Top 5 Intellij idea shortcuts: JetBrains provides IntelliJ IDEA which is the most popular IDE (Integrated Development Environments) for software development. Whether you’re a seasoned developer or just starting, knowing your way around your tools can make a world of difference in productivity. To improve efficiency the keyboard shortcuts, play an important role. The primary advantage of shortcuts is to reduce the time spent on repetitive tasks. This post will show you the top 5 IntelliJ IDEA shortcuts that will elevate your coding skills to the next level.
You can learn Coding Best Practices And Clean Code
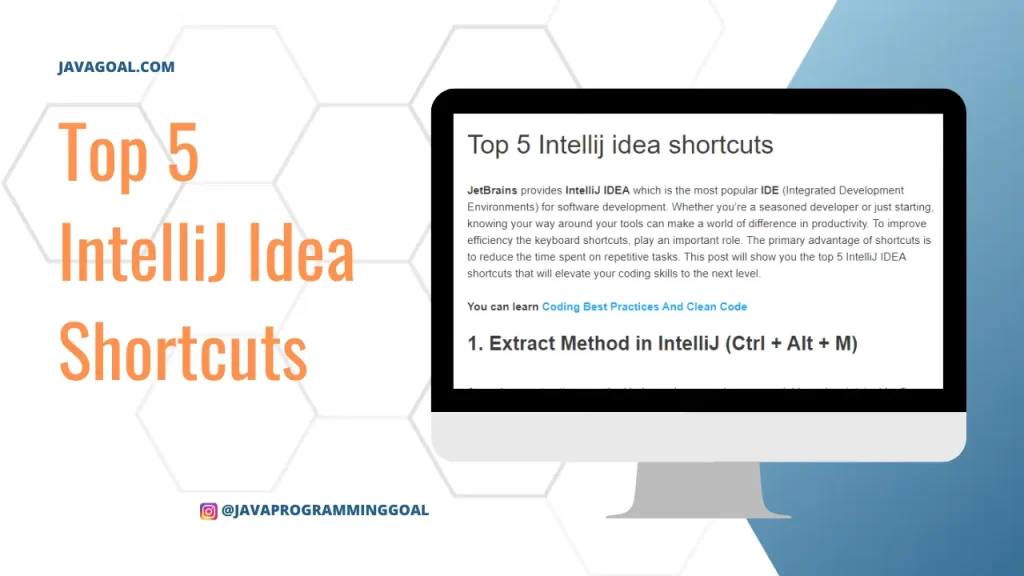
1. Extract Method in IntelliJ (Ctrl + Alt + M)
As we know extracting a method helps make our code more readable and maintainable. So you can use the Ctrl + Alt + M shortcut to quickly refactor a code block into its own method. Let me show you how,
Here you can see the code before Refactoring we have a simple Java class with a primary method. In this example, we have a method calculateArea() that calculates the area of a rectangle and prints it.
Before using Ctrl + Alt + M
public class RectangleArea { public static void main(String[] args) { calculateArea(); } public static void calculateArea() { int width = 5; int height = 10; int area = width * height; System.out.println("The area of the rectangle is: " + area); } }
But I want to keep the logic and print statement in a separate method. So that I can reuse it whenever I need it.
How to use Ctrl + Alt + M
- Select the lines responsible for calculating and printing the area, here we will select lines 9 and 10.
- Now Press a shortcut Ctrl + Alt + M.
- The new method will be automatically named “extracted,” and this name will be selected by default for renaming, allowing us to change it if desired.
After using Ctrl + Alt + M
public class RectangleArea { public static void main(String[] args) { calculateArea(); } public static void calculateArea() { int width = 5; int height = 10; calculateAndPrintArea(width, height); } private static void calculateAndPrintArea(int width, int height) { int area = width * height; System.out.println("The area of the rectangle is: " + area); } }
Now we have created a new method where the logic for calculating and printing the area of a rectangle. It makes the code easier to understand, test, and maintain. So, use the Ctrl + Alt + M shortcut in IntelliJ IDEA to extract methods and improve your code quality.
2. Inline Method in IntelliJ (Ctrl + Alt + N)
The Ctrl + Alt + N shortcut is used to create the inlining of a variable, method, or constant. It replaces the occurrences of a variable, method, or constant with its actual value or definition. We should perform this type of action only in a limited scope and doesn’t add much to readability or reusability.
Before using Ctrl + Alt + N
public class RectangleArea { public static void main(String[] args) { calculateArea(); } public static void calculateArea() { int width = 5; int height = 10; int area = width * height; printArea(area); } private static void printArea(int area) { System.out.println("The area of the rectangle is: " + area); } }
In this class, we have a main method where we calculate the area of a rectangle and store it in a variable. We then print the value of this variable. However, the printArea method is not necessary if its logic is straightforward and not reused elsewhere. So, we should simplify the code.
How to use Ctrl + Alt + M
- Place the cursor on the printArea method.
- Press Ctrl + Alt + N.
- It will show a dialog where you have to select one option
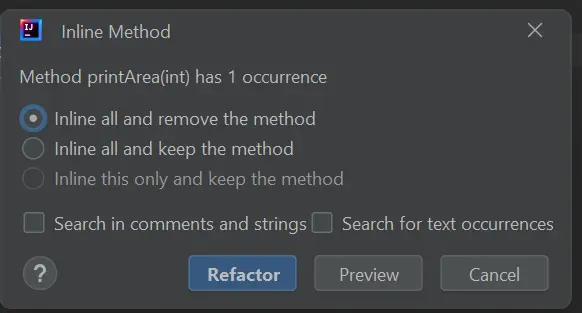
4. Confirm that you want to inline all and remove the method.
public class RectangleArea { public static void main(String[] args) { calculateArea(); } public static void calculateArea() { int width = 5; int height = 10; int area = width * height; System.out.println("The area of the rectangle is: " + area); } }
Now you can see, the printArea method has been inlined, and its logic has been directly incorporated into the main method.
3. Column Selection Mode in IntelliJ (Alt + Shift + Insert)
The “Column Selection Mode” is also known as “Block Selection Mode”. To enable this, we can use the Alt + Shift + Insert shortcut in IntelliJ IDEA. By using this feature, you can select text vertically rather than just horizontally. They will make it easier to edit multiple lines of code simultaneously. It can be extremely useful when you need to insert, delete, or modify the same text in multiple lines.
Before Using ALT+SHIFT+INSERT
Let’s take an example of a simple Java class with several attributes, and we want to add the private keyword in front of each attribute. In row selection mode (without Column Selection Mode), I have to modify each attribute one by one, and it will be time-consuming.
class Student { String name; int age; double GPA; String major; int studentID; }
How to Use ALT+SHIFT+INSERT
- Press Alt + Shift + Insert to toggle on the Column Selection Mode. You can see the Column Selection mode on the right bottom of the screen.

