The sleep() method belongs to the Thread class. This sleep thread java method is used to sleep the currently executing thread for the specified amount of time. When the compiler executes the sleep() method it sleeps (Stop the executes) currently executing thread and control flow moved to another thread.
Let’s say we have three threads, thread1, thread2, and thread3. Now suppose all threads are in the RUNNABLE state it means they all are executing by CPU, but we want to execute stop the execution of thread1 only for some time period. Then we used the sleep() method. We can call it by class name because it is a static method.
className.sleep();
When JVM executes the above line, it moves the control flow from the thread1. But there is no grantee which thread will be the next for execution. It totally depends on the thread scheduler.
Real-life example: Let’s say we are downloading two files from the internet. The file1(Which is thread1) is in downloading state but file2(Which is thread2) is waiting for the server. So now I want to stop the downloading of file1 for some time period it means we can use the sleep thread java method.

After calling Thread.sleep(). The CPU can execute any thread it totally depends upon the thread scheduler.
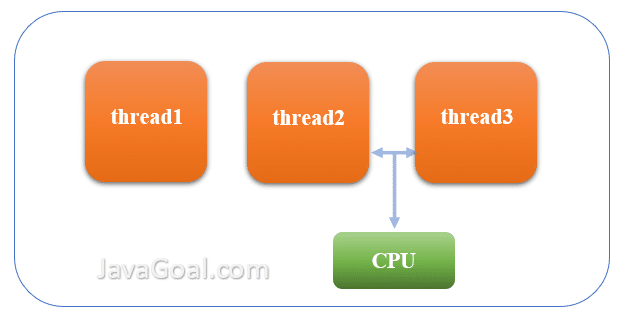
There are two overloaded sleep functions:
1. sleep(long milliseconds) method: This method doesn’t take any parameter. It will sleep the currently executing thread for the specified number of milliseconds. If a thread is interrupted, then it will throw InterruptedException. It doesn’t return anything. Its return type is void. It is a static method so you don’t need to call it by the object, it can call directly with a class name.
public static native void sleep()
className.join()
public class ExampleOfSleepMethod extends Thread { public void run() { for(int i = 1; i <= 5; i++) { System.out.println(Thread.currentThread().getName() + " is running and value of i = "+i); try { Thread.sleep(1000); // Sleep the current thread for 1 second } catch (InterruptedException e) { e.printStackTrace(); } } } public static void main(String args[]) { ExampleOfSleepMethod thread1 = new ExampleOfSleepMethod (); thread1.setName("Thread1"); ExampleOfSleepMethod thread2 = new ExampleOfSleepMethod (); thread2.setName("Thread2"); thread1.start(); thread2.start(); } }
Output:
Thread1 is running and value of i = 1
Thread2 is running and value of i = 1
Thread1 is running and value of i = 2
Thread2 is running and value of i = 2
Thread2 is running and value of i = 3
Thread1 is running and value of i = 3
Thread2 is running and value of i = 4
Thread1 is running and value of i = 4
Thread2 is running and value of i = 5
Thread1 is running and value of i = 5
In the above example, we created two threads thread1 and thread2. Both threads are used to print the counting. We called Thread.sleep() method for 1000 milliseconds in run() method. So, it sleeps the current running threads for one second and printing the output.
2. sleep(long milliseconds, int nanoseconds) method: This method takes two parameters of one is a type of long and another is int. It will sleep the currently executing thread for the specified number of times (milliseconds + nanosecond). If a thread is interrupted, then it will throw InterruptedException. It doesn’t return anything. Its return type is void. It is a static method so you don’t need to call it by the object, it can call directly with a class name.
public static void sleep(long milliseconds, int nanoseconds)
className.sleep(milliseconds, nanoseconds)
public class ExampleOfSleepMethod extends Thread { public void run() { for(int i = 1; i <= 5; i++) { System.out.println(Thread.currentThread().getName() + " is running and value of i = "+i); try { Thread.sleep(1000, 500); // Sleep the current thread for 1 second } catch (InterruptedException e) { e.printStackTrace(); } } } public static void main(String args[]) { ExampleOfSleepMethod thread1 = new ExampleOfSleepMethod (); thread1.setName("Thread1"); ExampleOfSleepMethod thread2 = new ExampleOfSleepMethod (); thread2.setName("Thread2"); thread1.start(); thread2.start(); } }
Output:
Thread1 is running and value of i = 1
Thread2 is running and value of i = 1
Thread1 is running and value of i = 2
Thread2 is running and value of i = 2
Thread2 is running and value of i = 3
Thread1 is running and value of i = 3
Thread2 is running and value of i = 4
Thread1 is running and value of i = 4
Thread2 is running and value of i = 5
Thread1 is running and value of i = 5