As you already know the == operator always checks the reference or objects. So, whenever you create an object of Integer class(Wrapper class), the valueOf() method called every time.
The value of the method checks the integer value if the value lies between -128 to 127 it returns the same reference. If value exceed from the limit then it creates a new object.
You can check the code of the ValueOf() method in JDK.
public static Integer valueOf(int i) { if (i >= IntegerCache.low && i <= IntegerCache.high) return IntegerCache.cache[i + (-IntegerCache.low)]; return new Integer(i); }
Example:
public class Program { public static void main(String[] args) { Integer a = 100; Integer b = 100; System.out.println(a == b); a=1000; b=1000; System.out.println(a == b); } }
Output: true
false
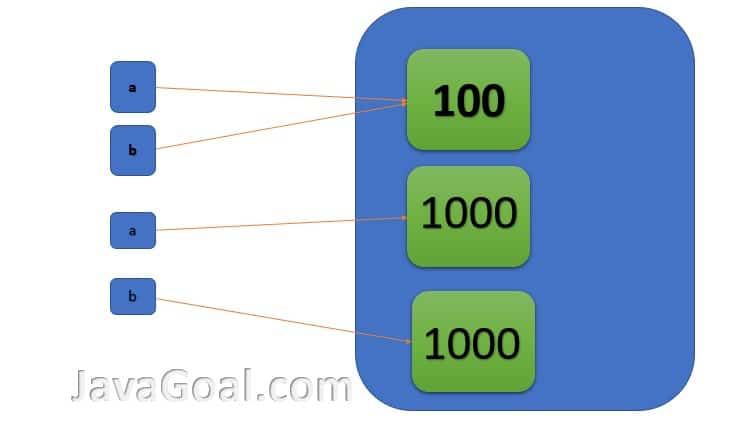
This answer is wrong answer should be true true,
as i have done it in c# it is giving true true
Hi nice to have your reply. Try it in Java please.
Why valueof creates a new object?
Hi Anirudh Sharma,
When we create an integer by direct assignment of an int literal. Then compiler converts it to an Integer reference.
For example:
Integer a = 100;
In this example, the compiler makes conversion from int literal to reference by use of the auto-boxing concept.
Integer a = Integer.valueOf(100);.
The Integer class(Wrapper) has an internal IntegerCache and the range of cache from -128 to 127(By default). So whenever we create int literal the Integer.valueOf() method returns objects of mentioned range from that cache. So a == b returns true because a and b both are pointing to the same object.
The valueOf() method checks the integer value. If the value of Integer is lies between -128 to 127 it returns the same reference from the pool. But if the value doesn’t lie in the limit it will create a new Object.
So if we later on increase the value beyond the cache allotted range or change it to a higher value later on in the program, will it again create a new object?
Yes, You can the cache size by VM argument It allowed us to set the high number. Which has given flexibility to tune the performance according to our application use case.