You can read about the java array in detail. Here we wrote an array with memory representation. But here we will do some exercise programs of java Array. Java Array Programs:
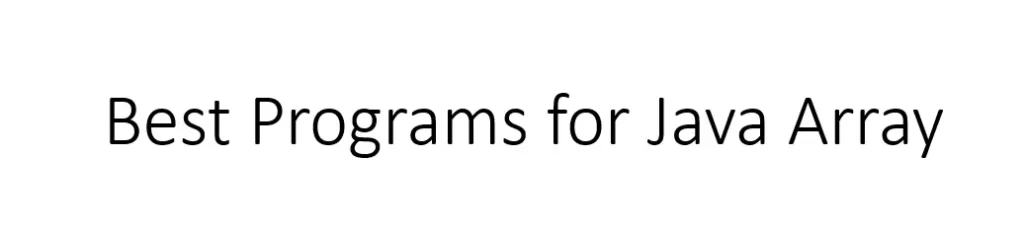
1. Write a Java program to sort a numeric array and a string array with comments on each line
import java.util.Arrays; import java.util.Collections; public class ArraySort { public static void main(String[] args) { // Numeric array - sort using Arrays class int[] numArray = {10, 5, 30, 20, 40}; System.out.println("Original Numeric Array: " + Arrays.toString(numArray)); Arrays.sort(numArray); System.out.println("Sorted Numeric Array (using Arrays.sort): " + Arrays.toString(numArray)); // Numeric array - sort using custom sorting int[] numArray2 = {10, 5, 30, 20, 40}; System.out.println("\nOriginal Numeric Array: " + Arrays.toString(numArray2)); for (int i = 0; i < numArray2.length - 1; i++) { for (int j = 0; j < numArray2.length - i - 1; j++) { if (numArray2[j] > numArray2[j + 1]) { int temp = numArray2[j]; numArray2[j] = numArray2[j + 1]; numArray2[j + 1] = temp; } } } System.out.println("Sorted Numeric Array (using custom sorting): " + Arrays.toString(numArray2)); // String array - sort using Arrays class String[] strArray = {"apple", "banana", "cherry", "date"}; System.out.println("\nOriginal String Array: " + Arrays.toString(strArray)); Arrays.sort(strArray); System.out.println("Sorted String Array (using Arrays.sort): " + Arrays.toString(strArray)); // String array - sort using Collections class String[] strArray2 = {"apple", "banana", "cherry", "date"}; System.out.println("\nOriginal String Array: " + Arrays.toString(strArray2)); Arrays.sort(strArray2, Collections.reverseOrder()); System.out.println("Sorted String Array (using Collections.sort in reverse order): " + Arrays.toString(strArray2)); } }
Output:
Original Numeric Array: [10, 5, 30, 20, 40]
Sorted Numeric Array (using Arrays.sort): [5, 10, 20, 30, 40]Original Numeric Array: [10, 5, 30, 20, 40]
Sorted Numeric Array (using custom sorting): [5, 10, 20, 30, 40]Original String Array: [apple, banana, cherry, date]
Sorted String Array (using Arrays.sort): [apple, banana, cherry, date]Original String Array: [apple, banana, cherry, date]
Sorted String Array (using Collections.sort in reverse order): [date, cherry, banana, apple]
2. Write a Java program to sum the values of an array in different ways with comments on each line
import java.util.stream.IntStream; public class ArraySum { public static void main(String[] args) { int[] numArray = {10, 20, 30, 40, 50}; // Calculate the sum of the array elements using for loop int sum = 0; for (int i = 0; i < numArray.length; i++) { sum += numArray[i]; } System.out.println("Sum of the array elements (using for loop): " + sum); // Calculate the sum of the array elements using IntStream int sum2 = IntStream.of(numArray).sum(); System.out.println("Sum of the array elements (using IntStream): " + sum2); // Calculate the sum of the array elements using stream and reduce int sum3 = IntStream.of(numArray).reduce(0, (a, b) -> a + b); System.out.println("Sum of the array elements (using stream and reduce): " + sum3); } }
Output:
Sum of the array elements (using for loop): 150
Sum of the array elements (using IntStream): 150
Sum of the array elements (using stream and reduce): 150
3. Write a Java program to print the following grid by multiple ways
Expected Output :
- - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - -
public class GridPrinter { public static void main(String[] args) { // Define the grid size int gridSize = 5; // Method 1: Using a nested for loop System.out.println("Method 1: Using a nested for loop"); for (int i = 0; i < gridSize; i++) { for (int j = 0; j < gridSize; j++) { System.out.print("X "); } System.out.println(); } // Method 2: Using a 2D array and a nested for loop System.out.println("\nMethod 2: Using a 2D array and a nested for loop"); char[][] grid = new char[gridSize][gridSize]; for (int i = 0; i < gridSize; i++) { for (int j = 0; j < gridSize; j++) { grid[i][j] = 'X'; } } for (int i = 0; i < gridSize; i++) { for (int j = 0; j < gridSize; j++) { System.out.print(grid[i][j] + " "); } System.out.println(); } // Method 3: Using a StringBuilder System.out.println("\nMethod 3: Using a StringBuilder"); StringBuilder sb = new StringBuilder(); for (int i = 0; i < gridSize; i++) { for (int j = 0; j < gridSize; j++) { sb.append("X "); } sb.append("\n"); } System.out.println(sb.toString()); } }
Output:
