1. abstract method with the final keyword
An abstract method can’t declare as final.
Reason: abstract and final both terms are opposite to each other. An abstract method says you must redefine it in a derived class. A final method says you can’t redefine this method in a derived class. Let’s see how use of abstract method in java.
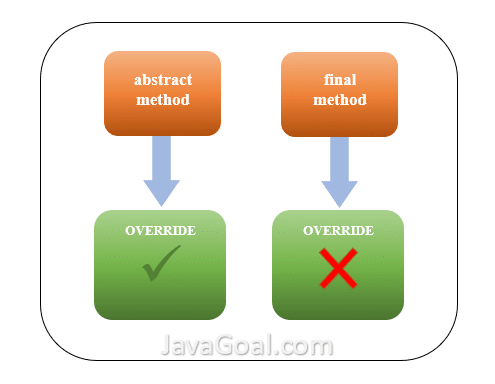
abstract class Data { abstract final void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
2. abstract method with the private keyword
An abstract method can’t be private because a private method is not accessible outside the class.
Reason: An abstract method says you must redefine it in a derived class. Users can redefine it if it is accessible from outside the class. A private method is not accessible from outside the class. So, we can’t use the private keyword with abstract methods.
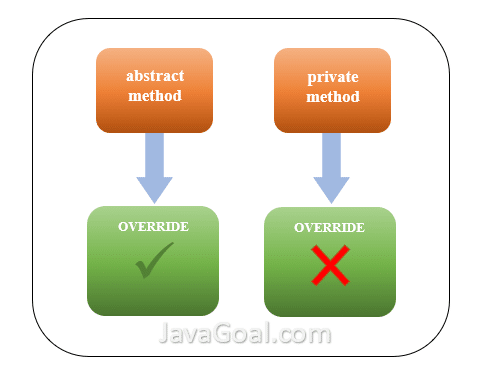
abstract class Data { abstract private void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
The abstract method showData() an in type Data can only set a visibility modifier, one of public or protected.
3. An abstract method with the static keyword
An abstract method can’t be static because we can’t override a static method.
Reason: If Java allows us to create an abstract static method(Method without body) and someone calls the abstract method using class name(because the static method can access directly by class name), then What would happen?
abstract class Data { abstract final void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
4. An abstract method with synchronized
An abstract method can’t be synchronized because a thread gets a lock on an object when it enters the method.
Reason: A thread that enters the synchronized method must get the lock of the object (or of the class) in which the method is defined. We can’t create an abstract class so there is no object with the lock.
abstract class Data { Abstract synchronized void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
5. An abstract method with strictfp
An abstract method can’t be strictfp.
Reason: Strictfp is a modifier and as you know we can change the modifier of an overridden method (method of parent class) while overriding the method in child class. It would make no sense to make an abstract method strictfp. That is, methods do not inherit modifiers.
NOTE: No modifiers are allowed on abstract methods except public and protected. You’ll get a compile-time error if you try.
Example:
abstract class Data { Abstract strictfp void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.