We already had a discussion on some keywords like the final keyword in java, static keyword in java, this keyword in java, etc. In this post, we will discuss the super keyword and the use of super keyword in java. In java super keyword is used to refer to the object of the immediate parent class. Let’s see how does super keyword in java with example and where we can use the super keyword.
Basically, the super keyword in java comes in a role with the concept of inheritance.
So, we will recommend you please read the inheritance first, which will provide ease to understand the super keyword.
As you know this keyword in java refers to the current object, in the same manner, the super keyword refers to the object of an immediate parent class. It means the super keyword uses in subclasses to access the object of the superclass. We can refer to the variable, constructor, and method of a superclass by using the super keyword. Suppose you have two classes, SubClass, and SuperClass. Both classes contain the variable or method with the same name. So, when we access the variable or method in the Subclass the compiler always gives preference to the SubClass variables. But there may be a situation when we want to access the variables or method of the Superclass. We use the super keyword to resolve these problems.
1. Java super keyword can access the variable of the parent class
2. Java super keyword can invoke the constructor of the parent class
3. Java super keyword can access the method of the parent class
1. Java super keyword can access the variable of the parent class
Suppose we are using inheritance in our classes. SubClass can have variables exactly the same as in SuperClass. So, when we will access the variable in the Subclass the compiler automatically accesses the variables of the SubClass. To access the variables of the Superclass we need to use the super keyword.
class ClassRecord { int rollNo = 5; String name = "Ram"; String className = "MCA"; public void showData() { System.out.println("RollNo : "+ rollNo); System.out.println("Name : "+ name); System.out.println("Class name : "+ className); } } class Record extends ClassRecord { int rollNo = 10; String name = "Krishan"; String className = "BCA"; public void printData() { System.out.println("RollNo : "+ rollNo); System.out.println("Name : "+ name); System.out.println("Class name : "+ className); } public static void main(String arg[]) { Record record = new Record(); record.printData(); } }
Output: RollNo : 10
Name : Krishan
Class name : BCA
As you can see in the above example ClassRecord is the super class and Record is the sub class. When we are accessing the variable in the Record class, so compiler always prints the variable declared in the same class. To access the variable of ClassRecord we will use the super keyword.
class ClassRecord { int rollNo = 5; String name = "Ram"; String className = "MCA"; public void showData() { System.out.println("RollNo : "+ rollNo); System.out.println("Name : "+ name); System.out.println("Class name : "+ className); } } class Record extends ClassRecord { int rollNo = 10; String name = "Krishan"; String className = "BCA"; public void printData() { System.out.println("RollNo : "+ super.rollNo); System.out.println("Name : "+ super.name); System.out.println("Class name : "+ super.className); } public static void main(String arg[]) { Record record = new Record(); record.printData(); } }
Output: RollNo : 5
Name : Ram
Class name : MCA
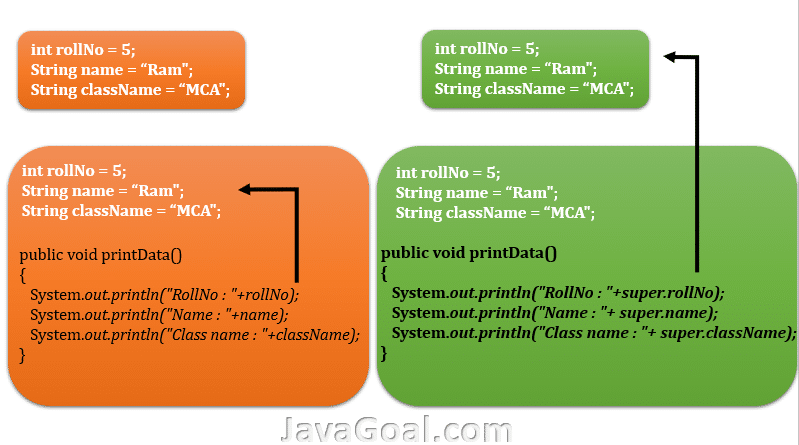
2. Java super keyword can invoke the constructor of the parent class
As we know, whenever we create an object of the class, the default constructor is automatically called by JVM. The JVM implicitly uses the super keyword from the constructor of the base class.