Method 1: Using a nested for loop
X X X X X
X X X X X
X X X X X
X X X X X
X X X X XMethod 2: Using a 2D array and a nested for loop
X X X X X
X X X X X
X X X X X
X X X X X
X X X X XMethod 3: Using a StringBuilder
X X X X X
X X X X X
X X X X X
X X X X X
X X X X X
4. Write a Java program to calculate the average value of array elements in multiple ways in the same program.
public class ArrayAverage { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; // Method 1: Using a for loop System.out.println("Method 1: Using a for loop"); int sum = 0; for (int i = 0; i < array.length; i++) { sum += array[i]; } double average = (double) sum / array.length; System.out.println("Average value: " + average); // Method 2: Using a foreach loop System.out.println("\nMethod 2: Using a foreach loop"); sum = 0; for (int value : array) { sum += value; } average = (double) sum / array.length; System.out.println("Average value: " + average); // Method 3: Using a stream System.out.println("\nMethod 3: Using a stream"); average = Arrays.stream(array).average().orElse(0.0); System.out.println("Average value: " + average); } }
Output:
Method 1: Using a for loop
Average value: 3.0Method 2: Using a foreach loop
Average value: 3.0Method 3: Using a stream
Average value: 3.0
5. Write a Java program to test if an array contains a specific value with multiple ways in the same program
import java.util.Arrays; public class ArrayContains { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; int target = 3; // Method 1: Using a for loop System.out.println("Method 1: Using a for loop"); boolean found = false; for (int value : array) { if (value == target) { found = true; break; } } System.out.println("Array contains " + target + ": " + found); // Method 2: Using Arrays.asList System.out.println("\nMethod 2: Using Arrays.asList"); found = Arrays.asList(array).contains(target); System.out.println("Array contains " + target + ": " + found); // Method 3: Using streams System.out.println("\nMethod 3: Using streams"); found = Arrays.stream(array).anyMatch(value -> value == target); System.out.println("Array contains " + target + ": " + found); } }
Output:
Method 1: Using a for loop
Array contains 3: trueMethod 2: Using Arrays.asList
Array contains 3: trueMethod 3: Using streams
Array contains 3: true
6. Write a Java program to find the index of an array element with multiple ways in the same program
import java.util.Arrays; public class ArrayIndex { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; int target = 3; // Method 1: Using a for loop System.out.println("Method 1: Using a for loop"); int index = -1; for (int i = 0; i < array.length; i++) { if (array[i] == target) { index = i; break; } } System.out.println("Index of " + target + ": " + index); // Method 2: Using Arrays.asList System.out.println("\nMethod 2: Using Arrays.asList"); index = Arrays.asList(array).indexOf(target); System.out.println("Index of " + target + ": " + index); // Method 3: Using streams System.out.println("\nMethod 3: Using streams"); index = Arrays.stream(array) .boxed() .indexOf(target); System.out.println("Index of " + target + ": " + index); } }
Output:
Method 1: Using a for loop
Index of 3: 2Method 2: Using Arrays.asList
Index of 3: 2Method 3: Using streams
Index of 3: 2
7. Write a Java program to remove a specific element from an array in multiple ways in the same program
import java.util.Arrays; import java.util.List; import java.util.stream.IntStream; public class RemoveElement { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; int target = 3; // Method 1: Using a for loop System.out.println("Method 1: Using a for loop"); int index = -1; for (int i = 0; i < array.length; i++) { if (array[i] == target) { index = i; break; } } if (index != -1) { int[] newArray = new int[array.length - 1]; System.arraycopy(array, 0, newArray, 0, index); System.arraycopy(array, index + 1, newArray, index, array.length - index - 1); array = newArray; } System.out.println("Array after removing " + target + ": " + Arrays.toString(array)); // Method 2: Using ArrayList System.out.println("\nMethod 2: Using ArrayList"); List<Integer> list = Arrays.stream(array) .boxed() .collect(java.util.stream.Collectors.toList()); list.remove((Integer) target); array = list.stream().mapToInt(Integer::intValue).toArray(); System.out.println("Array after removing " + target + ": " + Arrays.toString(array)); // Method 3: Using IntStream System.out.println("\nMethod 3: Using IntStream"); array = IntStream.of(array) .filter(value -> value != target) .toArray(); System.out.println("Array after removing " + target + ": " + Arrays.toString(array)); } }
Output:
Method 1: Using a for loop
Array after removing 3: [1, 2, 4, 5]Method 2: Using ArrayList
Array after removing 3: [1, 2, 4, 5]Method 3: Using streams
