In this post, we will see how to print the Fibonacci series in java and the Fibonacci series using recursion in java. It is the most common program for interviews. Now this question has two parts. The interviewer can simply ask to write a Java program to print the Fibonacci series up to a given number. Even this program is very popular in viva, school or college classes. Before moving further, we have to understand what the Fibonacci series is and what is the logic behind it. So that we can simply convert that logic into java code.
Fibonacci series is a series of natural numbers where each next number is the sum of the previous two numbers. For example, 0, 1, 1, 2, 3, 5, 8, 11…. Here first two numbers of the Fibonacci series are always 0, 1. We start the logic from 3rd number and so on.
Let’s try to create a program. We will create a function to calculate Fibonacci numbers and then print those numbers on the Java console. In this example, we will use for loop.
Sometimes interviews can ask asks to write a Java program for Fibonacci numbers using recursion. So, here we will see both ways.
class FibonacciSeries { public static void main(String arg[]) { // Creating an object of FibonacciSeries class so that // we can call the printFibonacciSeries() method FibonacciSeries obj = new FibonacciSeries(); System.out.print("Printing Series:"); obj.printFibonacciSeries(8); } /** * This method print the FibonacciSeries by given maxNumber * @param maxNumber int value */ private void printFibonacciSeries(int maxNumber) { // Creating three variables that will contain the values int containerOne = 0, containerTwo = 1, sumOfContainer = 0; for(int number = 0; number < maxNumber; number++) { // For first two numbers we don't need any logic // we will them directly if (number == 0) { System.out.print(0 + " "); } else if(number == 1) System.out.print(1 + " "); else { //sumOfContainer(Fibonacci maxNumber) is sum of previous two Fibonacci maxNumber (containerOne + containerTwo) sumOfContainer = containerOne + containerTwo; containerOne = containerTwo; containerTwo = sumOfContainer; System.out.print(sumOfContainer +" "); } } } }
Output: Printing Series:0 1 1 2 3 5 8 13
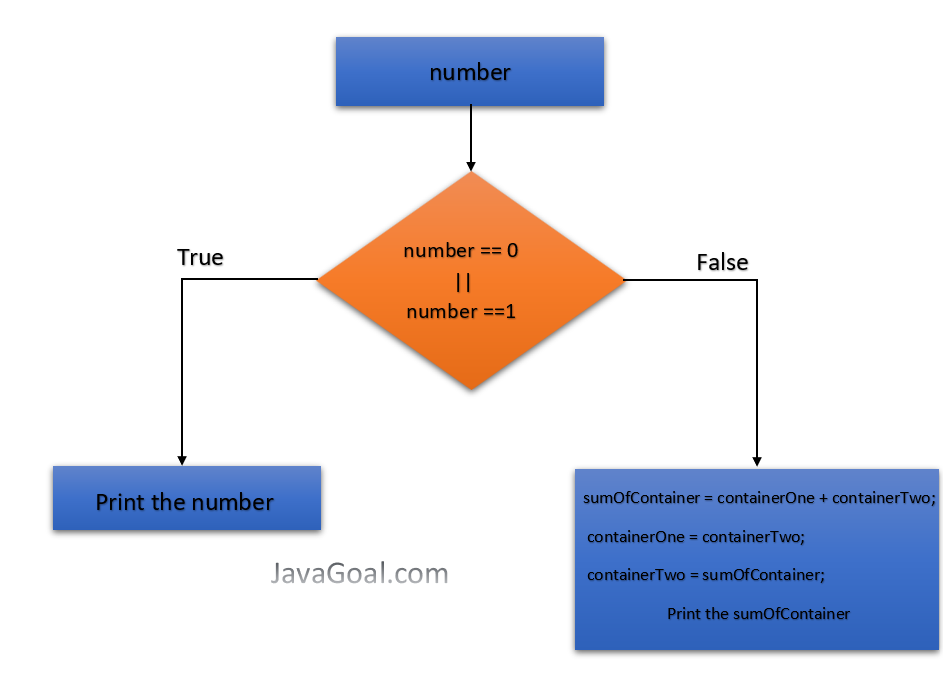
Firstly, we declared three variables with some specific values.
int containerOne = 0, containerTwo = 1, sumOfContainer = 0;

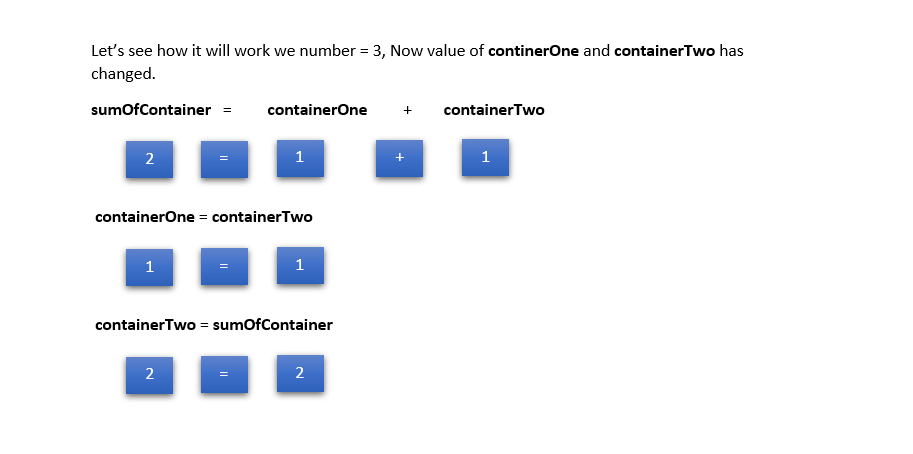
Fibonacci series by the recursive method
Let’s see another example by the recursive method.
You may be aware of the recursive method in java. A recursive method is one that has the capability to call itself. So in this example, we will see how to write a code in the recursive method so that it can call itself and print the Fibonacci series.
fibonacciRecursion():
Step 1: This series starts from 0 and 1. The recursion function takes an input number.
Step 2: When input number > 1, The function will call itself recursively.
//Using Recursion print the Fibonacci series public class FibonacciSeries { /** * This method prints the Fibonacci series * Here we will call this method by recursion * @param number, int type * @return an int type value */ public static int fibonacciRecursion(int number) { if(number == 0){ return 0; } if(number == 1){ return 1; } return fibonacciRecursion(number-2) + fibonacciRecursion(number-1); } public static void main(String args[]) { System.out.print("Print Series: "); for(int i = 0; i < 8; i++) { System.out.print(fibonacciRecursion(i) +" "); } } }
Output: Print Series: 0 1 1 2 3 5 8 13