The ArrayDeque in java is a class that is a part of the Java Collection framework which extends AbstractCollection class and implements the Deque, Cloneable, and Serializable interface.
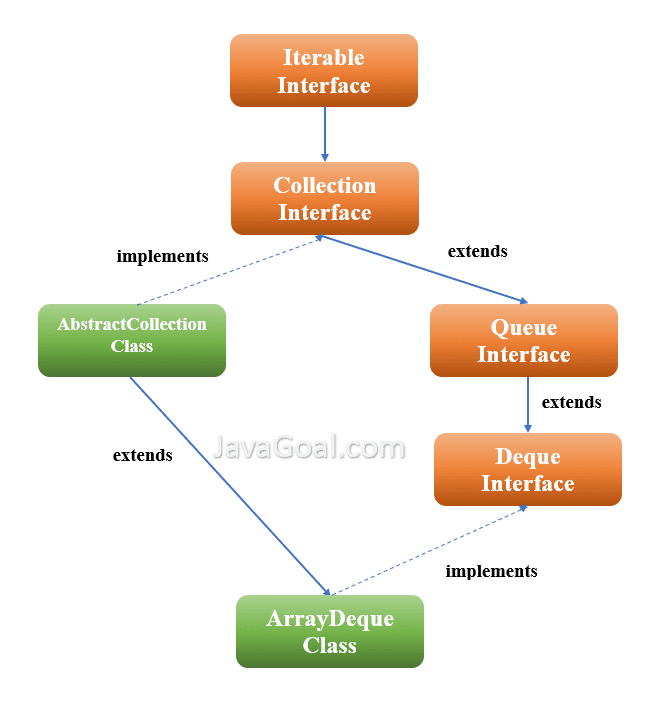
ArrayDeque class provides the implementation of the Deque interface and provides a resizable array. It is also known as Array Double Ended Queue or Array Deck
- Array deques grow automatically, there are no capacity restrictions.
- Array deques are not thread-safe. It means ArrayDeque does not support concurrent access by multiple threads.
- In ArrayDeque Null elements are not allowed.
- ArrayDeque class is faster than Stack and Deque.
Constructors of ArrayDeque in java
- ArrayDeque(): It is a default constructor of ArrayDeque class. It is used to create an empty ArrayDeque with initial default capacity 16.
- ArrayDeque(Collection<E> c): ): It is used to create an ArrayDeque using the elements of the specified collection c.
- ArrayDeque (int initialCapacity): It is used to create an empty ArrayDeque with a specified capacity.
Methods of ArrayDeque in java
- add(E e): This method is used to add the given element in Deque. It appends the specified element at the end of Deque. Its return type is boolean.
- addFirst(E e): This method is used to add the given element at the beginning of Deque. Its return type is void.
- addLast(E e): This method is used to add the given element at the end of Deque. Its return type is void.
- offer(E e): This method is used to add the given element at the end of Deque. Its return type is boolean.
- offerFirst(E e): This method is used to add the given element at the beginning of Deque. Its return type is boolean.
- offerLast(E e): This method is used to add the given element at the end of Deque. Its return type is boolean.
- push(): This method is used to add the given element at the beginning of Deque. Its return type is void.
- remove(): This method is used removes and returns the first element from Deque. Its return type is E.
- removeFirst(): This method is used to remove and return the first element from Deque. Its return type is E.
- removeLast(): This method is used removes and returns the last element from Deque. Its return type is E.
- removeFirstOccurrence(): This method is used removes the first occurrence of the specified element from Deque. Its return type is boolean.
- removeLastOccurrence(): This method is used removes the last occurrence of the specified element from Deque. Its return type is boolean.
- getFirst(): This method is used to get the first element from Deque. Its return type is E.
- getLast(): This method is used to get the last element from Deque. Its return type is E.
- peek(): This method is used to get to the first element from Deque. Its return type is E.
- peekFirst(): This method is also used to get the first element from Deque. Its return type is E.
- peekLast(): This method is used get to the last element from Deque. Its return type is E.
- poll(): This method is used to removes and return the first element from Deque. Its return type is E.
- pollFirst(): This method is also used to removes and return the first element from Deque. Its return type is E.
- pollLast(): This method is used to removes and returns the last element from Deque. Its return type is E.
- pop(): This method is also used to removes and return the first element from Deque. Its return type is E.
- element(): This method is used to get the first element from Deque. But doesn’t remove the element from the list. Its return type is E.
- isEmpty(): This method is used to whether Deque is empty or not. Its return type is boolean.
- clear(): This method is used to remove all elements from the Deque. It doesn’t return anything, so its return type is void.
- contains(Object o): It is used to check whether the given element present in ArrayList or not. Its return type is boolean.
- size(): It is used to check the number of elements present in Deque.
- clone(): It is used to return a shallow copy of this Deque instance.
- iterator(): It is used to return an iterator over the elements in this Deque.
- descendingIterator(): It is used to return an iterator over the elements in descending order.
- This method is used to return an array containing all of the elements of the ArrayList in correct order.
// Java program to demonstrate few functions of // ArrayDeque in Java import java.util.ArrayDeque; public class ExampleOfArrayDeque { public static void main(String[] args) { ArrayDeque<Integer> numbers = new ArrayDeque<Integer>(10); numbers.add(10); numbers.add(20); numbers.add(30); numbers.add(40); numbers.add(50); for (Integer element : numbers) { System.out.println("Element : " + element); } // remove() method : to get head System.out.println("Head element remove : " + numbers.remove()); System.out.println("The final array is: "+numbers); } }
Output: Element : 10
Element : 20
Element : 30
Element : 40
Element : 50
Head element remove : 10
The final array is: [20, 30, 40, 50]