Array after removing 3: [1, 2, 4, 5]
8. Write a Java program to copy an array by iterating the array in multiple ways
import java.util.Arrays; public class ArrayCopy { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; // Method 1: Using a for loop System.out.println("Method 1: Using a for loop"); int[] copy1 = new int[array.length]; for (int i = 0; i < array.length; i++) { copy1[i] = array[i]; } System.out.println("Copied Array: " + Arrays.toString(copy1)); // Method 2: Using the clone() method System.out.println("\nMethod 2: Using the clone() method"); int[] copy2 = array.clone(); System.out.println("Copied Array: " + Arrays.toString(copy2)); // Method 3: Using Arrays.copyOf() method System.out.println("\nMethod 3: Using Arrays.copyOf() method"); int[] copy3 = Arrays.copyOf(array, array.length); System.out.println("Copied Array: " + Arrays.toString(copy3)); // Method 4: Using System.arraycopy() method System.out.println("\nMethod 4: Using System.arraycopy() method"); int[] copy4 = new int[array.length]; System.arraycopy(array, 0, copy4, 0, array.length); System.out.println("Copied Array: " + Arrays.toString(copy4)); } }
Output:
Method 1: Using a for loop
Copied Array: [1, 2, 3, 4, 5]Method 2: Using the clone() method
Copied Array: [1, 2, 3, 4, 5]Method 3: Using Arrays.copyOf() method
Copied Array: [1, 2, 3, 4, 5]Method 4: Using System.arraycopy() method
Copied Array: [1, 2, 3, 4, 5]
9. Write a Java program to insert an element (specific position) into an array in multiple ways
import java.util.Arrays; public class ArrayInsert { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; int position = 3; int value = 9; // Method 1: Using a for loop System.out.println("Method 1: Using a for loop"); int[] copy1 = new int[array.length + 1]; for (int i = 0; i < position; i++) { copy1[i] = array[i]; } copy1[position] = value; for (int i = position; i < array.length; i++) { copy1[i + 1] = array[i]; } System.out.println("Inserted Array: " + Arrays.toString(copy1)); // Method 2: Using Arrays.copyOfRange() method System.out.println("\nMethod 2: Using Arrays.copyOfRange() method"); int[] copy2 = new int[array.length + 1]; System.arraycopy(array, 0, copy2, 0, position); copy2[position] = value; System.arraycopy(array, position, copy2, position + 1, array.length - position); System.out.println("Inserted Array: " + Arrays.toString(copy2)); } }
Output:
Method 1: Using a for loop
Inserted Array: [1, 2, 3, 9, 4, 5]Method 2: Using Arrays.copyOfRange() method
Inserted Array: [1, 2, 3, 9, 4, 5]
10. Write a Java program to find the maximum and minimum value of an array in multiple ways
import java.util.Arrays; public class Main { public static void main(String[] args) { int[] numbers = {5, 2, 8, 9, 1, 4}; // Initialize min and max values with first element of the array int min = numbers[0]; int max = numbers[0]; // Iterate through the array and compare each element with the current min and max values for (int i = 1; i < numbers.length; i++) { if (numbers[i] < min) { min = numbers[i]; } if (numbers[i] > max) { max = numbers[i]; } } System.out.println("Min: " + min); System.out.println("Max: " + max); // Use Java's in-built Arrays class to find the min and max values int min1 = Arrays.stream(numbers).min().getAsInt(); int max1 = Arrays.stream(numbers).max().getAsInt(); System.out.println("Min: " + min1); System.out.println("Max: " + max1); } }
Output:
Min: 1
Max: 9
Min: 1
Max: 9
11. Write a Java program to reverse an array of integer values in multiple ways
import java.util.Arrays; class ReverseArray { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; System.out.println("Original array: " + Arrays.toString(array)); // Method 1: Using a loop System.out.println("\nMethod 1: Using a loop"); int[] reversedArray = reverseArrayUsingLoop(array); System.out.println("Reversed array: " + Arrays.toString(reversedArray)); // Method 2: Using a recursive function System.out.println("\nMethod 2: Using a recursive function"); int[] originalArray = {1, 2, 3, 4, 5}; reverseArrayRecursively(originalArray, 0, originalArray.length - 1); System.out.println("Reversed array: " + Arrays.toString(originalArray)); } private static int[] reverseArrayUsingLoop(int[] array) { int[] reversedArray = new int[array.length]; for (int i = 0, j = array.length - 1; i < array.length; i++, j--) { reversedArray[i] = array[j]; } return reversedArray; } private static void reverseArrayRecursively(int[] array, int startIndex, int endIndex) { if (startIndex >= endIndex) { return; } int temp = array[startIndex]; array[startIndex] = array[endIndex]; array[endIndex] = temp; reverseArrayRecursively(array, startIndex + 1, endIndex - 1); } }
Output:
Original array: [1, 2, 3, 4, 5]
Method 1: Using a loop
Reversed array: [5, 4, 3, 2, 1]Method 2: Using a recursive function
Reversed array: [5, 4, 3, 2, 1]