Object-Oriented Programming is totally based on four major pillars Inheritance, Abstraction, Encapsulation, and Polymorphism. A professional java developer must have knowledge of these pillars. In this post, we will give you complete knowledge of one of the most important concepts of OOPs i.e. Encapsulation in Java. How to achieve encapsulation in oop and how does work encapsulation in java with real time example.
Here is the table content of the article will we will cover this topic.
1. What is Encapsulation in Java?
2. How to achieve encapsulation?
3. Rules for encapsulation in Java?
4. Advantages of Encapsulation in Java?
5. Encapsulation in java example?
6. When to use encapsulation in java?
What is Encapsulation in Java?
Let’s define encapsulation in java or data encapsulation in oops:
Encapsulation is a process in which data and action bind together. By the use of encapsulation, data and function can wrap together in a single unit.
The encapsulation is like a shield that protects the data and prevents it from being accessed by the code outside the shield.
We can create a fully encapsulated class in Java by making all the data members of the class private. In encapsulation, the variables of a class are not accessible from outside the class. These variables can access only by the methods in which declared in the same class. It is also known as data hiding.

We can hide the variables of the classes and protect them by declaring all the variables private. The private variables are only accessible through the method, so we declared the method as public. If any class wants to interact with class variables, then there is only one way to interact which is a public method. We will explain it by diagram and after that discuss it in detail.
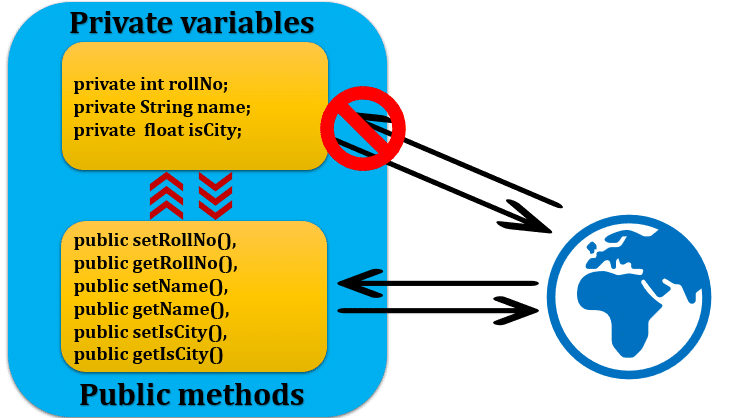
Encapsulation hides the internal representation from outside the class. You can protect the state of an object. Let’s say, we implemented encapsulation in a class then an attribute that is not visible from the outside of an object, and no one can change its state without the read and write access.
How to achieve encapsulation?
We can achieve encapsulation in java, we need to follow some rules and syntax. Whenever you are creating a class POJO(Plain old java object) class? Then you have to declare variables with the private keywords and methods with the public keyword.
class <class_name> { private variableName; // To achieve encapsulation variables should be private //Getter and Setter methods public returntype methodName(){ // Methods should be public } public void methodName(dataType variableName) { // Methods should be public } }
class Student { // For encapsulation all variables should be private private int rollNo; private String name; private String className; //You should provide setter ad getters for variables public int getRollNo (){ return rollNo; } public String getName(){ return name; } public String getClassName(){ return className; } public void setRollNo (int value){ rollNo = value; } public void setName (String value){ name = value; } public void setClassName (String value){ className = value; } } public class EncapsulationExample { public static void main(String args[]) { Student obj = new Student(); obj. setRollNo (1); obj. setName ("RAVI"); obj. setClassName ("MCA"); System.out.println("Student RollNo: " + obj.getRollNo ()); System.out.println("Student Name: " + obj.getName ()); System.out.println("Student Class: " + obj.getClassName ()); } }
Output: Student RollNo: 1
Student Name: RAVI
Student Class: MCA
Rules for encapsulation in Java
1. Class Variable should be private
2. To access the values of the variables, create public setter and getter methods.
Advantage of Encapsulation in Java
1. It gives better maintainability and flexibility and re-usability
Let’s discuss the above example. If you are changing in variable name or value, then other classes will not affect this change. They can still simply access the setter and getter. We can it at any point in time. This is introducing maintained property because we can change it without breaking classes.
2. read-only mode
If we don’t provide setter methods in the class, then the variable will be read-only. If we don’t want to change the value of any variable, then we will not provide its setter method.
Example :
- First, we need to declare it a private keyword.
- Don’t declare any setter method for that variable that you wouldn’t be changed.
- Since the set method is not present, so there is no way an outside class can modify the value of that field.