It is used to invoke the constructor of the parent class. To know how to use of super keyword in java please read the constructor chaining first. You can invoke the constructors of the parent class from the base class. To invoke the constructor of the parent class the super keyword must be the first statement in the constructor of the child class, otherwise, it will throw a compilation error.
class ClassRecord { int rollNo = 5; String name = "Ram"; String className = "MCA"; public ClassRecord(int rollNo, String name, String className) { System.out.println("Roll no : "+ rollNo); System.out.println("Name : "+ name); System.out.println("Class Name : "+ className); } } class Record extends ClassRecord { int numberOfRecord = 10; String schoolName = "Krishana Public school"; Record() { super(101, "Ram", "MCA"); System.out.println("Constructor of Child class"); System.out.println("Number of records : "+ numberOfRecord); System.out.println("School Name : "+ schoolName); } public static void main(String arg[]) { Record record = new Record(); } }
Output: Roll no : 101
Name : Ram
Class Name : MCA
Constructor of Child class
Number of records : 10
School Name : Krishana Public school
1. It invokes the constructor of the parent class. The JVM automatically uses the super keyword to invoke the constructor of the parent class. As you know every class inherits the Object class, so whenever we create an object of any class, the JVM automatically invokes the constructor of the Object class.
class ClassRecord { int rollNo = 5; String name = "Ram"; String className = "MCA"; ClassRecord() { System.out.println("Constructor of Parent class"); System.out.println("RollNo : "+ rollNo); System.out.println("Name : "+ name); System.out.println("Class name : "+ className); } } class Record extends ClassRecord { int numberOfRecord = 10; String schoolName = "Krishana Public school"; Record() { System.out.println("Constructor of Child class"); System.out.println("Number of records : "+ numberOfRecord); System.out.println("School Name : "+ schoolName); } public static void main(String arg[]) { Record record = new Record(); } }
Output: Constructor of Parent class
RollNo : 5
Name : Ram
Class name : MCA
Constructor of Child class
Number of records : 10
School Name : Krishana Public school
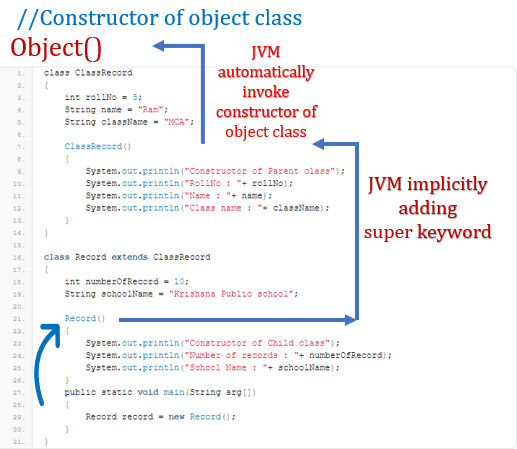
2. The super keyword is used to perform constructor chaining. We can call a default constructor of the parent class by use of the super() keyword (without arguments), to call a parameterized constructor of the parent class we can use the super(arguments) keyword(with arguments).
class BaseClass { // default constructor BaseClass() { System.out.println("This is default constructor of Base class"); } // parameterized construction have int type parameter BaseClass(String a) { System.out.println("This is parameterized constructor of base class. It is called form default constructor of derived class"); } } public class DerivedClass extends BaseClass { DerivedClass() { super("Hello"); System.out.println("This is default constructor of Drived class"); } public static void main (String arg[]) { DerivedClass n = new DerivedClass(); } }
Output:
This is parameterized constructor of base class. It is called form default constructor
This is default constructor of Derived class
3. To access the method of the parent class
In java super keyword is also used to invoke the method of the parent class. Actually, invoking the method of the parent class is useful in method overriding. Suppose a subClass has a method that is already defined in the superClass then it’s known as method overriding. In method overriding, the method of the child class hides the method of the parent class. To invoke the method of the parent class we can use the super keyword.
class ClassRecord { int rollNo = 5; String name = "Ram"; String className = "MCA"; public void showData() { System.out.println("RollNo : "+ rollNo); System.out.println("Name : "+ name); System.out.println("Class name : "+ className); } } class Record extends ClassRecord { int numberOfRecord = 10; String schoolName = "Krishana Public school"; public void showData() { System.out.println("Number of records : "+ numberOfRecord); System.out.println("School Name : "+ schoolName); } public static void main(String arg[]) { Record record = new Record(); record.showData(); } }
Output: Number of records : 10
School Name : Krishana Public school
As we have seen it’s printing the output from the Record class(child class). Because the child class overrides the parent class method. So let’s use the super keyword and invoke the method of StudentRecord class(Parent class).
class ClassRecord { int rollNo = 5; String name = "Ram"; String className = "MCA"; public void showData() { System.out.println("RollNo : "+ rollNo); System.out.println("Name : "+ name); System.out.println("Class name : "+ className); } } class Record extends ClassRecord { int numberOfRecord = 10; String schoolName = "Krishana Public school"; public void showData() { super.showData(); System.out.println("Number of records : "+ numberOfRecord); System.out.println("School Name : "+ schoolName); } public static void main(String arg[]) { Record record = new Record(); record.showData(); } }
Output: RollNo : 5
Name : Ram
Class name : MCA
Number of records : 10
School Name : Krishana Public school
This webisite is very usefull to learn the java with example. It too helpfull for me
Thankyou Keerthi 🙂
could u please post jdbc ,servelt,jsp concepts like this
Nice content. Cover all the topics. Add data structure and algorithm as well