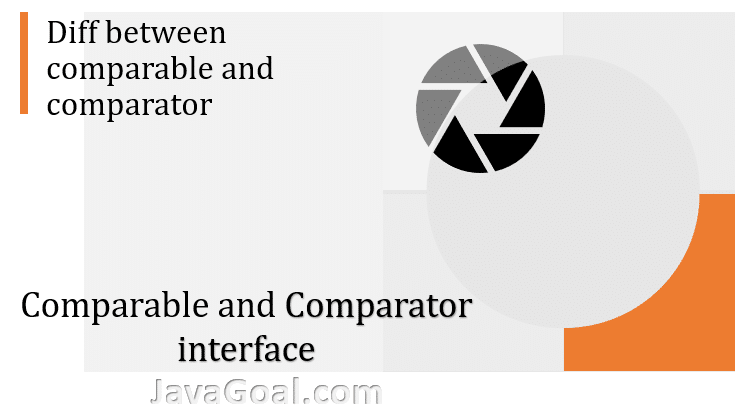
Diff between comparable and comparator
Usage of Java function Arays.sort() and Collection.sort(): If you are using Comparable for Sorting then you can use Arrays.sort() and Collections.sort() for sorting directly. You do not need to provide a comparator. You just need to call the method.
Provide ease for TreeMap and TreeSet: Here I want to suggest you please read TreeMap and TreeSet. Because TreeMap and TreeSet also use the Comparator and Comparable.
If your implementing Comparable interface in your class, then objects of that class can be used as keys in a TreeMap or as elements in a TreeSet. But if you are using Comparator interface then you have to write separate Comparator and pass it in the constructor of TreeMap or TreeSet. For more details click here (TreeSet with Comparator and TreeMap with Comparator).
Now we will see how can use comparable with TreeSet:
import java.util.TreeSet; class SortedString implements Comparable<String> { String string1; SortedString(String s) { this.string1 = s; } @Override public int compareTo(String string2) { return string1.compareTo(string2); } } public class ExampleOfTreeSet { public static void main(String arg[]) { TreeSet<String> listOfNames = new TreeSet<String>(); listOfNames.add("RAVI"); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("SITE"); for(String name : listOfNames) { System.out.println(name); } } }
Output: GOAL
JAVA
RAVI
SITE
Number of classes: As you know to implement the Comparator interface you have provides extra classes. There is some situation where you do not want to write extra class the you should go with Comparable interface. Because Comparable does not require creation of extra classes.
For more details of example please here for Comparable and Comparator .