The HashSet class in java has a lot of methods that are used to operate the data. Here we will discuss all the methods with examples. Let’s see all the HashSet Methods In Java.
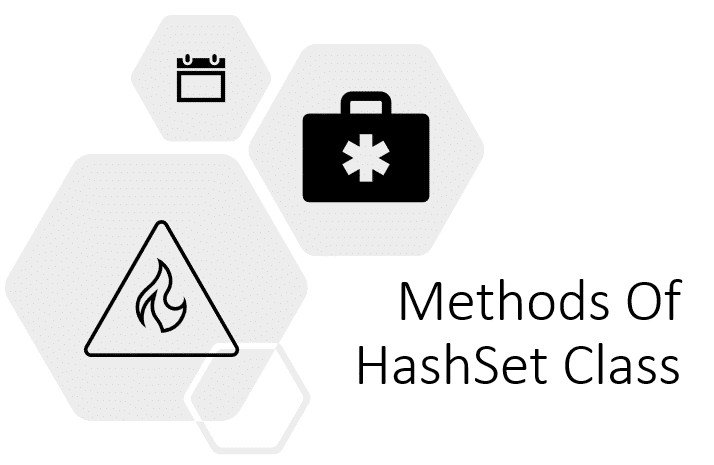
How to add elements to an HashSet?
1. add(E e) method: There is only one way to add the element in HashSet, the add(E e) method. This method returns a boolean value. You can read it in detail.
How to remove elements from HashSet?
2. remove(Object o) method: The remove(Object o) method is used to remove the given object from the HashSet. Its return type is boolean. You can read it in detail.
Some other methods
3. isEmpty(): The isEmpty() method returns a boolean value and check whether the HashSet is empty or not.It returns true if HashSet doesn’t contain any object otherwise false.
import java.util.HashSet; public class HashSetExample { public static void main(String[] args) { HashSet<String> setOfNames = new HashSet<String>(); setOfNames.add("Java"); setOfNames.add("Goal"); setOfNames.add("Website"); System.out.println("Is HashSet empty: "+ setOfNames.isEmpty()); } }
Output: Is HashSet empty: false
4. clear() method: The clear() method is used to remove all elements from the HashSet. It doesn’t return anything, because its return type is void.
import java.util.HashSet; public class HashSetExample { public static void main(String[] args) { HashSet<String> setOfNames = new HashSet<String>(); setOfNames.add("Java"); setOfNames.add("Goal"); setOfNames.add("Website"); System.out.println("Before clear() method, Is HashSet empty : "+ setOfNames.isEmpty()); setOfNames.clear(); System.out.println("After clear() method, Is HashSet empty: "+ setOfNames.isEmpty()); } }
Output: Before clear() method, Is HashSet empty : false
After clear() method, Is HashSet empty: true
5. contains(Object o): The contains(Object o) method is used to check whether the given object exist in the HashSet or not. Its return type is boolean.
import java.util.HashSet; public class HashSetExample { public static void main(String[] args) { HashSet<String> setOfNames = new HashSet<String>(); setOfNames.add("Java"); setOfNames.add("Goal"); setOfNames.add("Website"); System.out.println("Is object exists: "+setOfNames.contains("Java")); System.out.println("Is object exists: "+setOfNames.contains("Hello")); } }
Output: Is object exists: true
Is object exists: false
6. size(): When we want to check the size of Hashset then we can use size() method. The size() method returns the count of object present in HashSet.
import java.util.HashSet; public class HashSetExample { public static void main(String[] args) { HashSet<String> setOfNames = new HashSet<String>(); setOfNames.add("Java"); setOfNames.add("Goal"); setOfNames.add("Website"); System.out.println("Size of HashSet: "+setOfNames.size()); } }
Output: Size of HashSet: 3
iterator(): It is used to return an iterator over the elements in the HashSet. For more details