- Position the cursor at the beginning of the first line (String name;).
- Use the down arrow key to select the lines of text where you want to insert the private keyword.
- Once the text is selected vertically across the lines, start typing private, and it will be inserted into each line simultaneously.
class Student { private String name; private int age; private double GPA; private String major; }
4. Extracting a constant in IntelliJ (CTRL+ALT+C)
The Ctrl + Alt + C shortcut in IntelliJ IDEA is used for extracting a constant. This shortcut is very useful when you have a number or a string that’s hard-coded in multiple places, which could make your code less readable and maintainable.
Before Using CTRL+ALT+C
Suppose we have a class that calculates the area and perimeter of a circle and has a hard-coded value of Pi (3.14159) in the calculations. We want to replace it with a constant because having the value 3.14159 hard-coded in multiple places is not ideal. If you ever need to update this value or use it elsewhere, you will have to change it in every instance, which is error-prone. So how can I replace it constant?
public class Circle { public static void main(String[] args) { double radius = 5.0; double area = 3.14159 * radius * radius; double perimeter = 2 * 3.14159 * radius; System.out.println("Area: " + area); System.out.println("Perimeter: " + perimeter); } }
How to Use CTRL+ALT+C
- Place the cursor on one instance of the hard-coded value. In this example, it’s in line number 4 which is 3.14159.
- Press Ctrl + Alt + C.
- IntelliJ IDEA will prompt you to name the constant. You could name it something meaningful like PI_VALUE.
- Confirm the refactoring.
After Using CTRL+ALT+C
As you can see, the value 3.14159 is now stored in a constant named PI_VALUE, making the code more readable and easier to maintain. Now, if you ever need to change this value, you can do it in just one place, and the change will be reflected everywhere the constant is used.
public class RectangleArea { public static void main(String[] args) { int area = 5 * 10; System.out.println("The area of the rectangle is: " + area); System.out.println("Half of the area of the rectangle is: " + area / 2); } }
5. Extracting a variable in IntelliJ (CTRL+ALT+V)
The Ctrl + Alt + V shortcut in IntelliJ IDEA is used for extracting a variable. This refactoring technique is particularly useful when you have an expression that is used multiple times or is complex enough that breaking it down would make the code more readable and maintainable.
Before Using CTRL+ALT+V
Let’s consider a class where you calculate the area of a rectangle multiple times:
public class RectangleArea { public static void main(String[] args) { System.out.println("The area of the rectangle is: " + (5 * 10)); System.out.println("Half of the area of the rectangle is: " + (5 * 10) / 2); } }
In this example, you can see we are using the expression 5 * 10 is used to represent the area of the rectangle. This is repeated twice in the code. We should create a variable where we will store the calculation of this expression and then use the variable.
How to Use CTRL+ALT+V
1. Place your cursor on the expression 5 * 10 at line 3
2. Press Ctrl + Alt + V.
3. It will show a dialog and you can select for which statement you want to create a variable.

4. In our case I select the first expression and after that, It is showing me another dialog

5. You can replace only this occurrence or all
6. IntelliJ IDEA will prompt you to name the variable. You could name it something like an area
7. Confirm the refactoring.
After Using CTRL+ALT+V
After extracting the variable, your refactored Java class will look like:
public class RectangleArea { public static void main(String[] args) { int area = 5 * 10; System.out.println("The area of the rectangle is: " + area); System.out.println("Half of the area of the rectangle is: " + area / 2); } }
So these were the Top 5 Intellij idea shortcuts. I created some posts where I have posted about the most common Top 5 Intellij idea shortcuts.