//This Java class which has only getter methods. It provides ready-only mode public class StudentKnowledge { //private variables private String knowledgeSource="JAVACONCEPTDOOR"; //getter method for knowledgeSource public String getKnowledgeSource() { return knowledgeSource; } } StudentKnowledge s = new StudentKnowledge(); s. setKnowledgeSource("ABC");//will render compile time error
Explanation: Now, you can’t change the value of the knowledge source data member that is “JAVACONCEPTDOOR”. You can’t change its value from outside the class as there is no setter method.
3. write-only mode
If we don’t provide getter methods in the class then the variable will be write-only(). If you don’t want to give access to the variable from outside the class? Then we will not provide its getter method.
Example :
- First, we need to declare it a private keyword.
- Don’t declare any getter method for that variable.
- Since the get method is not present, so there is no way an outside class can modify the value of that field.
Example of Read-only:
//This Java class which has only setter methods. It provides write-only public class StudentExample { //private variables private String knowledgeSource; //setter method for knowledge public void setKnowledgeSource (String knowledge) { this. knowledgeSource = knowledgeSource; } } System.out.println(s.getKnowledgeSource());//Compile Time Error, because there is no such method System.out.println(s.knowledgeSource);//Compile Time Error
Explanation: Now, you can’t get the value of the knowledge because the college data member is private. So, it can’t be accessed from outside the class. You can only change the value of the knowledge data members.
Encapsulation in java example
In java, by use of encapsulation in java, we can hide the inner implementation of the class. The inner implementation is only visible in its own class but its hides from outside the class. In this post, we will see how to use the Encapsulation in java example or encapsulation in oops with example or encapsulation example program in java.
Its software development technique is used in OOPs to hide internal object details. In other words, we can say it hides the data members of the class, that maintain the state of the object. Data hiding protects the class members to access from outside the class.

By using data hiding a program can be divided into objects with specific data and functions. We can create separate objects with unique data sets and functions, avoiding unnecessary penetration from other program classes.
Let’s discuss encapsulation in java with example, how data hiding protects the details of class members from outside. Suppose you have a class that contains several interdependent fields(variables). So, you should take care that must be in a consistent state. If any programmer is manipulating those fields directly, it may break the consistency of those fields. The programmer may change only one field without changing important related fields.
But instead of this, we can make a method that will communicate to interdependent fields. If the programmer wants to change the field, he just needs to call a method and the method will update all fields.
Let’s discuss again encapsulation in oops with example, suppose you have a project in which you are dealing with sensitive data. You don’t want to provide the read access of that and that should be right once after that programmer will not able to edit it. You must see the username or email address; it is chosen only one time after that you can’t change it.
In such type of scenario, we should make the private variable and provides the setter and getter accordingly.
class Account { // For encapsulation all variables should be private private String userName; private String password; private String name; private int age; private String country; public Account(String userName, String password, String name, int age, String country) { this.userName= userName; this.password = password; this.name = name; this.age = age; this.country = country; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getCountry() { return country; } public void setCountry(String country) { this.country = country; } } public class EncapsulationExample { public static void main(String args[]) { Account obj = new Account("javagoal", "myblog", "Ram", 27, "India"); // Here we can update variable those have setter System.out.println("Name : "+ obj.getName()); System.out.println("Age : "+ obj.getAge()); System.out.println("Country : "+ obj.getCountry()); } }
Output: Name : Ram
Age : 27
Country : India
When to use encapsulation in java
We have discussed the encapsulation in java and the encapsulation in java example. But what are the advantages of encapsulation? Why we should use java encapsulation?
We will explain all the advantages of encapsulation and also cover them in an encapsulation example.
1. Improve flexibility and maintainability
In Java, Encapsulation binds the data and action together. Using encapsulation, variable, and methods can wrap together in a single unit. To achieve encapsulation, all variables must be private, and they should be handled by the only method in the class. It improves the maintainability and flexibility and re-usability of code.
Let’s take an example of the student class. Here we are declaring all variables as private and creating the setter and getter to handle them.
class Employee { // For encapsulation all variables should be private private String name; private int empId; private String department; private int age; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getEmpId() { return empId; } public void setEmpId(int empId) { this.empId = empId; } public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } public class EncapsulationExample { public static void main(String args[]) { Employee emp1 = new Employee(); emp1.setName("Ram"); emp1.setEmpId(101); emp1.setAge(27); emp1.setDepartment("IT"); Employee emp2 = new Employee(); emp2.setName("Krishana"); emp2.setEmpId(105); emp2.setAge(25); emp2.setDepartment("Management"); System.out.println("Name : "+emp1.getName() + ", Age : "+emp1.getAge()); System.out.println("EmpId : "+emp1.getEmpId() + ", Department : "+emp1.getDepartment()); System.out.println("Name : "+emp2.getName() + ", Age : "+emp2.getAge()); System.out.println("EmpId : "+emp2.getEmpId() + ", Department : "+emp2.getDepartment()); } }
Output: Name : Ram, Age : 27
EmpId : 101, Department : IT
Name : Krishana, Age : 25
EmpId : 105, Department : Management
In the above example, all the variables and codes are implemented by the setter and getter. You can change the implementation of any setter or getter at any point in time. Because implementation is hidden for outside classes, they just use the setter and getter to access the private field. Suppose if make any change in the setEmpId() method(Setter) we just need to update the inner code of the method and it would not break the classes that use the code.
2. Provide more security
By use of encapsulation, you can provide more security to the class. You can make
the fields in read-only mode or write-only mode. Let’s take an example that will help you to understand the use of read-only or write-only mode.
Scenario 1 for reading mode
Suppose an example of the Employee records. Each employee has an id that must be created when the employee joins the company. The id remains the same till he does the job. If we create it in a java program, then id should be created only when we are creating an object of the employee
class Employee { // For encapsulation all variables should be private private String name; private int empId; private String department; private int age; // Creating a constrcutor that will set empId during creation of employee onbject Employee(int empId) { this.empId = empId; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getEmpId() { return empId; } public void setEmpId(int empId) { this.empId = empId; } public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } public class EncapsulationExample { public static void main(String args[]) { Employee emp1 = new Employee(101); emp1.setName("Ram"); emp1.setAge(27); emp1.setDepartment("IT"); Employee emp2 = new Employee(105); emp2.setName("Krishana"); emp2.setAge(25); emp2.setDepartment("Management"); System.out.println("Name : "+emp1.getName() + ", Age : "+emp1.getAge()); System.out.println("EmpId : "+emp1.getEmpId() + ", Department : "+emp1.getDepartment()); System.out.println("Name : "+emp2.getName() + ", Age : "+emp2.getAge()); System.out.println("EmpId : "+emp2.getEmpId() + ", Department : "+emp2.getDepartment()); } }
Output: Name : Ram, Age : 27
EmpId : 101, Department : IT
Name : Krishana, Age : 25
EmpId : 105, Department : Management
Here we are not providing the setter for empId field. The value of empId field is only initialized when we create an object of Employee. We will provide the getter of empId only. There is no other way to set the value of empId field because empId is private.
Scenario 2 for writing mode
Let’s say you do not want to provide read access to the age field. So, you can remove the getter of the age field. It will restrict the read access of the field because the field is private.
class Employee { // For encapsulation all variables should be private private String name; private int empId; private String department; private int age; // Creating a constrcutor that will set empId during creation of employee onbject Employee(int empId) { this.empId = empId; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getEmpId() { return empId; } public void setEmpId(int empId) { this.empId = empId; } public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } public void setAge(int age) { this.age = age; } } public class EncapsulationExample { public static void main(String args[]) { Employee emp1 = new Employee(101); emp1.setName("Ram"); emp1.setAge(27); emp1.setDepartment("IT"); Employee emp2 = new Employee(105); emp2.setName("Krishana"); emp2.setAge(25); emp2.setDepartment("Management"); System.out.println("Name : "+emp1.getName() + ", Age : "+emp1.getAge()); System.out.println("EmpId : "+emp1.getEmpId() + ", Department : "+emp1.getDepartment()); System.out.println("Name : "+emp2.getName() + ", Age : "+emp2.getAge()); System.out.println("EmpId : "+emp2.getEmpId() + ", Department : "+emp2.getDepartment()); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problems: The method getAge() is undefined for the type Employee The method getAge() is undefined for the type Employee at create.EncapsulationExample.main(EncapsulationExample.java:54)
It is throwing an exception because getAge() method is not defined in the class.
3. Protect class data
By using data hiding a program can be divided into objects with specific data and functions. We can create separate objects with unique data sets and functions, avoiding unnecessary penetration from other program classes.
Let’s take an example of how data hiding protects the details of class members from the outside. Suppose you have a class that contains several interdependent fields(variables). So, you should take care that must be in a consistent state. If any programmer is manipulating those fields directly, it may break the consistency of those fields. The programmer may change only one field without changing important related fields.
But instead of this, we can make a method that will communicate to interdependent fields. If the programmer wants to change the field, he just needs to call a method and the method will update all fields.
4. Data hiding
By use of encapsulation, we can achieve data hiding. It hides the implementation detail of the class from the outside user. The user only knows how to pass the value to the setter and how to get the value from the getter. There is nothing too visible to the user about how the class is storing values in the variables.
This site is really efficient way to gather leaf to root information